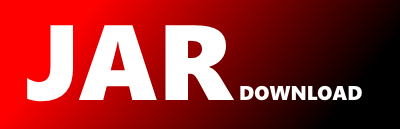
io.sphere.sdk.customers.CustomerImpl Maven / Gradle / Ivy
/*
This class has been generated by class io.sphere.sdk.annotations.processors.ResourceValueAnnotationProcessor
induced by the annotation io.sphere.sdk.annotations.ResourceValue.
in the source class io.sphere.sdk.customers.Customer.
*/
package io.sphere.sdk.customers;
import javax.annotation.Nullable;
import io.sphere.sdk.models.*;
import java.util.*;
import io.sphere.sdk.utils.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.annotation.JsonCreator;
final class CustomerImpl extends io.sphere.sdk.models.Base implements Customer {
private final java.lang.String id;
@Nullable()
private final java.lang.String customerNumber;
private final java.lang.String email;
@Nullable()
private final java.lang.String firstName;
@Nullable()
private final java.lang.String lastName;
private final java.lang.String password;
@Nullable()
private final java.lang.String middleName;
@Nullable()
private final java.lang.String title;
private final java.util.List addresses;
@Nullable()
private final java.lang.String defaultShippingAddressId;
@Nullable()
private final java.lang.String defaultBillingAddressId;
@JsonProperty("isEmailVerified")
private final java.lang.Boolean emailVerified;
@Nullable()
private final java.lang.String externalId;
@Nullable()
private final io.sphere.sdk.models.Reference customerGroup;
@Nullable()
private final java.lang.String companyName;
@Nullable()
private final java.lang.String vatId;
@Nullable()
private final java.time.LocalDate dateOfBirth;
@Nullable()
private final io.sphere.sdk.types.CustomFields custom;
@Nullable()
private final java.util.Locale locale;
private final java.util.List shippingAddressIds;
private final java.util.List billingAddressIds;
private final java.lang.Long version;
private final java.time.ZonedDateTime createdAt;
private final java.time.ZonedDateTime lastModifiedAt;
@JsonCreator()
CustomerImpl(final java.util.List addresses, final java.util.List billingAddressIds, final java.lang.String companyName, final java.time.ZonedDateTime createdAt, final io.sphere.sdk.types.CustomFields custom, final io.sphere.sdk.models.Reference customerGroup, final java.lang.String customerNumber, final java.time.LocalDate dateOfBirth, final java.lang.String defaultBillingAddressId, final java.lang.String defaultShippingAddressId, final java.lang.String email, @com.fasterxml.jackson.annotation.JsonProperty("isEmailVerified") final java.lang.Boolean emailVerified, final java.lang.String externalId, final java.lang.String firstName, final java.lang.String id, final java.time.ZonedDateTime lastModifiedAt, final java.lang.String lastName, final java.util.Locale locale, final java.lang.String middleName, final java.lang.String password, final java.util.List shippingAddressIds, final java.lang.String title, final java.lang.String vatId, final java.lang.Long version) {
this.addresses = addresses;
this.billingAddressIds = billingAddressIds;
this.companyName = companyName;
this.createdAt = createdAt;
this.custom = custom;
this.customerGroup = customerGroup;
this.customerNumber = customerNumber;
this.dateOfBirth = dateOfBirth;
this.defaultBillingAddressId = defaultBillingAddressId;
this.defaultShippingAddressId = defaultShippingAddressId;
this.email = email;
this.emailVerified = emailVerified;
this.externalId = externalId;
this.firstName = firstName;
this.id = id;
this.lastModifiedAt = lastModifiedAt;
this.lastName = lastName;
this.locale = locale;
this.middleName = middleName;
this.password = password;
this.shippingAddressIds = shippingAddressIds;
this.title = title;
this.vatId = vatId;
this.version = version;
}
public java.util.List getAddresses() {
return addresses;
}
public java.util.List getBillingAddressIds() {
return billingAddressIds;
}
@Nullable()
public java.lang.String getCompanyName() {
return companyName;
}
public java.time.ZonedDateTime getCreatedAt() {
return createdAt;
}
@Nullable()
public io.sphere.sdk.types.CustomFields getCustom() {
return custom;
}
@Nullable()
public io.sphere.sdk.models.Reference getCustomerGroup() {
return customerGroup;
}
@Nullable()
public java.lang.String getCustomerNumber() {
return customerNumber;
}
@Nullable()
public java.time.LocalDate getDateOfBirth() {
return dateOfBirth;
}
@Nullable()
public java.lang.String getDefaultBillingAddressId() {
return defaultBillingAddressId;
}
@Nullable()
public java.lang.String getDefaultShippingAddressId() {
return defaultShippingAddressId;
}
public java.lang.String getEmail() {
return email;
}
@JsonProperty("isEmailVerified")
public java.lang.Boolean isEmailVerified() {
return emailVerified;
}
@Nullable()
public java.lang.String getExternalId() {
return externalId;
}
@Nullable()
public java.lang.String getFirstName() {
return firstName;
}
public java.lang.String getId() {
return id;
}
public java.time.ZonedDateTime getLastModifiedAt() {
return lastModifiedAt;
}
@Nullable()
public java.lang.String getLastName() {
return lastName;
}
@Nullable()
public java.util.Locale getLocale() {
return locale;
}
@Nullable()
public java.lang.String getMiddleName() {
return middleName;
}
public java.lang.String getPassword() {
return password;
}
public java.util.List getShippingAddressIds() {
return shippingAddressIds;
}
@Nullable()
public java.lang.String getTitle() {
return title;
}
@Nullable()
public java.lang.String getVatId() {
return vatId;
}
public java.lang.Long getVersion() {
return version;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy