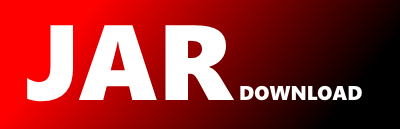
io.sphere.sdk.reviews.ReviewDraftBuilder Maven / Gradle / Ivy
/*
This class has been generated by class io.sphere.sdk.annotations.processors.ResourceDraftValueAnnotationProcessor
induced by the annotation io.sphere.sdk.annotations.ResourceDraftValue.
in the source class io.sphere.sdk.reviews.ReviewDraft.
*/
package io.sphere.sdk.reviews;
import javax.annotation.Nullable;
import io.sphere.sdk.models.*;
import java.util.*;
import io.sphere.sdk.utils.*;
import com.fasterxml.jackson.annotation.*;
import io.sphere.sdk.models.Builder;
import com.fasterxml.jackson.annotation.JsonCreator;
/** Builder for {@link ReviewDraft}. */
public final class ReviewDraftBuilder extends io.sphere.sdk.models.Base implements Builder {
@Nullable()
private java.lang.String authorName;
@Nullable()
private io.sphere.sdk.types.CustomFieldsDraft custom;
@Nullable()
private io.sphere.sdk.models.ResourceIdentifier customer;
@Nullable()
private java.lang.String key;
@Nullable()
private java.util.Locale locale;
@Nullable()
private java.lang.Integer rating;
@Nullable()
private io.sphere.sdk.models.ResourceIdentifier state;
@Nullable()
private io.sphere.sdk.models.ResourceIdentifier> target;
@Nullable()
private java.lang.String text;
@Nullable()
private java.lang.String title;
@Nullable()
private java.lang.String uniquenessValue;
@JsonCreator()
ReviewDraftBuilder(final java.lang.String authorName, final io.sphere.sdk.types.CustomFieldsDraft custom, final io.sphere.sdk.models.ResourceIdentifier customer, final java.lang.String key, final java.util.Locale locale, final java.lang.Integer rating, final io.sphere.sdk.models.ResourceIdentifier state, final io.sphere.sdk.models.ResourceIdentifier> target, final java.lang.String text, final java.lang.String title, final java.lang.String uniquenessValue) {
this.authorName = authorName;
this.custom = custom;
this.customer = customer;
this.key = key;
this.locale = locale;
this.rating = rating;
this.state = state;
this.target = target;
this.text = text;
this.title = title;
this.uniquenessValue = uniquenessValue;
}
public ReviewDraftBuilder authorName(@Nullable() final java.lang.String authorName) {
this.authorName = authorName;
return this;
}
public ReviewDraftBuilder custom(@Nullable() final io.sphere.sdk.types.CustomFieldsDraft custom) {
this.custom = custom;
return this;
}
public ReviewDraftBuilder customer(@Nullable() final io.sphere.sdk.models.ResourceIdentifier customer) {
this.customer = customer;
return this;
}
public ReviewDraftBuilder key(@Nullable() final java.lang.String key) {
this.key = key;
return this;
}
public ReviewDraftBuilder locale(@Nullable() final java.util.Locale locale) {
this.locale = locale;
return this;
}
public ReviewDraftBuilder rating(@Nullable() final java.lang.Integer rating) {
this.rating = rating;
return this;
}
public ReviewDraftBuilder state(@Nullable() final io.sphere.sdk.models.ResourceIdentifier state) {
this.state = state;
return this;
}
public ReviewDraftBuilder target(@Nullable() final io.sphere.sdk.models.ResourceIdentifier> target) {
this.target = target;
return this;
}
public ReviewDraftBuilder text(@Nullable() final java.lang.String text) {
this.text = text;
return this;
}
public ReviewDraftBuilder title(@Nullable() final java.lang.String title) {
this.title = title;
return this;
}
public ReviewDraftBuilder uniquenessValue(@Nullable() final java.lang.String uniquenessValue) {
this.uniquenessValue = uniquenessValue;
return this;
}
public ReviewDraftDsl build() {
return new ReviewDraftDsl(authorName, custom, customer, key, locale, rating, state, target, text, title, uniquenessValue);
}
public static ReviewDraftBuilder ofTitle(final java.lang.String title) {
return new ReviewDraftBuilder(null, null, null, null, null, null, null, null, null, title, null);
}
public static ReviewDraftBuilder ofText(final java.lang.String text) {
return new ReviewDraftBuilder(null, null, null, null, null, null, null, null, text, null, null);
}
public static ReviewDraftBuilder ofRating(final java.lang.Integer rating) {
return new ReviewDraftBuilder(null, null, null, null, null, rating, null, null, null, null, null);
}
public static ReviewDraftBuilder of(final ReviewDraft template) {
return new ReviewDraftBuilder(template.getAuthorName(), template.getCustom(), template.getCustomer(), template.getKey(), template.getLocale(), template.getRating(), template.getState(), template.getTarget(), template.getText(), template.getTitle(), template.getUniquenessValue());
}
public ReviewDraftBuilder target(@Nullable final ResourceIdentifiable> target) {
this.target = Optional.ofNullable(target).map(ResourceIdentifiable::toResourceIdentifier).orElse(null);
return this;
}
public ReviewDraftBuilder state(@Nullable final ResourceIdentifiable state) {
this.state = Optional.ofNullable(state).map(ResourceIdentifiable::toResourceIdentifier).orElse(null);
return this;
}
public ReviewDraftBuilder customer(@Nullable final ResourceIdentifiable customer) {
this.customer = Optional.ofNullable(customer).map(ResourceIdentifiable::toResourceIdentifier).orElse(null);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy