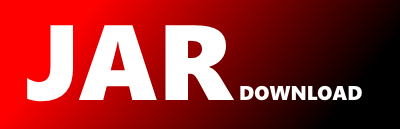
io.sphere.sdk.customers.CustomerDraftDsl Maven / Gradle / Ivy
/*
This class has been generated by class io.sphere.sdk.annotations.processors.ResourceDraftValueAnnotationProcessor
induced by the annotation io.sphere.sdk.annotations.ResourceDraftValue.
in the source class io.sphere.sdk.customers.CustomerDraft.
*/
package io.sphere.sdk.customers;
import javax.annotation.Nullable;
import io.sphere.sdk.models.*;
import java.util.*;
import io.sphere.sdk.utils.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.annotation.JsonCreator;
import io.sphere.sdk.customers.CustomerDraftBuilder;
public final class CustomerDraftDsl extends io.sphere.sdk.models.Base implements CustomerDraft {
@Nullable()
private final java.lang.String customerNumber;
private final java.lang.String email;
@Nullable()
private final java.lang.String firstName;
@Nullable()
private final java.lang.String lastName;
@Nullable()
private final java.lang.String middleName;
private final java.lang.String password;
@Nullable()
private final java.lang.String title;
@Nullable()
private final java.lang.String externalId;
@Nullable()
private final java.lang.String anonymousCartId;
@Nullable()
private final java.lang.String anonymousId;
@Nullable()
private final java.lang.String companyName;
@Nullable()
private final io.sphere.sdk.models.Reference customerGroup;
@Nullable()
private final java.time.LocalDate dateOfBirth;
@Nullable()
private final java.lang.Integer defaultBillingAddress;
@Nullable()
private final java.lang.Integer defaultShippingAddress;
@JsonProperty("isEmailVerified")
private final java.lang.Boolean emailVerified;
@Nullable()
private final java.lang.String vatId;
@Nullable()
private final java.util.List addresses;
@Nullable()
private final io.sphere.sdk.types.CustomFieldsDraft custom;
@Nullable()
private final java.util.Locale locale;
@Nullable()
private final java.util.List billingAddresses;
@Nullable()
private final java.util.List shippingAddresses;
@JsonCreator()
CustomerDraftDsl(final java.util.List addresses, final java.lang.String anonymousCartId, final java.lang.String anonymousId, final java.util.List billingAddresses, final java.lang.String companyName, final io.sphere.sdk.types.CustomFieldsDraft custom, final io.sphere.sdk.models.Reference customerGroup, final java.lang.String customerNumber, final java.time.LocalDate dateOfBirth, final java.lang.Integer defaultBillingAddress, final java.lang.Integer defaultShippingAddress, final java.lang.String email, @com.fasterxml.jackson.annotation.JsonProperty("isEmailVerified") final java.lang.Boolean emailVerified, final java.lang.String externalId, final java.lang.String firstName, final java.lang.String lastName, final java.util.Locale locale, final java.lang.String middleName, final java.lang.String password, final java.util.List shippingAddresses, final java.lang.String title, final java.lang.String vatId) {
this.addresses = addresses;
this.anonymousCartId = anonymousCartId;
this.anonymousId = anonymousId;
this.billingAddresses = billingAddresses;
this.companyName = companyName;
this.custom = custom;
this.customerGroup = customerGroup;
this.customerNumber = customerNumber;
this.dateOfBirth = dateOfBirth;
this.defaultBillingAddress = defaultBillingAddress;
this.defaultShippingAddress = defaultShippingAddress;
this.email = email;
this.emailVerified = emailVerified;
this.externalId = externalId;
this.firstName = firstName;
this.lastName = lastName;
this.locale = locale;
this.middleName = middleName;
this.password = password;
this.shippingAddresses = shippingAddresses;
this.title = title;
this.vatId = vatId;
if (!isValidAddressIndex(addresses, defaultBillingAddress)
|| !isValidAddressIndex(addresses, defaultShippingAddress)) {
throw new IllegalArgumentException("The defaultBillingAddress and defaultShippingAddress cannot contain an index which is not in the address list");
}
}
@Nullable()
public java.util.List getAddresses() {
return addresses;
}
@Nullable()
public java.lang.String getAnonymousCartId() {
return anonymousCartId;
}
@Nullable()
public java.lang.String getAnonymousId() {
return anonymousId;
}
@Nullable()
public java.util.List getBillingAddresses() {
return billingAddresses;
}
@Nullable()
public java.lang.String getCompanyName() {
return companyName;
}
@Nullable()
public io.sphere.sdk.types.CustomFieldsDraft getCustom() {
return custom;
}
@Nullable()
public io.sphere.sdk.models.Reference getCustomerGroup() {
return customerGroup;
}
@Nullable()
public java.lang.String getCustomerNumber() {
return customerNumber;
}
@Nullable()
public java.time.LocalDate getDateOfBirth() {
return dateOfBirth;
}
@Nullable()
public java.lang.Integer getDefaultBillingAddress() {
return defaultBillingAddress;
}
@Nullable()
public java.lang.Integer getDefaultShippingAddress() {
return defaultShippingAddress;
}
public java.lang.String getEmail() {
return email;
}
@JsonProperty("isEmailVerified")
public java.lang.Boolean isEmailVerified() {
return emailVerified;
}
@Nullable()
public java.lang.String getExternalId() {
return externalId;
}
@Nullable()
public java.lang.String getFirstName() {
return firstName;
}
@Nullable()
public java.lang.String getLastName() {
return lastName;
}
@Nullable()
public java.util.Locale getLocale() {
return locale;
}
@Nullable()
public java.lang.String getMiddleName() {
return middleName;
}
public java.lang.String getPassword() {
return password;
}
@Nullable()
public java.util.List getShippingAddresses() {
return shippingAddresses;
}
@Nullable()
public java.lang.String getTitle() {
return title;
}
@Nullable()
public java.lang.String getVatId() {
return vatId;
}
public CustomerDraftDsl withCustomerNumber(@Nullable() final java.lang.String customerNumber) {
return newBuilder().customerNumber(customerNumber).build();
}
public CustomerDraftDsl withEmail(final java.lang.String email) {
return newBuilder().email(email).build();
}
public CustomerDraftDsl withFirstName(@Nullable() final java.lang.String firstName) {
return newBuilder().firstName(firstName).build();
}
public CustomerDraftDsl withLastName(@Nullable() final java.lang.String lastName) {
return newBuilder().lastName(lastName).build();
}
public CustomerDraftDsl withMiddleName(@Nullable() final java.lang.String middleName) {
return newBuilder().middleName(middleName).build();
}
public CustomerDraftDsl withPassword(final java.lang.String password) {
return newBuilder().password(password).build();
}
public CustomerDraftDsl withTitle(@Nullable() final java.lang.String title) {
return newBuilder().title(title).build();
}
public CustomerDraftDsl withExternalId(@Nullable() final java.lang.String externalId) {
return newBuilder().externalId(externalId).build();
}
public CustomerDraftDsl withAnonymousCartId(@Nullable() final java.lang.String anonymousCartId) {
return newBuilder().anonymousCartId(anonymousCartId).build();
}
public CustomerDraftDsl withAnonymousId(@Nullable() final java.lang.String anonymousId) {
return newBuilder().anonymousId(anonymousId).build();
}
public CustomerDraftDsl withCompanyName(@Nullable() final java.lang.String companyName) {
return newBuilder().companyName(companyName).build();
}
public CustomerDraftDsl withCustomerGroup(@Nullable() final io.sphere.sdk.models.Referenceable customerGroup) {
return newBuilder().customerGroup(Optional.ofNullable(customerGroup).map(Referenceable::toReference).orElse(null)).build();
}
public CustomerDraftDsl withDateOfBirth(@Nullable() final java.time.LocalDate dateOfBirth) {
return newBuilder().dateOfBirth(dateOfBirth).build();
}
public CustomerDraftDsl withDefaultBillingAddress(@Nullable() final java.lang.Integer defaultBillingAddress) {
return newBuilder().defaultBillingAddress(defaultBillingAddress).build();
}
public CustomerDraftDsl withDefaultShippingAddress(@Nullable() final java.lang.Integer defaultShippingAddress) {
return newBuilder().defaultShippingAddress(defaultShippingAddress).build();
}
public CustomerDraftDsl withEmailVerified(final java.lang.Boolean emailVerified) {
return newBuilder().emailVerified(emailVerified).build();
}
public CustomerDraftDsl withIsEmailVerified(final java.lang.Boolean emailVerified) {
return newBuilder().emailVerified(emailVerified).build();
}
public CustomerDraftDsl withVatId(@Nullable() final java.lang.String vatId) {
return newBuilder().vatId(vatId).build();
}
public CustomerDraftDsl withAddresses(@Nullable() final java.util.List addresses) {
return newBuilder().addresses(addresses).build();
}
public CustomerDraftDsl withCustom(@Nullable() final io.sphere.sdk.types.CustomFieldsDraft custom) {
return newBuilder().custom(custom).build();
}
public CustomerDraftDsl withLocale(@Nullable() final java.util.Locale locale) {
return newBuilder().locale(locale).build();
}
public CustomerDraftDsl withBillingAddresses(@Nullable() final java.util.List billingAddresses) {
return newBuilder().billingAddresses(billingAddresses).build();
}
public CustomerDraftDsl withShippingAddresses(@Nullable() final java.util.List shippingAddresses) {
return newBuilder().shippingAddresses(shippingAddresses).build();
}
private CustomerDraftBuilder newBuilder() {
return new CustomerDraftBuilder(addresses, anonymousCartId, anonymousId, billingAddresses, companyName, custom, customerGroup, customerNumber, dateOfBirth, defaultBillingAddress, defaultShippingAddress, email, emailVerified, externalId, firstName, lastName, locale, middleName, password, shippingAddresses, title, vatId);
}
public static CustomerDraftDsl of(final java.lang.String email, final java.lang.String password) {
return new CustomerDraftDsl(null, null, null, null, null, null, null, null, null, null, null, email, null, null, null, null, null, null, password, null, null, null);
}
public static CustomerDraftDsl of(final CustomerDraft template) {
return new CustomerDraftDsl(template.getAddresses(), template.getAnonymousCartId(), template.getAnonymousId(), template.getBillingAddresses(), template.getCompanyName(), template.getCustom(), template.getCustomerGroup(), template.getCustomerNumber(), template.getDateOfBirth(), template.getDefaultBillingAddress(), template.getDefaultShippingAddress(), template.getEmail(), template.isEmailVerified(), template.getExternalId(), template.getFirstName(), template.getLastName(), template.getLocale(), template.getMiddleName(), template.getPassword(), template.getShippingAddresses(), template.getTitle(), template.getVatId());
}
public static CustomerDraftDsl of(final CustomerName customerName, final String email, final String password) {
return CustomerDraftBuilder.of(customerName, email, password).build();
}
public CustomerDraftDsl withCart(final io.sphere.sdk.carts.Cart cart) {
Objects.requireNonNull(cart);
return withAnonymousCartId(cart.getId());
}
private static boolean isValidAddressIndex(final List addresses, final Integer addressIndex) {
return Optional.ofNullable(addressIndex).map(i -> i < addresses.size() && i >= 0).orElse(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy