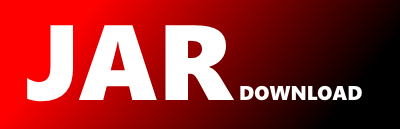
io.sphere.sdk.carts.CartDraftBuilder Maven / Gradle / Ivy
package io.sphere.sdk.carts;
import com.neovisionaries.i18n.CountryCode;
import io.sphere.sdk.models.Address;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.models.Referenceable;
import io.sphere.sdk.shippingmethods.ShippingMethod;
import io.sphere.sdk.types.CustomFieldsDraft;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Locale;
import java.util.Optional;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.money.CurrencyUnit;
/**
* Builder for {@link CartDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.carts.CartDraft"
)
public final class CartDraftBuilder extends Base implements Builder {
@Nullable
private String anonymousId;
@Nullable
private Address billingAddress;
@Nullable
private CountryCode country;
private CurrencyUnit currency;
@Nullable
private CustomFieldsDraft custom;
@Nullable
private List customLineItems;
@Nullable
private String customerEmail;
@Nullable
private String customerId;
@Nullable
private Integer deleteDaysAfterLastModification;
@Nullable
private InventoryMode inventoryMode;
@Nullable
private List lineItems;
@Nullable
private Locale locale;
@Nullable
private Address shippingAddress;
@Nullable
private Reference shippingMethod;
@Nullable
private TaxMode taxMode;
@Nullable
private RoundingMode taxRoundingMode;
CartDraftBuilder() {
}
CartDraftBuilder(@Nullable final String anonymousId, @Nullable final Address billingAddress,
@Nullable final CountryCode country, final CurrencyUnit currency,
@Nullable final CustomFieldsDraft custom,
@Nullable final List customLineItems,
@Nullable final String customerEmail, @Nullable final String customerId,
@Nullable final Integer deleteDaysAfterLastModification,
@Nullable final InventoryMode inventoryMode, @Nullable final List lineItems,
@Nullable final Locale locale, @Nullable final Address shippingAddress,
@Nullable final Reference shippingMethod, @Nullable final TaxMode taxMode,
@Nullable final RoundingMode taxRoundingMode) {
this.anonymousId = anonymousId;
this.billingAddress = billingAddress;
this.country = country;
this.currency = currency;
this.custom = custom;
this.customLineItems = customLineItems;
this.customerEmail = customerEmail;
this.customerId = customerId;
this.deleteDaysAfterLastModification = deleteDaysAfterLastModification;
this.inventoryMode = inventoryMode;
this.lineItems = lineItems;
this.locale = locale;
this.shippingAddress = shippingAddress;
this.shippingMethod = shippingMethod;
this.taxMode = taxMode;
this.taxRoundingMode = taxRoundingMode;
}
/**
* Sets the {@code anonymousId} property of this builder.
*
* @param anonymousId the value for {@link CartDraft#getAnonymousId()}
* @return this builder
*/
public CartDraftBuilder anonymousId(@Nullable final String anonymousId) {
this.anonymousId = anonymousId;
return this;
}
/**
* Sets the {@code billingAddress} property of this builder.
*
* @param billingAddress the value for {@link CartDraft#getBillingAddress()}
* @return this builder
*/
public CartDraftBuilder billingAddress(@Nullable final Address billingAddress) {
this.billingAddress = billingAddress;
return this;
}
/**
* Sets the {@code country} property of this builder.
*
* @param country the value for {@link CartDraft#getCountry()}
* @return this builder
*/
public CartDraftBuilder country(@Nullable final CountryCode country) {
this.country = country;
return this;
}
/**
* Sets the {@code currency} property of this builder.
*
* @param currency the value for {@link CartDraft#getCurrency()}
* @return this builder
*/
public CartDraftBuilder currency(final CurrencyUnit currency) {
this.currency = currency;
return this;
}
/**
* Sets the {@code custom} property of this builder.
*
* @param custom the value for {@link CartDraft#getCustom()}
* @return this builder
*/
public CartDraftBuilder custom(@Nullable final CustomFieldsDraft custom) {
this.custom = custom;
return this;
}
/**
* Sets the {@code customLineItems} property of this builder.
*
* @param customLineItems the value for {@link CartDraft#getCustomLineItems()}
* @return this builder
*/
public CartDraftBuilder customLineItems(@Nullable final List customLineItems) {
this.customLineItems = customLineItems;
return this;
}
/**
* Sets the {@code customerEmail} property of this builder.
*
* @param customerEmail the value for {@link CartDraft#getCustomerEmail()}
* @return this builder
*/
public CartDraftBuilder customerEmail(@Nullable final String customerEmail) {
this.customerEmail = customerEmail;
return this;
}
/**
* Sets the {@code customerId} property of this builder.
*
* @param customerId the value for {@link CartDraft#getCustomerId()}
* @return this builder
*/
public CartDraftBuilder customerId(@Nullable final String customerId) {
this.customerId = customerId;
return this;
}
/**
* Sets the {@code deleteDaysAfterLastModification} property of this builder.
*
* @param deleteDaysAfterLastModification the value for {@link CartDraft#getDeleteDaysAfterLastModification()}
* @return this builder
*/
public CartDraftBuilder deleteDaysAfterLastModification(@Nullable final Integer deleteDaysAfterLastModification) {
this.deleteDaysAfterLastModification = deleteDaysAfterLastModification;
return this;
}
/**
* Sets the {@code inventoryMode} property of this builder.
*
* @param inventoryMode the value for {@link CartDraft#getInventoryMode()}
* @return this builder
*/
public CartDraftBuilder inventoryMode(@Nullable final InventoryMode inventoryMode) {
this.inventoryMode = inventoryMode;
return this;
}
/**
* Sets the {@code lineItems} property of this builder.
*
* @param lineItems the value for {@link CartDraft#getLineItems()}
* @return this builder
*/
public CartDraftBuilder lineItems(@Nullable final List lineItems) {
this.lineItems = lineItems;
return this;
}
/**
* Sets the {@code locale} property of this builder.
*
* @param locale the value for {@link CartDraft#getLocale()}
* @return this builder
*/
public CartDraftBuilder locale(@Nullable final Locale locale) {
this.locale = locale;
return this;
}
/**
* Sets the {@code shippingAddress} property of this builder.
*
* @param shippingAddress the value for {@link CartDraft#getShippingAddress()}
* @return this builder
*/
public CartDraftBuilder shippingAddress(@Nullable final Address shippingAddress) {
this.shippingAddress = shippingAddress;
return this;
}
/**
* Sets the {@code shippingMethod} property of this builder.
*
* @param shippingMethod the value for {@link CartDraft#getShippingMethod()}
* @return this builder
*/
public CartDraftBuilder shippingMethod(@Nullable final Referenceable shippingMethod) {
this.shippingMethod = Optional.ofNullable(shippingMethod).map(Referenceable::toReference).orElse(null);;
return this;
}
/**
* Sets the {@code taxMode} property of this builder.
*
* @param taxMode the value for {@link CartDraft#getTaxMode()}
* @return this builder
*/
public CartDraftBuilder taxMode(@Nullable final TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Sets the {@code taxRoundingMode} property of this builder.
*
* @param taxRoundingMode the value for {@link CartDraft#getTaxRoundingMode()}
* @return this builder
*/
public CartDraftBuilder taxRoundingMode(@Nullable final RoundingMode taxRoundingMode) {
this.taxRoundingMode = taxRoundingMode;
return this;
}
@Nullable
public String getAnonymousId() {
return anonymousId;
}
@Nullable
public Address getBillingAddress() {
return billingAddress;
}
@Nullable
public CountryCode getCountry() {
return country;
}
public CurrencyUnit getCurrency() {
return currency;
}
@Nullable
public CustomFieldsDraft getCustom() {
return custom;
}
@Nullable
public List getCustomLineItems() {
return customLineItems;
}
@Nullable
public String getCustomerEmail() {
return customerEmail;
}
@Nullable
public String getCustomerId() {
return customerId;
}
@Nullable
public Integer getDeleteDaysAfterLastModification() {
return deleteDaysAfterLastModification;
}
@Nullable
public InventoryMode getInventoryMode() {
return inventoryMode;
}
@Nullable
public List getLineItems() {
return lineItems;
}
@Nullable
public Locale getLocale() {
return locale;
}
@Nullable
public Address getShippingAddress() {
return shippingAddress;
}
@Nullable
public Reference getShippingMethod() {
return shippingMethod;
}
@Nullable
public TaxMode getTaxMode() {
return taxMode;
}
@Nullable
public RoundingMode getTaxRoundingMode() {
return taxRoundingMode;
}
/**
* Creates a new instance of {@code CartDraftDsl} with the values of this builder.
*
* @return the instance
*/
public CartDraftDsl build() {
return new CartDraftDsl(anonymousId, billingAddress, country, currency, custom, customLineItems, customerEmail, customerId, deleteDaysAfterLastModification, inventoryMode, lineItems, locale, shippingAddress, shippingMethod, taxMode, taxRoundingMode);
}
/**
* Creates a builder initialized with the given values.
*
* @param currency initial value for the {@link CartDraft#getCurrency()} property
* @return new builder initialized with the given values
*/
public static CartDraftBuilder of(final CurrencyUnit currency) {
return new CartDraftBuilder(null, null, null, currency, null, null, null, null, null, null, null, null, null, null, null, null);
}
/**
* Creates a builder initialized with the fields of the template parameter.
*
* @param template the template
* @return a new builder initialized from the template
*/
public static CartDraftBuilder of(final CartDraft template) {
return new CartDraftBuilder(template.getAnonymousId(), template.getBillingAddress(), template.getCountry(), template.getCurrency(), template.getCustom(), template.getCustomLineItems(), template.getCustomerEmail(), template.getCustomerId(), template.getDeleteDaysAfterLastModification(), template.getInventoryMode(), template.getLineItems(), template.getLocale(), template.getShippingAddress(), template.getShippingMethod(), template.getTaxMode(), template.getTaxRoundingMode());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy