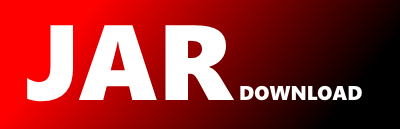
io.sphere.sdk.carts.CartDraftDsl Maven / Gradle / Ivy
/*
This class has been generated by class io.sphere.sdk.annotations.processors.ResourceDraftValueAnnotationProcessor
induced by the annotation io.sphere.sdk.annotations.ResourceDraftValue.
in the source class io.sphere.sdk.carts.CartDraft.
*/
package io.sphere.sdk.carts;
import javax.annotation.Nullable;
import io.sphere.sdk.models.*;
import java.util.*;
import io.sphere.sdk.utils.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.annotation.JsonCreator;
import io.sphere.sdk.carts.CartDraftBuilder;
public final class CartDraftDsl extends io.sphere.sdk.models.Base implements CartDraft {
private final javax.money.CurrencyUnit currency;
@Nullable()
private final java.lang.String customerId;
@Nullable()
private final com.neovisionaries.i18n.CountryCode country;
@Nullable()
private final io.sphere.sdk.carts.InventoryMode inventoryMode;
@Nullable()
private final java.lang.String customerEmail;
@Nullable()
private final io.sphere.sdk.models.Address billingAddress;
@Nullable()
private final java.util.List customLineItems;
@Nullable()
private final java.util.List lineItems;
@Nullable()
private final io.sphere.sdk.models.Address shippingAddress;
@Nullable()
private final io.sphere.sdk.models.Reference shippingMethod;
@Nullable()
private final io.sphere.sdk.types.CustomFieldsDraft custom;
@Nullable()
private final io.sphere.sdk.carts.TaxMode taxMode;
@Nullable()
private final java.lang.String anonymousId;
@Nullable()
private final java.util.Locale locale;
@Nullable()
private final java.lang.Integer deleteDaysAfterLastModification;
@Nullable()
private final io.sphere.sdk.carts.RoundingMode taxRoundingMode;
@JsonCreator()
CartDraftDsl(final java.lang.String anonymousId, final io.sphere.sdk.models.Address billingAddress, final com.neovisionaries.i18n.CountryCode country, final javax.money.CurrencyUnit currency, final io.sphere.sdk.types.CustomFieldsDraft custom, final java.util.List customLineItems, final java.lang.String customerEmail, final java.lang.String customerId, final java.lang.Integer deleteDaysAfterLastModification, final io.sphere.sdk.carts.InventoryMode inventoryMode, final java.util.List lineItems, final java.util.Locale locale, final io.sphere.sdk.models.Address shippingAddress, final io.sphere.sdk.models.Reference shippingMethod, final io.sphere.sdk.carts.TaxMode taxMode, final io.sphere.sdk.carts.RoundingMode taxRoundingMode) {
this.anonymousId = anonymousId;
this.billingAddress = billingAddress;
this.country = country;
this.currency = currency;
this.custom = custom;
this.customLineItems = customLineItems;
this.customerEmail = customerEmail;
this.customerId = customerId;
this.deleteDaysAfterLastModification = deleteDaysAfterLastModification;
this.inventoryMode = inventoryMode;
this.lineItems = lineItems;
this.locale = locale;
this.shippingAddress = shippingAddress;
this.shippingMethod = shippingMethod;
this.taxMode = taxMode;
this.taxRoundingMode = taxRoundingMode;
}
@Nullable()
public java.lang.String getAnonymousId() {
return anonymousId;
}
@Nullable()
public io.sphere.sdk.models.Address getBillingAddress() {
return billingAddress;
}
@Nullable()
public com.neovisionaries.i18n.CountryCode getCountry() {
return country;
}
public javax.money.CurrencyUnit getCurrency() {
return currency;
}
@Nullable()
public io.sphere.sdk.types.CustomFieldsDraft getCustom() {
return custom;
}
@Nullable()
public java.util.List getCustomLineItems() {
return customLineItems;
}
@Nullable()
public java.lang.String getCustomerEmail() {
return customerEmail;
}
@Nullable()
public java.lang.String getCustomerId() {
return customerId;
}
@Nullable()
public java.lang.Integer getDeleteDaysAfterLastModification() {
return deleteDaysAfterLastModification;
}
@Nullable()
public io.sphere.sdk.carts.InventoryMode getInventoryMode() {
return inventoryMode;
}
@Nullable()
public java.util.List getLineItems() {
return lineItems;
}
@Nullable()
public java.util.Locale getLocale() {
return locale;
}
@Nullable()
public io.sphere.sdk.models.Address getShippingAddress() {
return shippingAddress;
}
@Nullable()
public io.sphere.sdk.models.Reference getShippingMethod() {
return shippingMethod;
}
@Nullable()
public io.sphere.sdk.carts.TaxMode getTaxMode() {
return taxMode;
}
@Nullable()
public io.sphere.sdk.carts.RoundingMode getTaxRoundingMode() {
return taxRoundingMode;
}
public CartDraftDsl withCurrency(final javax.money.CurrencyUnit currency) {
return newBuilder().currency(currency).build();
}
public CartDraftDsl withCustomerId(@Nullable() final java.lang.String customerId) {
return newBuilder().customerId(customerId).build();
}
public CartDraftDsl withCountry(@Nullable() final com.neovisionaries.i18n.CountryCode country) {
return newBuilder().country(country).build();
}
public CartDraftDsl withInventoryMode(@Nullable() final io.sphere.sdk.carts.InventoryMode inventoryMode) {
return newBuilder().inventoryMode(inventoryMode).build();
}
public CartDraftDsl withCustomerEmail(@Nullable() final java.lang.String customerEmail) {
return newBuilder().customerEmail(customerEmail).build();
}
public CartDraftDsl withBillingAddress(@Nullable() final io.sphere.sdk.models.Address billingAddress) {
return newBuilder().billingAddress(billingAddress).build();
}
public CartDraftDsl withCustomLineItems(@Nullable() final java.util.List customLineItems) {
return newBuilder().customLineItems(customLineItems).build();
}
public CartDraftDsl withLineItems(@Nullable() final java.util.List lineItems) {
return newBuilder().lineItems(lineItems).build();
}
public CartDraftDsl withShippingAddress(@Nullable() final io.sphere.sdk.models.Address shippingAddress) {
return newBuilder().shippingAddress(shippingAddress).build();
}
public CartDraftDsl withShippingMethod(@Nullable() final io.sphere.sdk.models.Referenceable shippingMethod) {
return newBuilder().shippingMethod(Optional.ofNullable(shippingMethod).map(Referenceable::toReference).orElse(null)).build();
}
public CartDraftDsl withCustom(@Nullable() final io.sphere.sdk.types.CustomFieldsDraft custom) {
return newBuilder().custom(custom).build();
}
public CartDraftDsl withTaxMode(@Nullable() final io.sphere.sdk.carts.TaxMode taxMode) {
return newBuilder().taxMode(taxMode).build();
}
public CartDraftDsl withAnonymousId(@Nullable() final java.lang.String anonymousId) {
return newBuilder().anonymousId(anonymousId).build();
}
public CartDraftDsl withLocale(@Nullable() final java.util.Locale locale) {
return newBuilder().locale(locale).build();
}
public CartDraftDsl withDeleteDaysAfterLastModification(@Nullable() final java.lang.Integer deleteDaysAfterLastModification) {
return newBuilder().deleteDaysAfterLastModification(deleteDaysAfterLastModification).build();
}
public CartDraftDsl withTaxRoundingMode(@Nullable() final io.sphere.sdk.carts.RoundingMode taxRoundingMode) {
return newBuilder().taxRoundingMode(taxRoundingMode).build();
}
private CartDraftBuilder newBuilder() {
return new CartDraftBuilder(anonymousId, billingAddress, country, currency, custom, customLineItems, customerEmail, customerId, deleteDaysAfterLastModification, inventoryMode, lineItems, locale, shippingAddress, shippingMethod, taxMode, taxRoundingMode);
}
public static CartDraftDsl of(final javax.money.CurrencyUnit currency) {
return new CartDraftDsl(null, null, null, currency, null, null, null, null, null, null, null, null, null, null, null, null);
}
public static CartDraftDsl of(final CartDraft template) {
return new CartDraftDsl(template.getAnonymousId(), template.getBillingAddress(), template.getCountry(), template.getCurrency(), template.getCustom(), template.getCustomLineItems(), template.getCustomerEmail(), template.getCustomerId(), template.getDeleteDaysAfterLastModification(), template.getInventoryMode(), template.getLineItems(), template.getLocale(), template.getShippingAddress(), template.getShippingMethod(), template.getTaxMode(), template.getTaxRoundingMode());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy