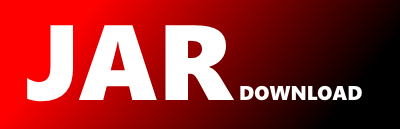
io.sphere.sdk.shippingmethods.ShippingMethodDraftBuilder Maven / Gradle / Ivy
package io.sphere.sdk.shippingmethods;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.models.Referenceable;
import io.sphere.sdk.taxcategories.TaxCategory;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
import javax.annotation.Nullable;
/**
* Builder for {@link ShippingMethodDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.shippingmethods.ShippingMethodDraft"
)
public final class ShippingMethodDraftBuilder extends Base implements Builder {
private Boolean _default;
@Nullable
private String description;
private String name;
private Reference taxCategory;
private List zoneRates;
ShippingMethodDraftBuilder() {
}
ShippingMethodDraftBuilder(final Boolean _default, @Nullable final String description,
final String name, final Reference taxCategory, final List zoneRates) {
this._default = _default;
this.description = description;
this.name = name;
this.taxCategory = taxCategory;
this.zoneRates = zoneRates;
}
/**
* Sets the {@code default} property of this builder.
*
* @param _default the value for {@link ShippingMethodDraft#isDefault()}
* @return this builder
*/
public ShippingMethodDraftBuilder _default(final Boolean _default) {
this._default = _default;
return this;
}
/**
* Sets the {@code default} property of this builder.
*
* @param _default the value for {@link ShippingMethodDraft#isDefault()}
* @return this builder
*/
public ShippingMethodDraftBuilder isDefault(final Boolean _default) {
this._default = _default;
return this;
}
/**
* Sets the {@code description} property of this builder.
*
* @param description the value for {@link ShippingMethodDraft#getDescription()}
* @return this builder
*/
public ShippingMethodDraftBuilder description(@Nullable final String description) {
this.description = description;
return this;
}
/**
* Sets the {@code name} property of this builder.
*
* @param name the value for {@link ShippingMethodDraft#getName()}
* @return this builder
*/
public ShippingMethodDraftBuilder name(final String name) {
this.name = name;
return this;
}
/**
* Sets the {@code taxCategory} property of this builder.
*
* @param taxCategory the value for {@link ShippingMethodDraft#getTaxCategory()}
* @return this builder
*/
public ShippingMethodDraftBuilder taxCategory(final Referenceable taxCategory) {
this.taxCategory = Optional.ofNullable(taxCategory).map(Referenceable::toReference).orElse(null);;
return this;
}
/**
* Sets the {@code zoneRates} property of this builder.
*
* @param zoneRates the value for {@link ShippingMethodDraft#getZoneRates()}
* @return this builder
*/
public ShippingMethodDraftBuilder zoneRates(final List zoneRates) {
this.zoneRates = zoneRates;
return this;
}
/**
* Creates a new instance of {@code ShippingMethodDraftDsl} with the values of this builder.
*
* @return the instance
*/
public ShippingMethodDraftDsl build() {
return new ShippingMethodDraftDsl(_default, description, name, taxCategory, zoneRates);
}
/**
* Creates a builder initialized with the given values.
*
* @param name initial value for the {@link ShippingMethodDraft#getName()} property
* @param description initial value for the {@link ShippingMethodDraft#getDescription()} property
* @param taxCategory initial value for the {@link ShippingMethodDraft#getTaxCategory()} property
* @param zoneRates initial value for the {@link ShippingMethodDraft#getZoneRates()} property
* @param _default initial value for the {@link ShippingMethodDraft#isDefault()} property
* @return new builder initialized with the given values
*/
public static ShippingMethodDraftBuilder of(final String name, final String description,
final Reference taxCategory, final List zoneRates,
final Boolean _default) {
return new ShippingMethodDraftBuilder(_default, description, name, taxCategory, zoneRates);
}
/**
* Creates a builder initialized with the given values.
*
* @param name initial value for the {@link ShippingMethodDraft#getName()} property
* @param taxCategory initial value for the {@link ShippingMethodDraft#getTaxCategory()} property
* @param zoneRates initial value for the {@link ShippingMethodDraft#getZoneRates()} property
* @param _default initial value for the {@link ShippingMethodDraft#isDefault()} property
* @return new builder initialized with the given values
*/
public static ShippingMethodDraftBuilder of(final String name,
final Reference taxCategory, final List zoneRates,
final Boolean _default) {
return new ShippingMethodDraftBuilder(_default, null, name, taxCategory, zoneRates);
}
/**
* Creates a builder initialized with the fields of the template parameter.
*
* @param template the template
* @return a new builder initialized from the template
*/
public static ShippingMethodDraftBuilder of(final ShippingMethodDraft template) {
return new ShippingMethodDraftBuilder(template.isDefault(), template.getDescription(), template.getName(), template.getTaxCategory(), template.getZoneRates());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy