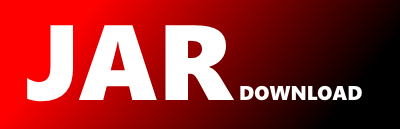
io.sphere.sdk.carts.CartImpl Maven / Gradle / Ivy
package io.sphere.sdk.carts;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.neovisionaries.i18n.CountryCode;
import io.sphere.sdk.cartdiscounts.CartDiscount;
import io.sphere.sdk.customergroups.CustomerGroup;
import io.sphere.sdk.discountcodes.DiscountCodeInfo;
import io.sphere.sdk.models.Address;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.types.CustomFields;
import java.lang.Integer;
import java.lang.Long;
import java.lang.String;
import java.time.ZonedDateTime;
import java.util.List;
import java.util.Locale;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.money.MonetaryAmount;
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.ResourceValueImplGenerator",
comments = "Generated from: io.sphere.sdk.carts.Cart"
)
final class CartImpl extends Base implements Cart {
@Nullable
private String anonymousId;
@Nullable
private Address billingAddress;
private CartState cartState;
@Nullable
private CountryCode country;
private ZonedDateTime createdAt;
@Nullable
private CustomFields custom;
private List customLineItems;
@Nullable
private String customerEmail;
@Nullable
private Reference customerGroup;
@Nullable
private String customerId;
@Nullable
private Integer deleteDaysAfterLastModification;
private List discountCodes;
private String id;
private InventoryMode inventoryMode;
private ZonedDateTime lastModifiedAt;
private List lineItems;
@Nullable
private Locale locale;
@Nullable
private PaymentInfo paymentInfo;
@Nullable
private List> refusedGifts;
@Nullable
private Address shippingAddress;
@Nullable
private CartShippingInfo shippingInfo;
@Nullable
private ShippingRateInput shippingRateInput;
private TaxCalculationMode taxCalculationMode;
private TaxMode taxMode;
private RoundingMode taxRoundingMode;
@Nullable
private TaxedPrice taxedPrice;
private MonetaryAmount totalPrice;
private Long version;
@JsonCreator
CartImpl(@Nullable final String anonymousId, @Nullable final Address billingAddress,
final CartState cartState, @Nullable final CountryCode country, final ZonedDateTime createdAt,
@Nullable final CustomFields custom, final List customLineItems,
@Nullable final String customerEmail, @Nullable final Reference customerGroup,
@Nullable final String customerId, @Nullable final Integer deleteDaysAfterLastModification,
final List discountCodes, final String id,
final InventoryMode inventoryMode, final ZonedDateTime lastModifiedAt,
final List lineItems, @Nullable final Locale locale,
@Nullable final PaymentInfo paymentInfo,
@Nullable final List> refusedGifts,
@Nullable final Address shippingAddress, @Nullable final CartShippingInfo shippingInfo,
@Nullable final ShippingRateInput shippingRateInput,
final TaxCalculationMode taxCalculationMode, final TaxMode taxMode,
final RoundingMode taxRoundingMode, @Nullable final TaxedPrice taxedPrice,
final MonetaryAmount totalPrice, final Long version) {
this.anonymousId = anonymousId;
this.billingAddress = billingAddress;
this.cartState = cartState;
this.country = country;
this.createdAt = createdAt;
this.custom = custom;
this.customLineItems = customLineItems;
this.customerEmail = customerEmail;
this.customerGroup = customerGroup;
this.customerId = customerId;
this.deleteDaysAfterLastModification = deleteDaysAfterLastModification;
this.discountCodes = discountCodes;
this.id = id;
this.inventoryMode = inventoryMode;
this.lastModifiedAt = lastModifiedAt;
this.lineItems = lineItems;
this.locale = locale;
this.paymentInfo = paymentInfo;
this.refusedGifts = refusedGifts;
this.shippingAddress = shippingAddress;
this.shippingInfo = shippingInfo;
this.shippingRateInput = shippingRateInput;
this.taxCalculationMode = taxCalculationMode;
this.taxMode = taxMode;
this.taxRoundingMode = taxRoundingMode;
this.taxedPrice = taxedPrice;
this.totalPrice = totalPrice;
this.version = version;
}
@Nullable
public String getAnonymousId() {
return anonymousId;
}
@Nullable
public Address getBillingAddress() {
return billingAddress;
}
public CartState getCartState() {
return cartState;
}
@Nullable
public CountryCode getCountry() {
return country;
}
public ZonedDateTime getCreatedAt() {
return createdAt;
}
@Nullable
public CustomFields getCustom() {
return custom;
}
public List getCustomLineItems() {
return customLineItems;
}
@Nullable
public String getCustomerEmail() {
return customerEmail;
}
@Nullable
public Reference getCustomerGroup() {
return customerGroup;
}
@Nullable
public String getCustomerId() {
return customerId;
}
@Nullable
public Integer getDeleteDaysAfterLastModification() {
return deleteDaysAfterLastModification;
}
public List getDiscountCodes() {
return discountCodes;
}
public String getId() {
return id;
}
public InventoryMode getInventoryMode() {
return inventoryMode;
}
public ZonedDateTime getLastModifiedAt() {
return lastModifiedAt;
}
public List getLineItems() {
return lineItems;
}
@Nullable
public Locale getLocale() {
return locale;
}
@Nullable
public PaymentInfo getPaymentInfo() {
return paymentInfo;
}
@Nullable
public List> getRefusedGifts() {
return refusedGifts;
}
@Nullable
public Address getShippingAddress() {
return shippingAddress;
}
@Nullable
public CartShippingInfo getShippingInfo() {
return shippingInfo;
}
@Nullable
public ShippingRateInput getShippingRateInput() {
return shippingRateInput;
}
public TaxCalculationMode getTaxCalculationMode() {
return taxCalculationMode;
}
public TaxMode getTaxMode() {
return taxMode;
}
public RoundingMode getTaxRoundingMode() {
return taxRoundingMode;
}
@Nullable
public TaxedPrice getTaxedPrice() {
return taxedPrice;
}
public MonetaryAmount getTotalPrice() {
return totalPrice;
}
public Long getVersion() {
return version;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy