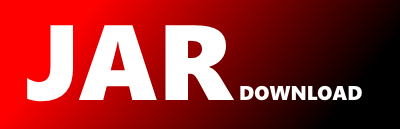
io.sphere.sdk.carts.CartImpl Maven / Gradle / Ivy
/*
This class has been generated by class io.sphere.sdk.annotations.processors.ResourceValueAnnotationProcessor
induced by the annotation io.sphere.sdk.annotations.ResourceValue.
in the source class io.sphere.sdk.carts.Cart.
*/
package io.sphere.sdk.carts;
import javax.annotation.Nullable;
import io.sphere.sdk.models.*;
import java.util.*;
import io.sphere.sdk.utils.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.annotation.JsonCreator;
final class CartImpl extends io.sphere.sdk.models.Base implements Cart {
private final io.sphere.sdk.carts.CartState cartState;
private final io.sphere.sdk.carts.InventoryMode inventoryMode;
@Nullable()
private final io.sphere.sdk.carts.CartShippingInfo shippingInfo;
@Nullable()
private final io.sphere.sdk.models.Address billingAddress;
@Nullable()
private final com.neovisionaries.i18n.CountryCode country;
@Nullable()
private final java.lang.String customerEmail;
@Nullable()
private final io.sphere.sdk.models.Reference customerGroup;
@Nullable()
private final java.lang.String customerId;
private final java.util.List customLineItems;
private final java.util.List lineItems;
@Nullable()
private final io.sphere.sdk.models.Address shippingAddress;
@Nullable()
private final io.sphere.sdk.carts.TaxedPrice taxedPrice;
private final javax.money.MonetaryAmount totalPrice;
private final java.util.List discountCodes;
@Nullable()
private final io.sphere.sdk.types.CustomFields custom;
@Nullable()
private final io.sphere.sdk.carts.PaymentInfo paymentInfo;
private final io.sphere.sdk.carts.TaxMode taxMode;
@Nullable()
private final java.lang.String anonymousId;
@Nullable()
private final java.util.Locale locale;
private final java.lang.String id;
private final java.lang.Long version;
private final java.time.ZonedDateTime createdAt;
private final java.time.ZonedDateTime lastModifiedAt;
@JsonCreator()
CartImpl(final java.lang.String anonymousId, final io.sphere.sdk.models.Address billingAddress, final io.sphere.sdk.carts.CartState cartState, final com.neovisionaries.i18n.CountryCode country, final java.time.ZonedDateTime createdAt, final io.sphere.sdk.types.CustomFields custom, final java.util.List customLineItems, final java.lang.String customerEmail, final io.sphere.sdk.models.Reference customerGroup, final java.lang.String customerId, final java.util.List discountCodes, final java.lang.String id, final io.sphere.sdk.carts.InventoryMode inventoryMode, final java.time.ZonedDateTime lastModifiedAt, final java.util.List lineItems, final java.util.Locale locale, final io.sphere.sdk.carts.PaymentInfo paymentInfo, final io.sphere.sdk.models.Address shippingAddress, final io.sphere.sdk.carts.CartShippingInfo shippingInfo, final io.sphere.sdk.carts.TaxMode taxMode, final io.sphere.sdk.carts.TaxedPrice taxedPrice, final javax.money.MonetaryAmount totalPrice, final java.lang.Long version) {
this.anonymousId = anonymousId;
this.billingAddress = billingAddress;
this.cartState = cartState;
this.country = country;
this.createdAt = createdAt;
this.custom = custom;
this.customLineItems = customLineItems;
this.customerEmail = customerEmail;
this.customerGroup = customerGroup;
this.customerId = customerId;
this.discountCodes = discountCodes;
this.id = id;
this.inventoryMode = inventoryMode;
this.lastModifiedAt = lastModifiedAt;
this.lineItems = lineItems;
this.locale = locale;
this.paymentInfo = paymentInfo;
this.shippingAddress = shippingAddress;
this.shippingInfo = shippingInfo;
this.taxMode = taxMode;
this.taxedPrice = taxedPrice;
this.totalPrice = totalPrice;
this.version = version;
}
@Nullable()
public java.lang.String getAnonymousId() {
return anonymousId;
}
@Nullable()
public io.sphere.sdk.models.Address getBillingAddress() {
return billingAddress;
}
public io.sphere.sdk.carts.CartState getCartState() {
return cartState;
}
@Nullable()
public com.neovisionaries.i18n.CountryCode getCountry() {
return country;
}
public java.time.ZonedDateTime getCreatedAt() {
return createdAt;
}
@Nullable()
public io.sphere.sdk.types.CustomFields getCustom() {
return custom;
}
public java.util.List getCustomLineItems() {
return customLineItems;
}
@Nullable()
public java.lang.String getCustomerEmail() {
return customerEmail;
}
@Nullable()
public io.sphere.sdk.models.Reference getCustomerGroup() {
return customerGroup;
}
@Nullable()
public java.lang.String getCustomerId() {
return customerId;
}
public java.util.List getDiscountCodes() {
return discountCodes;
}
public java.lang.String getId() {
return id;
}
public io.sphere.sdk.carts.InventoryMode getInventoryMode() {
return inventoryMode;
}
public java.time.ZonedDateTime getLastModifiedAt() {
return lastModifiedAt;
}
public java.util.List getLineItems() {
return lineItems;
}
@Nullable()
public java.util.Locale getLocale() {
return locale;
}
@Nullable()
public io.sphere.sdk.carts.PaymentInfo getPaymentInfo() {
return paymentInfo;
}
@Nullable()
public io.sphere.sdk.models.Address getShippingAddress() {
return shippingAddress;
}
@Nullable()
public io.sphere.sdk.carts.CartShippingInfo getShippingInfo() {
return shippingInfo;
}
public io.sphere.sdk.carts.TaxMode getTaxMode() {
return taxMode;
}
@Nullable()
public io.sphere.sdk.carts.TaxedPrice getTaxedPrice() {
return taxedPrice;
}
public javax.money.MonetaryAmount getTotalPrice() {
return totalPrice;
}
public java.lang.Long getVersion() {
return version;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy