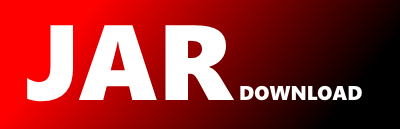
io.sphere.sdk.payments.PaymentDraftBuilder Maven / Gradle / Ivy
/*
This class has been generated by class io.sphere.sdk.annotations.processors.ResourceDraftValueAnnotationProcessor
induced by the annotation io.sphere.sdk.annotations.ResourceDraftValue.
in the source class io.sphere.sdk.payments.PaymentDraft.
*/
package io.sphere.sdk.payments;
import javax.annotation.Nullable;
import io.sphere.sdk.models.*;
import java.util.*;
import io.sphere.sdk.utils.*;
import com.fasterxml.jackson.annotation.*;
import io.sphere.sdk.models.Builder;
import com.fasterxml.jackson.annotation.JsonCreator;
/** Builder for {@link PaymentDraft}. */
public final class PaymentDraftBuilder extends io.sphere.sdk.models.Base implements Builder, io.sphere.sdk.types.CustomDraft {
@Nullable()
private io.sphere.sdk.models.Reference customer;
@Nullable()
private java.lang.String externalId;
@Nullable()
private java.lang.String interfaceId;
private javax.money.MonetaryAmount amountPlanned;
@Nullable()
private javax.money.MonetaryAmount amountAuthorized;
@Nullable()
private java.time.ZonedDateTime authorizedUntil;
@Nullable()
private javax.money.MonetaryAmount amountPaid;
@Nullable()
private javax.money.MonetaryAmount amountRefunded;
@Nullable()
private io.sphere.sdk.payments.PaymentMethodInfo paymentMethodInfo;
@Nullable()
private io.sphere.sdk.types.CustomFieldsDraft custom;
@Nullable()
private io.sphere.sdk.payments.PaymentStatus paymentStatus;
@Nullable()
private java.util.List transactions;
@Nullable()
private java.util.List interfaceInteractions;
@JsonCreator()
PaymentDraftBuilder(final javax.money.MonetaryAmount amountAuthorized, final javax.money.MonetaryAmount amountPaid, final javax.money.MonetaryAmount amountPlanned, final javax.money.MonetaryAmount amountRefunded, final java.time.ZonedDateTime authorizedUntil, final io.sphere.sdk.types.CustomFieldsDraft custom, final io.sphere.sdk.models.Reference customer, final java.lang.String externalId, final java.lang.String interfaceId, final java.util.List interfaceInteractions, final io.sphere.sdk.payments.PaymentMethodInfo paymentMethodInfo, final io.sphere.sdk.payments.PaymentStatus paymentStatus, final java.util.List transactions) {
this.amountAuthorized = amountAuthorized;
this.amountPaid = amountPaid;
this.amountPlanned = amountPlanned;
this.amountRefunded = amountRefunded;
this.authorizedUntil = authorizedUntil;
this.custom = custom;
this.customer = customer;
this.externalId = externalId;
this.interfaceId = interfaceId;
this.interfaceInteractions = interfaceInteractions;
this.paymentMethodInfo = paymentMethodInfo;
this.paymentStatus = paymentStatus;
this.transactions = transactions;
}
public PaymentDraftBuilder customer(@Nullable() final io.sphere.sdk.models.Referenceable customer) {
this.customer = Optional.ofNullable(customer).map(Referenceable::toReference).orElse(null);
return this;
}
public PaymentDraftBuilder externalId(@Nullable() final java.lang.String externalId) {
this.externalId = externalId;
return this;
}
public PaymentDraftBuilder interfaceId(@Nullable() final java.lang.String interfaceId) {
this.interfaceId = interfaceId;
return this;
}
public PaymentDraftBuilder amountPlanned(final javax.money.MonetaryAmount amountPlanned) {
this.amountPlanned = amountPlanned;
return this;
}
public PaymentDraftBuilder amountAuthorized(@Nullable() final javax.money.MonetaryAmount amountAuthorized) {
this.amountAuthorized = amountAuthorized;
return this;
}
public PaymentDraftBuilder authorizedUntil(@Nullable() final java.time.ZonedDateTime authorizedUntil) {
this.authorizedUntil = authorizedUntil;
return this;
}
public PaymentDraftBuilder amountPaid(@Nullable() final javax.money.MonetaryAmount amountPaid) {
this.amountPaid = amountPaid;
return this;
}
public PaymentDraftBuilder amountRefunded(@Nullable() final javax.money.MonetaryAmount amountRefunded) {
this.amountRefunded = amountRefunded;
return this;
}
public PaymentDraftBuilder paymentMethodInfo(@Nullable() final io.sphere.sdk.payments.PaymentMethodInfo paymentMethodInfo) {
this.paymentMethodInfo = paymentMethodInfo;
return this;
}
public PaymentDraftBuilder custom(@Nullable() final io.sphere.sdk.types.CustomFieldsDraft custom) {
this.custom = custom;
return this;
}
public PaymentDraftBuilder paymentStatus(@Nullable() final io.sphere.sdk.payments.PaymentStatus paymentStatus) {
this.paymentStatus = paymentStatus;
return this;
}
public PaymentDraftBuilder transactions(@Nullable() final java.util.List transactions) {
this.transactions = transactions;
return this;
}
public PaymentDraftBuilder interfaceInteractions(@Nullable() final java.util.List interfaceInteractions) {
this.interfaceInteractions = interfaceInteractions;
return this;
}
public PaymentDraftDsl build() {
return new PaymentDraftDsl(amountAuthorized, amountPaid, amountPlanned, amountRefunded, authorizedUntil, custom, customer, externalId, interfaceId, interfaceInteractions, paymentMethodInfo, paymentStatus, transactions);
}
public static PaymentDraftBuilder of(final javax.money.MonetaryAmount amountPlanned) {
return new PaymentDraftBuilder(null, null, amountPlanned, null, null, null, null, null, null, null, null, null, null);
}
public static PaymentDraftBuilder of(final PaymentDraft template) {
return new PaymentDraftBuilder(template.getAmountAuthorized(), template.getAmountPaid(), template.getAmountPlanned(), template.getAmountRefunded(), template.getAuthorizedUntil(), template.getCustom(), template.getCustomer(), template.getExternalId(), template.getInterfaceId(), template.getInterfaceInteractions(), template.getPaymentMethodInfo(), template.getPaymentStatus(), template.getTransactions());
}
@Nullable()
public javax.money.MonetaryAmount getAmountAuthorized() {
return amountAuthorized;
}
@Nullable()
public javax.money.MonetaryAmount getAmountPaid() {
return amountPaid;
}
public javax.money.MonetaryAmount getAmountPlanned() {
return amountPlanned;
}
@Nullable()
public javax.money.MonetaryAmount getAmountRefunded() {
return amountRefunded;
}
@Nullable()
public java.time.ZonedDateTime getAuthorizedUntil() {
return authorizedUntil;
}
@Nullable()
public io.sphere.sdk.types.CustomFieldsDraft getCustom() {
return custom;
}
@Nullable()
public io.sphere.sdk.models.Reference getCustomer() {
return customer;
}
@Nullable()
public java.lang.String getExternalId() {
return externalId;
}
@Nullable()
public java.lang.String getInterfaceId() {
return interfaceId;
}
@Nullable()
public java.util.List getInterfaceInteractions() {
return interfaceInteractions;
}
@Nullable()
public io.sphere.sdk.payments.PaymentMethodInfo getPaymentMethodInfo() {
return paymentMethodInfo;
}
@Nullable()
public io.sphere.sdk.payments.PaymentStatus getPaymentStatus() {
return paymentStatus;
}
@Nullable()
public java.util.List getTransactions() {
return transactions;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy