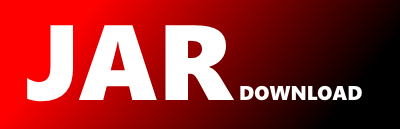
io.sphere.sdk.apiclient.ApiClientDraftBuilderBase Maven / Gradle / Ivy
package io.sphere.sdk.apiclient;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import java.lang.Integer;
import java.lang.String;
import java.lang.SuppressWarnings;
import javax.annotation.Generated;
import javax.annotation.Nullable;
/**
* Abstract base builder for {@link ApiClientDraft} which needs to be extended to add additional methods.
* Subclasses have to provide the same non-default constructor as this class.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.apiclient.ApiClientDraft"
)
abstract class ApiClientDraftBuilderBase extends Base implements Builder {
@Nullable
Integer deleteDaysAfterCreation;
String name;
String scope;
protected ApiClientDraftBuilderBase() {
}
protected ApiClientDraftBuilderBase(@Nullable final Integer deleteDaysAfterCreation,
final String name, final String scope) {
this.deleteDaysAfterCreation = deleteDaysAfterCreation;
this.name = name;
this.scope = scope;
}
/**
* Sets the {@code deleteDaysAfterCreation} property of this builder.
*
* @param deleteDaysAfterCreation the value for {@link ApiClientDraft#getDeleteDaysAfterCreation()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T deleteDaysAfterCreation(@Nullable final Integer deleteDaysAfterCreation) {
this.deleteDaysAfterCreation = deleteDaysAfterCreation;
return (T) this;
}
/**
* Sets the {@code name} property of this builder.
*
* @param name the value for {@link ApiClientDraft#getName()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T name(final String name) {
this.name = name;
return (T) this;
}
/**
* Sets the {@code scope} property of this builder.
*
* @param scope the value for {@link ApiClientDraft#getScope()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T scope(final String scope) {
this.scope = scope;
return (T) this;
}
@Nullable
public Integer getDeleteDaysAfterCreation() {
return deleteDaysAfterCreation;
}
public String getName() {
return name;
}
public String getScope() {
return scope;
}
/**
* Creates a new instance of {@code ApiClientDraftDsl} with the values of this builder.
*
* @return the instance
*/
public ApiClientDraftDsl build() {
return new ApiClientDraftDsl(deleteDaysAfterCreation, name, scope);
}
/**
* Creates a new object initialized with the given values.
*
* @param name initial value for the {@link ApiClientDraft#getName()} property
* @param scope initial value for the {@link ApiClientDraft#getScope()} property
* @return new object initialized with the given values
*/
public static ApiClientDraftBuilder of(final String name, final String scope) {
return new ApiClientDraftBuilder(null, name, scope);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static ApiClientDraftBuilder of(final ApiClientDraft template) {
return new ApiClientDraftBuilder(template.getDeleteDaysAfterCreation(), template.getName(), template.getScope());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy