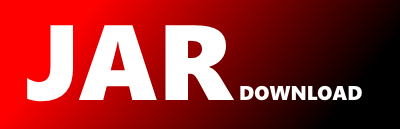
io.sphere.sdk.customers.CustomerImpl Maven / Gradle / Ivy
package io.sphere.sdk.customers;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.sphere.sdk.customergroups.CustomerGroup;
import io.sphere.sdk.models.Address;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.KeyReference;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.stores.Store;
import io.sphere.sdk.types.CustomFields;
import java.lang.Boolean;
import java.lang.Long;
import java.lang.String;
import java.time.LocalDate;
import java.time.ZonedDateTime;
import java.util.List;
import java.util.Locale;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.ResourceValueImplGenerator",
comments = "Generated from: io.sphere.sdk.customers.Customer"
)
final class CustomerImpl extends Base implements Customer {
private List addresses;
private List billingAddressIds;
@Nullable
private String companyName;
private ZonedDateTime createdAt;
@Nullable
private CustomFields custom;
@Nullable
private Reference customerGroup;
@Nullable
private String customerNumber;
@Nullable
private LocalDate dateOfBirth;
@Nullable
private String defaultBillingAddressId;
@Nullable
private String defaultShippingAddressId;
private String email;
@JsonProperty("isEmailVerified")
private Boolean emailVerified;
@Nullable
private String externalId;
@Nullable
private String firstName;
private String id;
@Nullable
private String key;
private ZonedDateTime lastModifiedAt;
@Nullable
private String lastName;
@Nullable
private Locale locale;
@Nullable
private String middleName;
private String password;
@Nullable
private String salutation;
private List shippingAddressIds;
@Nullable
private List> stores;
@Nullable
private String title;
@Nullable
private String vatId;
private Long version;
@JsonCreator
CustomerImpl(final List addresses, final List billingAddressIds,
@Nullable final String companyName, final ZonedDateTime createdAt,
@Nullable final CustomFields custom, @Nullable final Reference customerGroup,
@Nullable final String customerNumber, @Nullable final LocalDate dateOfBirth,
@Nullable final String defaultBillingAddressId,
@Nullable final String defaultShippingAddressId, final String email,
@JsonProperty("isEmailVerified") final Boolean emailVerified,
@Nullable final String externalId, @Nullable final String firstName, final String id,
@Nullable final String key, final ZonedDateTime lastModifiedAt,
@Nullable final String lastName, @Nullable final Locale locale,
@Nullable final String middleName, final String password, @Nullable final String salutation,
final List shippingAddressIds, @Nullable final List> stores,
@Nullable final String title, @Nullable final String vatId, final Long version) {
this.addresses = addresses;
this.billingAddressIds = billingAddressIds;
this.companyName = companyName;
this.createdAt = createdAt;
this.custom = custom;
this.customerGroup = customerGroup;
this.customerNumber = customerNumber;
this.dateOfBirth = dateOfBirth;
this.defaultBillingAddressId = defaultBillingAddressId;
this.defaultShippingAddressId = defaultShippingAddressId;
this.email = email;
this.emailVerified = emailVerified;
this.externalId = externalId;
this.firstName = firstName;
this.id = id;
this.key = key;
this.lastModifiedAt = lastModifiedAt;
this.lastName = lastName;
this.locale = locale;
this.middleName = middleName;
this.password = password;
this.salutation = salutation;
this.shippingAddressIds = shippingAddressIds;
this.stores = stores;
this.title = title;
this.vatId = vatId;
this.version = version;
}
public List getAddresses() {
return addresses;
}
public List getBillingAddressIds() {
return billingAddressIds;
}
@Nullable
public String getCompanyName() {
return companyName;
}
public ZonedDateTime getCreatedAt() {
return createdAt;
}
@Nullable
public CustomFields getCustom() {
return custom;
}
@Nullable
public Reference getCustomerGroup() {
return customerGroup;
}
@Nullable
public String getCustomerNumber() {
return customerNumber;
}
@Nullable
public LocalDate getDateOfBirth() {
return dateOfBirth;
}
@Nullable
public String getDefaultBillingAddressId() {
return defaultBillingAddressId;
}
@Nullable
public String getDefaultShippingAddressId() {
return defaultShippingAddressId;
}
public String getEmail() {
return email;
}
@JsonProperty("isEmailVerified")
public Boolean isEmailVerified() {
return emailVerified;
}
@Nullable
public String getExternalId() {
return externalId;
}
@Nullable
public String getFirstName() {
return firstName;
}
public String getId() {
return id;
}
@Nullable
public String getKey() {
return key;
}
public ZonedDateTime getLastModifiedAt() {
return lastModifiedAt;
}
@Nullable
public String getLastName() {
return lastName;
}
@Nullable
public Locale getLocale() {
return locale;
}
@Nullable
public String getMiddleName() {
return middleName;
}
public String getPassword() {
return password;
}
@Nullable
public String getSalutation() {
return salutation;
}
public List getShippingAddressIds() {
return shippingAddressIds;
}
@Nullable
public List> getStores() {
return stores;
}
@Nullable
public String getTitle() {
return title;
}
@Nullable
public String getVatId() {
return vatId;
}
public Long getVersion() {
return version;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy