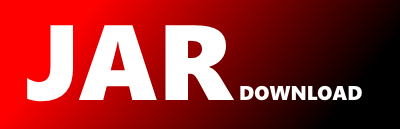
io.sphere.sdk.payments.PaymentDraftBuilder Maven / Gradle / Ivy
package io.sphere.sdk.payments;
import io.sphere.sdk.customers.Customer;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.models.Referenceable;
import io.sphere.sdk.types.CustomDraft;
import io.sphere.sdk.types.CustomFieldsDraft;
import io.sphere.sdk.utils.SphereInternalUtils;
import java.lang.Deprecated;
import java.lang.String;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.money.MonetaryAmount;
/**
* Builder for {@link PaymentDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.payments.PaymentDraft"
)
public final class PaymentDraftBuilder extends Base implements CustomDraft, Builder {
@Nullable
private MonetaryAmount amountAuthorized;
@Nullable
private MonetaryAmount amountPaid;
private MonetaryAmount amountPlanned;
@Nullable
private MonetaryAmount amountRefunded;
@Nullable
private String anonymousId;
@Nullable
private ZonedDateTime authorizedUntil;
@Nullable
private CustomFieldsDraft custom;
@Nullable
private Reference customer;
@Nullable
private String externalId;
@Nullable
private String interfaceId;
@Nullable
private List interfaceInteractions;
@Nullable
private String key;
@Nullable
private PaymentMethodInfo paymentMethodInfo;
@Nullable
private PaymentStatus paymentStatus;
@Nullable
private List transactions;
PaymentDraftBuilder() {
}
PaymentDraftBuilder(@Nullable final MonetaryAmount amountAuthorized,
@Nullable final MonetaryAmount amountPaid, final MonetaryAmount amountPlanned,
@Nullable final MonetaryAmount amountRefunded, @Nullable final String anonymousId,
@Nullable final ZonedDateTime authorizedUntil, @Nullable final CustomFieldsDraft custom,
@Nullable final Reference customer, @Nullable final String externalId,
@Nullable final String interfaceId,
@Nullable final List interfaceInteractions, @Nullable final String key,
@Nullable final PaymentMethodInfo paymentMethodInfo,
@Nullable final PaymentStatus paymentStatus,
@Nullable final List transactions) {
this.amountAuthorized = amountAuthorized;
this.amountPaid = amountPaid;
this.amountPlanned = amountPlanned;
this.amountRefunded = amountRefunded;
this.anonymousId = anonymousId;
this.authorizedUntil = authorizedUntil;
this.custom = custom;
this.customer = customer;
this.externalId = externalId;
this.interfaceId = interfaceId;
this.interfaceInteractions = interfaceInteractions;
this.key = key;
this.paymentMethodInfo = paymentMethodInfo;
this.paymentStatus = paymentStatus;
this.transactions = transactions;
}
/**
* Sets the {@code amountAuthorized} property of this builder.
*
* @param amountAuthorized the value for {@link PaymentDraft#getAmountAuthorized()}
* @return this builder
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftBuilder amountAuthorized(@Nullable final MonetaryAmount amountAuthorized) {
this.amountAuthorized = amountAuthorized;
return this;
}
/**
* Sets the {@code amountPaid} property of this builder.
*
* @param amountPaid the value for {@link PaymentDraft#getAmountPaid()}
* @return this builder
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftBuilder amountPaid(@Nullable final MonetaryAmount amountPaid) {
this.amountPaid = amountPaid;
return this;
}
/**
* Sets the {@code amountPlanned} property of this builder.
*
* @param amountPlanned the value for {@link PaymentDraft#getAmountPlanned()}
* @return this builder
*/
public PaymentDraftBuilder amountPlanned(final MonetaryAmount amountPlanned) {
this.amountPlanned = amountPlanned;
return this;
}
/**
* Sets the {@code amountRefunded} property of this builder.
*
* @param amountRefunded the value for {@link PaymentDraft#getAmountRefunded()}
* @return this builder
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftBuilder amountRefunded(@Nullable final MonetaryAmount amountRefunded) {
this.amountRefunded = amountRefunded;
return this;
}
/**
* Sets the {@code anonymousId} property of this builder.
*
* @param anonymousId the value for {@link PaymentDraft#getAnonymousId()}
* @return this builder
*/
public PaymentDraftBuilder anonymousId(@Nullable final String anonymousId) {
this.anonymousId = anonymousId;
return this;
}
/**
* Sets the {@code authorizedUntil} property of this builder.
*
* @param authorizedUntil the value for {@link PaymentDraft#getAuthorizedUntil()}
* @return this builder
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftBuilder authorizedUntil(@Nullable final ZonedDateTime authorizedUntil) {
this.authorizedUntil = authorizedUntil;
return this;
}
/**
* Sets the {@code custom} property of this builder.
*
* @param custom the value for {@link PaymentDraft#getCustom()}
* @return this builder
*/
public PaymentDraftBuilder custom(@Nullable final CustomFieldsDraft custom) {
this.custom = custom;
return this;
}
/**
* Sets the {@code customer} property of this builder.
*
* @param customer the value for {@link PaymentDraft#getCustomer()}
* @return this builder
*/
public PaymentDraftBuilder customer(@Nullable final Referenceable customer) {
this.customer = Optional.ofNullable(customer).map(Referenceable::toReference).orElse(null);;
return this;
}
/**
* Sets the {@code externalId} property of this builder.
*
* @param externalId the value for {@link PaymentDraft#getExternalId()}
* @return this builder
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftBuilder externalId(@Nullable final String externalId) {
this.externalId = externalId;
return this;
}
/**
* Sets the {@code interfaceId} property of this builder.
*
* @param interfaceId the value for {@link PaymentDraft#getInterfaceId()}
* @return this builder
*/
public PaymentDraftBuilder interfaceId(@Nullable final String interfaceId) {
this.interfaceId = interfaceId;
return this;
}
/**
* Sets the {@code interfaceInteractions} property of this builder.
*
* @param interfaceInteractions the value for {@link PaymentDraft#getInterfaceInteractions()}
* @return this builder
*/
public PaymentDraftBuilder interfaceInteractions(
@Nullable final List interfaceInteractions) {
this.interfaceInteractions = interfaceInteractions;
return this;
}
/**
* Sets the {@code key} property of this builder.
*
* @param key the value for {@link PaymentDraft#getKey()}
* @return this builder
*/
public PaymentDraftBuilder key(@Nullable final String key) {
this.key = key;
return this;
}
/**
* Sets the {@code paymentMethodInfo} property of this builder.
*
* @param paymentMethodInfo the value for {@link PaymentDraft#getPaymentMethodInfo()}
* @return this builder
*/
public PaymentDraftBuilder paymentMethodInfo(
@Nullable final PaymentMethodInfo paymentMethodInfo) {
this.paymentMethodInfo = paymentMethodInfo;
return this;
}
/**
* Sets the {@code paymentStatus} property of this builder.
*
* @param paymentStatus the value for {@link PaymentDraft#getPaymentStatus()}
* @return this builder
*/
public PaymentDraftBuilder paymentStatus(@Nullable final PaymentStatus paymentStatus) {
this.paymentStatus = paymentStatus;
return this;
}
/**
* Sets the {@code transactions} property of this builder.
*
* @param transactions the value for {@link PaymentDraft#getTransactions()}
* @return this builder
*/
public PaymentDraftBuilder transactions(@Nullable final List transactions) {
this.transactions = transactions;
return this;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public MonetaryAmount getAmountAuthorized() {
return amountAuthorized;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public MonetaryAmount getAmountPaid() {
return amountPaid;
}
public MonetaryAmount getAmountPlanned() {
return amountPlanned;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public MonetaryAmount getAmountRefunded() {
return amountRefunded;
}
@Nullable
public String getAnonymousId() {
return anonymousId;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public ZonedDateTime getAuthorizedUntil() {
return authorizedUntil;
}
@Nullable
public CustomFieldsDraft getCustom() {
return custom;
}
@Nullable
public Reference getCustomer() {
return customer;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public String getExternalId() {
return externalId;
}
@Nullable
public String getInterfaceId() {
return interfaceId;
}
@Nullable
public List getInterfaceInteractions() {
return interfaceInteractions;
}
@Nullable
public String getKey() {
return key;
}
@Nullable
public PaymentMethodInfo getPaymentMethodInfo() {
return paymentMethodInfo;
}
@Nullable
public PaymentStatus getPaymentStatus() {
return paymentStatus;
}
@Nullable
public List getTransactions() {
return transactions;
}
/**
* Concatenate {@code interfaceInteractions} parameter to the {@code interfaceInteractions} list property of this builder.
*
* @param interfaceInteractions the value for {@link PaymentDraft#getInterfaceInteractions()}
* @return this builder
*/
public PaymentDraftBuilder plusInterfaceInteractions(
final List interfaceInteractions) {
this.interfaceInteractions = SphereInternalUtils.listOf(Optional.ofNullable(this.interfaceInteractions).orElseGet(ArrayList::new), interfaceInteractions);
return this;
}
/**
* Concatenate {@code transactions} parameter to the {@code transactions} list property of this builder.
*
* @param transactions the value for {@link PaymentDraft#getTransactions()}
* @return this builder
*/
public PaymentDraftBuilder plusTransactions(final List transactions) {
this.transactions = SphereInternalUtils.listOf(Optional.ofNullable(this.transactions).orElseGet(ArrayList::new), transactions);
return this;
}
/**
* Adds {@code interfaceInteractions} parameter to the {@code interfaceInteractions} list property of this builder.
*
* @param interfaceInteractions the value of the element to add to {@link PaymentDraft#getInterfaceInteractions()}
* @return this builder
*/
public PaymentDraftBuilder plusInterfaceInteractions(
final CustomFieldsDraft interfaceInteractions) {
this.interfaceInteractions = SphereInternalUtils.listOf(Optional.ofNullable(this.interfaceInteractions).orElseGet(ArrayList::new), Collections.singletonList(interfaceInteractions));
return this;
}
/**
* Adds {@code transactions} parameter to the {@code transactions} list property of this builder.
*
* @param transactions the value of the element to add to {@link PaymentDraft#getTransactions()}
* @return this builder
*/
public PaymentDraftBuilder plusTransactions(final TransactionDraft transactions) {
this.transactions = SphereInternalUtils.listOf(Optional.ofNullable(this.transactions).orElseGet(ArrayList::new), Collections.singletonList(transactions));
return this;
}
/**
* Creates a new instance of {@code PaymentDraftDsl} with the values of this builder.
*
* @return the instance
*/
public PaymentDraftDsl build() {
return new PaymentDraftDsl(amountAuthorized, amountPaid, amountPlanned, amountRefunded, anonymousId, authorizedUntil, custom, customer, externalId, interfaceId, interfaceInteractions, key, paymentMethodInfo, paymentStatus, transactions);
}
/**
* Creates a new object initialized with the given values.
*
* @param amountPlanned initial value for the {@link PaymentDraft#getAmountPlanned()} property
* @return new object initialized with the given values
*/
public static PaymentDraftBuilder of(final MonetaryAmount amountPlanned) {
return new PaymentDraftBuilder(null, null, amountPlanned, null, null, null, null, null, null, null, null, null, null, null, null);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static PaymentDraftBuilder of(final PaymentDraft template) {
return new PaymentDraftBuilder(template.getAmountAuthorized(), template.getAmountPaid(), template.getAmountPlanned(), template.getAmountRefunded(), template.getAnonymousId(), template.getAuthorizedUntil(), template.getCustom(), template.getCustomer(), template.getExternalId(), template.getInterfaceId(), template.getInterfaceInteractions(), template.getKey(), template.getPaymentMethodInfo(), template.getPaymentStatus(), template.getTransactions());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy