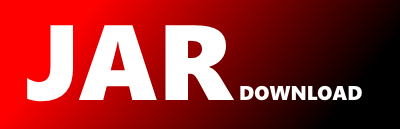
io.sphere.sdk.reviews.ReviewImpl Maven / Gradle / Ivy
package io.sphere.sdk.reviews;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.sphere.sdk.customers.Customer;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.states.State;
import io.sphere.sdk.types.CustomFields;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.String;
import java.time.ZonedDateTime;
import java.util.Locale;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.ResourceValueImplGenerator",
comments = "Generated from: io.sphere.sdk.reviews.Review"
)
final class ReviewImpl extends Base implements Review {
@Nullable
private String authorName;
private ZonedDateTime createdAt;
@Nullable
private CustomFields custom;
@Nullable
private Reference customer;
private String id;
@JsonProperty("includedInStatistics")
private Boolean includedInStatistics;
@Nullable
private String key;
private ZonedDateTime lastModifiedAt;
@Nullable
private Locale locale;
@Nullable
private Integer rating;
@Nullable
private Reference state;
@Nullable
private Reference> target;
@Nullable
private String text;
@Nullable
private String title;
@Nullable
private String uniquenessValue;
private Long version;
@JsonCreator
ReviewImpl(@Nullable final String authorName, final ZonedDateTime createdAt,
@Nullable final CustomFields custom, @Nullable final Reference customer,
final String id, @JsonProperty("includedInStatistics") final Boolean includedInStatistics,
@Nullable final String key, final ZonedDateTime lastModifiedAt, @Nullable final Locale locale,
@Nullable final Integer rating, @Nullable final Reference state,
@Nullable final Reference> target, @Nullable final String text,
@Nullable final String title, @Nullable final String uniquenessValue, final Long version) {
this.authorName = authorName;
this.createdAt = createdAt;
this.custom = custom;
this.customer = customer;
this.id = id;
this.includedInStatistics = includedInStatistics;
this.key = key;
this.lastModifiedAt = lastModifiedAt;
this.locale = locale;
this.rating = rating;
this.state = state;
this.target = target;
this.text = text;
this.title = title;
this.uniquenessValue = uniquenessValue;
this.version = version;
}
@Nullable
public String getAuthorName() {
return authorName;
}
public ZonedDateTime getCreatedAt() {
return createdAt;
}
@Nullable
public CustomFields getCustom() {
return custom;
}
@Nullable
public Reference getCustomer() {
return customer;
}
public String getId() {
return id;
}
@JsonProperty("includedInStatistics")
public Boolean isIncludedInStatistics() {
return includedInStatistics;
}
@Nullable
public String getKey() {
return key;
}
public ZonedDateTime getLastModifiedAt() {
return lastModifiedAt;
}
@Nullable
public Locale getLocale() {
return locale;
}
@Nullable
public Integer getRating() {
return rating;
}
@Nullable
public Reference getState() {
return state;
}
@Nullable
public Reference> getTarget() {
return target;
}
@Nullable
public String getText() {
return text;
}
@Nullable
public String getTitle() {
return title;
}
@Nullable
public String getUniquenessValue() {
return uniquenessValue;
}
public Long getVersion() {
return version;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy