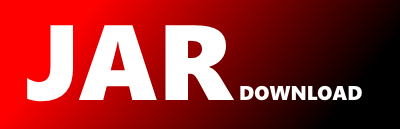
io.sphere.sdk.apiclient.ApiClientDraftDsl Maven / Gradle / Ivy
package io.sphere.sdk.apiclient;
import com.fasterxml.jackson.annotation.JsonCreator;
import io.sphere.sdk.models.Base;
import java.lang.Integer;
import java.lang.Long;
import java.lang.String;
import javax.annotation.Generated;
import javax.annotation.Nullable;
/**
* Dsl class for {@link ApiClientDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.ResourceDraftValueGenerator",
comments = "Generated from: io.sphere.sdk.apiclient.ApiClientDraft"
)
public final class ApiClientDraftDsl extends Base implements ApiClientDraft {
@Nullable
private Long accessTokenValiditySeconds;
@Nullable
private Integer deleteDaysAfterCreation;
private String name;
@Nullable
private Long refreshTokenValiditySeconds;
private String scope;
@JsonCreator
ApiClientDraftDsl(@Nullable final Long accessTokenValiditySeconds,
@Nullable final Integer deleteDaysAfterCreation, final String name,
@Nullable final Long refreshTokenValiditySeconds, final String scope) {
this.accessTokenValiditySeconds = accessTokenValiditySeconds;
this.deleteDaysAfterCreation = deleteDaysAfterCreation;
this.name = name;
this.refreshTokenValiditySeconds = refreshTokenValiditySeconds;
this.scope = scope;
}
@Nullable
public Long getAccessTokenValiditySeconds() {
return accessTokenValiditySeconds;
}
@Nullable
public Integer getDeleteDaysAfterCreation() {
return deleteDaysAfterCreation;
}
public String getName() {
return name;
}
@Nullable
public Long getRefreshTokenValiditySeconds() {
return refreshTokenValiditySeconds;
}
public String getScope() {
return scope;
}
/**
* Creates a new builder with the values of this object.
*
* @return new builder
*/
public ApiClientDraftBuilder newBuilder() {
return new ApiClientDraftBuilder(accessTokenValiditySeconds, deleteDaysAfterCreation, name, refreshTokenValiditySeconds, scope);
}
public ApiClientDraftDsl withAccessTokenValiditySeconds(
@Nullable final Long accessTokenValiditySeconds) {
return newBuilder().accessTokenValiditySeconds(accessTokenValiditySeconds).build();
}
public ApiClientDraftDsl withDeleteDaysAfterCreation(
@Nullable final Integer deleteDaysAfterCreation) {
return newBuilder().deleteDaysAfterCreation(deleteDaysAfterCreation).build();
}
public ApiClientDraftDsl withName(final String name) {
return newBuilder().name(name).build();
}
public ApiClientDraftDsl withRefreshTokenValiditySeconds(
@Nullable final Long refreshTokenValiditySeconds) {
return newBuilder().refreshTokenValiditySeconds(refreshTokenValiditySeconds).build();
}
public ApiClientDraftDsl withScope(final String scope) {
return newBuilder().scope(scope).build();
}
/**
* Creates a new object initialized with the given values.
*
* @param name initial value for the {@link ApiClientDraft#getName()} property
* @param scope initial value for the {@link ApiClientDraft#getScope()} property
* @return new object initialized with the given values
*/
public static ApiClientDraftDsl of(final String name, final String scope) {
return new ApiClientDraftDsl(null, null, name, null, scope);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static ApiClientDraftDsl of(final ApiClientDraft template) {
return new ApiClientDraftDsl(template.getAccessTokenValiditySeconds(), template.getDeleteDaysAfterCreation(), template.getName(), template.getRefreshTokenValiditySeconds(), template.getScope());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy