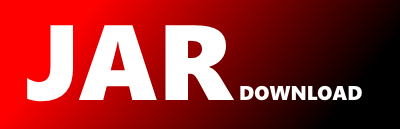
io.sphere.sdk.customers.CustomerDraftBuilderBase Maven / Gradle / Ivy
package io.sphere.sdk.customers;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.sphere.sdk.carts.Cart;
import io.sphere.sdk.customergroups.CustomerGroup;
import io.sphere.sdk.models.Address;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.models.ResourceIdentifier;
import io.sphere.sdk.stores.Store;
import io.sphere.sdk.types.CustomFieldsDraft;
import io.sphere.sdk.utils.SphereInternalUtils;
import java.lang.Boolean;
import java.lang.Deprecated;
import java.lang.Integer;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Locale;
import java.util.Optional;
import javax.annotation.Generated;
import javax.annotation.Nullable;
/**
* Abstract base builder for {@link CustomerDraft} which needs to be extended to add additional methods.
* Subclasses have to provide the same non-default constructor as this class.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.customers.CustomerDraft"
)
abstract class CustomerDraftBuilderBase extends Base implements Builder {
@Nullable
List addresses;
@Nullable
ResourceIdentifier anonymousCart;
@Nullable
String anonymousCartId;
@Nullable
String anonymousId;
@Nullable
AuthenticationMode authenticationMode;
@Nullable
List billingAddresses;
@Nullable
String companyName;
@Nullable
CustomFieldsDraft custom;
@Nullable
ResourceIdentifier customerGroup;
@Nullable
String customerNumber;
@Nullable
LocalDate dateOfBirth;
@Nullable
Integer defaultBillingAddress;
@Nullable
Integer defaultShippingAddress;
String email;
Boolean emailVerified;
@Nullable
String externalId;
@Nullable
String firstName;
@Nullable
String key;
@Nullable
String lastName;
@Nullable
Locale locale;
@Nullable
String middleName;
String password;
@Nullable
String salutation;
@Nullable
List shippingAddresses;
@Nullable
List> stores;
@Nullable
String title;
@Nullable
String vatId;
protected CustomerDraftBuilderBase() {
}
protected CustomerDraftBuilderBase(@Nullable final List addresses,
@Nullable final ResourceIdentifier anonymousCart,
@Nullable final String anonymousCartId, @Nullable final String anonymousId,
@Nullable final AuthenticationMode authenticationMode,
@Nullable final List billingAddresses, @Nullable final String companyName,
@Nullable final CustomFieldsDraft custom,
@Nullable final ResourceIdentifier customerGroup,
@Nullable final String customerNumber, @Nullable final LocalDate dateOfBirth,
@Nullable final Integer defaultBillingAddress, @Nullable final Integer defaultShippingAddress,
final String email, final Boolean emailVerified, @Nullable final String externalId,
@Nullable final String firstName, @Nullable final String key, @Nullable final String lastName,
@Nullable final Locale locale, @Nullable final String middleName, final String password,
@Nullable final String salutation, @Nullable final List shippingAddresses,
@Nullable final List> stores, @Nullable final String title,
@Nullable final String vatId) {
this.addresses = addresses;
this.anonymousCart = anonymousCart;
this.anonymousCartId = anonymousCartId;
this.anonymousId = anonymousId;
this.authenticationMode = authenticationMode;
this.billingAddresses = billingAddresses;
this.companyName = companyName;
this.custom = custom;
this.customerGroup = customerGroup;
this.customerNumber = customerNumber;
this.dateOfBirth = dateOfBirth;
this.defaultBillingAddress = defaultBillingAddress;
this.defaultShippingAddress = defaultShippingAddress;
this.email = email;
this.emailVerified = emailVerified;
this.externalId = externalId;
this.firstName = firstName;
this.key = key;
this.lastName = lastName;
this.locale = locale;
this.middleName = middleName;
this.password = password;
this.salutation = salutation;
this.shippingAddresses = shippingAddresses;
this.stores = stores;
this.title = title;
this.vatId = vatId;
}
/**
* Sets the {@code addresses} property of this builder.
*
* @param addresses the value for {@link CustomerDraft#getAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T addresses(@Nullable final List addresses) {
this.addresses = addresses;
return (T) this;
}
/**
* Sets the {@code anonymousCart} property of this builder.
*
* @param anonymousCart the value for {@link CustomerDraft#getAnonymousCart()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T anonymousCart(@Nullable final ResourceIdentifier anonymousCart) {
this.anonymousCart = anonymousCart;
return (T) this;
}
/**
* Sets the {@code anonymousCartId} property of this builder.
*
* @param anonymousCartId the value for {@link CustomerDraft#getAnonymousCartId()}
* @return this builder
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
@SuppressWarnings("unchecked")
public T anonymousCartId(@Nullable final String anonymousCartId) {
this.anonymousCartId = anonymousCartId;
return (T) this;
}
/**
* Sets the {@code anonymousId} property of this builder.
*
* @param anonymousId the value for {@link CustomerDraft#getAnonymousId()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T anonymousId(@Nullable final String anonymousId) {
this.anonymousId = anonymousId;
return (T) this;
}
/**
* Sets the {@code authenticationMode} property of this builder.
*
* @param authenticationMode the value for {@link CustomerDraft#getAuthenticationMode()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T authenticationMode(@Nullable final AuthenticationMode authenticationMode) {
this.authenticationMode = authenticationMode;
return (T) this;
}
/**
* Sets the {@code billingAddresses} property of this builder.
*
* @param billingAddresses the value for {@link CustomerDraft#getBillingAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T billingAddresses(@Nullable final List billingAddresses) {
this.billingAddresses = billingAddresses;
return (T) this;
}
/**
* Sets the {@code companyName} property of this builder.
*
* @param companyName the value for {@link CustomerDraft#getCompanyName()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T companyName(@Nullable final String companyName) {
this.companyName = companyName;
return (T) this;
}
/**
* Sets the {@code custom} property of this builder.
*
* @param custom the value for {@link CustomerDraft#getCustom()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T custom(@Nullable final CustomFieldsDraft custom) {
this.custom = custom;
return (T) this;
}
/**
* Sets the {@code customerGroup} property of this builder.
*
* @param customerGroup the value for {@link CustomerDraft#getCustomerGroup()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T customerGroup(@Nullable final ResourceIdentifier customerGroup) {
this.customerGroup = customerGroup;
return (T) this;
}
/**
* Sets the {@code customerNumber} property of this builder.
*
* @param customerNumber the value for {@link CustomerDraft#getCustomerNumber()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T customerNumber(@Nullable final String customerNumber) {
this.customerNumber = customerNumber;
return (T) this;
}
/**
* Sets the {@code dateOfBirth} property of this builder.
*
* @param dateOfBirth the value for {@link CustomerDraft#getDateOfBirth()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T dateOfBirth(@Nullable final LocalDate dateOfBirth) {
this.dateOfBirth = dateOfBirth;
return (T) this;
}
/**
* Sets the {@code defaultBillingAddress} property of this builder.
*
* @param defaultBillingAddress the value for {@link CustomerDraft#getDefaultBillingAddress()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T defaultBillingAddress(@Nullable final Integer defaultBillingAddress) {
this.defaultBillingAddress = defaultBillingAddress;
return (T) this;
}
/**
* Sets the {@code defaultShippingAddress} property of this builder.
*
* @param defaultShippingAddress the value for {@link CustomerDraft#getDefaultShippingAddress()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T defaultShippingAddress(@Nullable final Integer defaultShippingAddress) {
this.defaultShippingAddress = defaultShippingAddress;
return (T) this;
}
/**
* Sets the {@code email} property of this builder.
*
* @param email the value for {@link CustomerDraft#getEmail()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T email(final String email) {
this.email = email;
return (T) this;
}
/**
* Sets the {@code emailVerified} property of this builder.
*
* @param emailVerified the value for {@link CustomerDraft#isEmailVerified()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T emailVerified(final Boolean emailVerified) {
this.emailVerified = emailVerified;
return (T) this;
}
/**
* Sets the {@code emailVerified} property of this builder.
*
* @param emailVerified the value for {@link CustomerDraft#isEmailVerified()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T isEmailVerified(final Boolean emailVerified) {
this.emailVerified = emailVerified;
return (T) this;
}
/**
* Sets the {@code externalId} property of this builder.
*
* @param externalId the value for {@link CustomerDraft#getExternalId()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T externalId(@Nullable final String externalId) {
this.externalId = externalId;
return (T) this;
}
/**
* Sets the {@code firstName} property of this builder.
*
* @param firstName the value for {@link CustomerDraft#getFirstName()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T firstName(@Nullable final String firstName) {
this.firstName = firstName;
return (T) this;
}
/**
* Sets the {@code key} property of this builder.
*
* @param key the value for {@link CustomerDraft#getKey()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T key(@Nullable final String key) {
this.key = key;
return (T) this;
}
/**
* Sets the {@code lastName} property of this builder.
*
* @param lastName the value for {@link CustomerDraft#getLastName()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T lastName(@Nullable final String lastName) {
this.lastName = lastName;
return (T) this;
}
/**
* Sets the {@code locale} property of this builder.
*
* @param locale the value for {@link CustomerDraft#getLocale()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T locale(@Nullable final Locale locale) {
this.locale = locale;
return (T) this;
}
/**
* Sets the {@code middleName} property of this builder.
*
* @param middleName the value for {@link CustomerDraft#getMiddleName()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T middleName(@Nullable final String middleName) {
this.middleName = middleName;
return (T) this;
}
/**
* Sets the {@code password} property of this builder.
*
* @param password the value for {@link CustomerDraft#getPassword()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T password(final String password) {
this.password = password;
return (T) this;
}
/**
* Sets the {@code salutation} property of this builder.
*
* @param salutation the value for {@link CustomerDraft#getSalutation()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T salutation(@Nullable final String salutation) {
this.salutation = salutation;
return (T) this;
}
/**
* Sets the {@code shippingAddresses} property of this builder.
*
* @param shippingAddresses the value for {@link CustomerDraft#getShippingAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T shippingAddresses(@Nullable final List shippingAddresses) {
this.shippingAddresses = shippingAddresses;
return (T) this;
}
/**
* Sets the {@code stores} property of this builder.
*
* @param stores the value for {@link CustomerDraft#getStores()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T stores(@Nullable final List> stores) {
this.stores = stores;
return (T) this;
}
/**
* Sets the {@code title} property of this builder.
*
* @param title the value for {@link CustomerDraft#getTitle()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T title(@Nullable final String title) {
this.title = title;
return (T) this;
}
/**
* Sets the {@code vatId} property of this builder.
*
* @param vatId the value for {@link CustomerDraft#getVatId()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T vatId(@Nullable final String vatId) {
this.vatId = vatId;
return (T) this;
}
@Nullable
public List getAddresses() {
return addresses;
}
@Nullable
public ResourceIdentifier getAnonymousCart() {
return anonymousCart;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public String getAnonymousCartId() {
return anonymousCartId;
}
@Nullable
public String getAnonymousId() {
return anonymousId;
}
@Nullable
public AuthenticationMode getAuthenticationMode() {
return authenticationMode;
}
@Nullable
public List getBillingAddresses() {
return billingAddresses;
}
@Nullable
public String getCompanyName() {
return companyName;
}
@Nullable
public CustomFieldsDraft getCustom() {
return custom;
}
@Nullable
public ResourceIdentifier getCustomerGroup() {
return customerGroup;
}
@Nullable
public String getCustomerNumber() {
return customerNumber;
}
@Nullable
public LocalDate getDateOfBirth() {
return dateOfBirth;
}
@Nullable
public Integer getDefaultBillingAddress() {
return defaultBillingAddress;
}
@Nullable
public Integer getDefaultShippingAddress() {
return defaultShippingAddress;
}
public String getEmail() {
return email;
}
@JsonProperty("isEmailVerified")
public Boolean isEmailVerified() {
return emailVerified;
}
@Nullable
public String getExternalId() {
return externalId;
}
@Nullable
public String getFirstName() {
return firstName;
}
@Nullable
public String getKey() {
return key;
}
@Nullable
public String getLastName() {
return lastName;
}
@Nullable
public Locale getLocale() {
return locale;
}
@Nullable
public String getMiddleName() {
return middleName;
}
public String getPassword() {
return password;
}
@Nullable
public String getSalutation() {
return salutation;
}
@Nullable
public List getShippingAddresses() {
return shippingAddresses;
}
@Nullable
public List> getStores() {
return stores;
}
@Nullable
public String getTitle() {
return title;
}
@Nullable
public String getVatId() {
return vatId;
}
/**
* Concatenate {@code addresses} parameter to the {@code addresses} list property of this builder.
*
* @param addresses the value for {@link CustomerDraft#getAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusAddresses(final List addresses) {
this.addresses = SphereInternalUtils.listOf(Optional.ofNullable(this.addresses).orElseGet(ArrayList::new), addresses);
return (T) this;
}
/**
* Concatenate {@code billingAddresses} parameter to the {@code billingAddresses} list property of this builder.
*
* @param billingAddresses the value for {@link CustomerDraft#getBillingAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusBillingAddresses(final List billingAddresses) {
this.billingAddresses = SphereInternalUtils.listOf(Optional.ofNullable(this.billingAddresses).orElseGet(ArrayList::new), billingAddresses);
return (T) this;
}
/**
* Concatenate {@code shippingAddresses} parameter to the {@code shippingAddresses} list property of this builder.
*
* @param shippingAddresses the value for {@link CustomerDraft#getShippingAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusShippingAddresses(final List shippingAddresses) {
this.shippingAddresses = SphereInternalUtils.listOf(Optional.ofNullable(this.shippingAddresses).orElseGet(ArrayList::new), shippingAddresses);
return (T) this;
}
/**
* Concatenate {@code stores} parameter to the {@code stores} list property of this builder.
*
* @param stores the value for {@link CustomerDraft#getStores()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusStores(final List> stores) {
this.stores = SphereInternalUtils.listOf(Optional.ofNullable(this.stores).orElseGet(ArrayList::new), stores);
return (T) this;
}
/**
* Adds {@code addresses} parameter to the {@code addresses} list property of this builder.
*
* @param addresses the value of the element to add to {@link CustomerDraft#getAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusAddresses(final Address addresses) {
this.addresses = SphereInternalUtils.listOf(Optional.ofNullable(this.addresses).orElseGet(ArrayList::new), Collections.singletonList(addresses));
return (T) this;
}
/**
* Adds {@code billingAddresses} parameter to the {@code billingAddresses} list property of this builder.
*
* @param billingAddresses the value of the element to add to {@link CustomerDraft#getBillingAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusBillingAddresses(final Integer billingAddresses) {
this.billingAddresses = SphereInternalUtils.listOf(Optional.ofNullable(this.billingAddresses).orElseGet(ArrayList::new), Collections.singletonList(billingAddresses));
return (T) this;
}
/**
* Adds {@code shippingAddresses} parameter to the {@code shippingAddresses} list property of this builder.
*
* @param shippingAddresses the value of the element to add to {@link CustomerDraft#getShippingAddresses()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusShippingAddresses(final Integer shippingAddresses) {
this.shippingAddresses = SphereInternalUtils.listOf(Optional.ofNullable(this.shippingAddresses).orElseGet(ArrayList::new), Collections.singletonList(shippingAddresses));
return (T) this;
}
/**
* Adds {@code stores} parameter to the {@code stores} list property of this builder.
*
* @param stores the value of the element to add to {@link CustomerDraft#getStores()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusStores(final ResourceIdentifier stores) {
this.stores = SphereInternalUtils.listOf(Optional.ofNullable(this.stores).orElseGet(ArrayList::new), Collections.singletonList(stores));
return (T) this;
}
/**
* Creates a new instance of {@code CustomerDraftDsl} with the values of this builder.
*
* @return the instance
*/
public CustomerDraftDsl build() {
return new CustomerDraftDsl(addresses, anonymousCart, anonymousCartId, anonymousId, authenticationMode, billingAddresses, companyName, custom, customerGroup, customerNumber, dateOfBirth, defaultBillingAddress, defaultShippingAddress, email, emailVerified, externalId, firstName, key, lastName, locale, middleName, password, salutation, shippingAddresses, stores, title, vatId);
}
/**
* Creates a new object initialized with the given values.
*
* @param email initial value for the {@link CustomerDraft#getEmail()} property
* @param password initial value for the {@link CustomerDraft#getPassword()} property
* @return new object initialized with the given values
*/
public static CustomerDraftBuilder of(final String email, final String password) {
return new CustomerDraftBuilder(null, null, null, null, null, null, null, null, null, null, null, null, null, email, null, null, null, null, null, null, null, password, null, null, null, null, null);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static CustomerDraftBuilder of(final CustomerDraft template) {
return new CustomerDraftBuilder(template.getAddresses(), template.getAnonymousCart(), template.getAnonymousCartId(), template.getAnonymousId(), template.getAuthenticationMode(), template.getBillingAddresses(), template.getCompanyName(), template.getCustom(), template.getCustomerGroup(), template.getCustomerNumber(), template.getDateOfBirth(), template.getDefaultBillingAddress(), template.getDefaultShippingAddress(), template.getEmail(), template.isEmailVerified(), template.getExternalId(), template.getFirstName(), template.getKey(), template.getLastName(), template.getLocale(), template.getMiddleName(), template.getPassword(), template.getSalutation(), template.getShippingAddresses(), template.getStores(), template.getTitle(), template.getVatId());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy