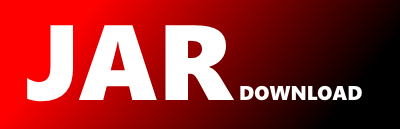
io.sphere.sdk.orderedits.OrderEditDraftDsl Maven / Gradle / Ivy
package io.sphere.sdk.orderedits;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.sphere.sdk.commands.StagedUpdateAction;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.models.Referenceable;
import io.sphere.sdk.orders.Order;
import io.sphere.sdk.types.CustomFieldsDraft;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
import javax.annotation.Nullable;
/**
* Dsl class for {@link OrderEditDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.ResourceDraftValueGenerator",
comments = "Generated from: io.sphere.sdk.orderedits.OrderEditDraft"
)
public final class OrderEditDraftDsl extends Base implements OrderEditDraft {
@Nullable
private String comment;
@Nullable
private CustomFieldsDraft custom;
@Nullable
private Boolean dryRun;
@Nullable
private String key;
private Reference resource;
private List> stagedActions;
@JsonCreator
OrderEditDraftDsl(@Nullable final String comment, @Nullable final CustomFieldsDraft custom,
@Nullable @JsonProperty("dryRun") final Boolean dryRun, @Nullable final String key,
final Reference resource, final List> stagedActions) {
this.comment = comment;
this.custom = custom;
this.dryRun = dryRun;
this.key = key;
this.resource = resource;
this.stagedActions = stagedActions;
}
@Nullable
public String getComment() {
return comment;
}
@Nullable
public CustomFieldsDraft getCustom() {
return custom;
}
@Nullable
@JsonProperty("dryRun")
public Boolean isDryRun() {
return dryRun;
}
@Nullable
public String getKey() {
return key;
}
public Reference getResource() {
return resource;
}
public List> getStagedActions() {
return stagedActions;
}
/**
* Creates a new builder with the values of this object.
*
* @return new builder
*/
public OrderEditDraftBuilder newBuilder() {
return new OrderEditDraftBuilder(comment, custom, dryRun, key, resource, stagedActions);
}
public OrderEditDraftDsl withComment(@Nullable final String comment) {
return newBuilder().comment(comment).build();
}
public OrderEditDraftDsl withCustom(@Nullable final CustomFieldsDraft custom) {
return newBuilder().custom(custom).build();
}
public OrderEditDraftDsl withDryRun(@Nullable final Boolean dryRun) {
return newBuilder().dryRun(dryRun).build();
}
public OrderEditDraftDsl withKey(@Nullable final String key) {
return newBuilder().key(key).build();
}
public OrderEditDraftDsl withResource(final Referenceable resource) {
return newBuilder().resource(Optional.ofNullable(resource).map(Referenceable::toReference).orElse(null)).build();
}
public OrderEditDraftDsl withStagedActions(
final List> stagedActions) {
return newBuilder().stagedActions(stagedActions).build();
}
/**
* Creates a new object initialized with the given values.
*
* @param key initial value for the {@link OrderEditDraft#getKey()} property
* @param resource initial value for the {@link OrderEditDraft#getResource()} property
* @param stagedActions initial value for the {@link OrderEditDraft#getStagedActions()} property
* @param custom initial value for the {@link OrderEditDraft#getCustom()} property
* @param comment initial value for the {@link OrderEditDraft#getComment()} property
* @param dryRun initial value for the {@link OrderEditDraft#isDryRun()} property
* @return new object initialized with the given values
*/
public static OrderEditDraftDsl of(@Nullable final String key, final Reference resource,
final List> stagedActions,
@Nullable final CustomFieldsDraft custom, @Nullable final String comment,
@Nullable final Boolean dryRun) {
return new OrderEditDraftDsl(comment, custom, dryRun, key, resource, stagedActions);
}
/**
* Creates a new object initialized with the given values.
*
* @param resource initial value for the {@link OrderEditDraft#getResource()} property
* @param stagedActions initial value for the {@link OrderEditDraft#getStagedActions()} property
* @return new object initialized with the given values
*/
public static OrderEditDraftDsl of(final Reference resource,
final List> stagedActions) {
return new OrderEditDraftDsl(null, null, null, null, resource, stagedActions);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static OrderEditDraftDsl of(final OrderEditDraft template) {
return new OrderEditDraftDsl(template.getComment(), template.getCustom(), template.isDryRun(), template.getKey(), template.getResource(), template.getStagedActions());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy