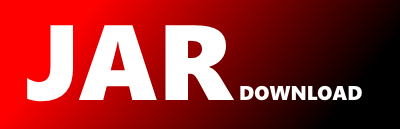
io.sphere.sdk.orders.ParcelDraftBuilder Maven / Gradle / Ivy
package io.sphere.sdk.orders;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.types.CustomFieldsDraft;
import io.sphere.sdk.utils.SphereInternalUtils;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
import javax.annotation.Nullable;
/**
* Builder for {@link ParcelDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.orders.ParcelDraft"
)
public final class ParcelDraftBuilder extends Base implements Builder {
@Nullable
private CustomFieldsDraft custom;
@Nullable
private List items;
@Nullable
private ParcelMeasurements measurements;
@Nullable
private TrackingData trackingData;
ParcelDraftBuilder() {
}
ParcelDraftBuilder(@Nullable final CustomFieldsDraft custom,
@Nullable final List items, @Nullable final ParcelMeasurements measurements,
@Nullable final TrackingData trackingData) {
this.custom = custom;
this.items = items;
this.measurements = measurements;
this.trackingData = trackingData;
}
/**
* Sets the {@code custom} property of this builder.
*
* @param custom the value for {@link ParcelDraft#getCustom()}
* @return this builder
*/
public ParcelDraftBuilder custom(@Nullable final CustomFieldsDraft custom) {
this.custom = custom;
return this;
}
/**
* Sets the {@code items} property of this builder.
*
* @param items the value for {@link ParcelDraft#getItems()}
* @return this builder
*/
public ParcelDraftBuilder items(@Nullable final List items) {
this.items = items;
return this;
}
/**
* Sets the {@code measurements} property of this builder.
*
* @param measurements the value for {@link ParcelDraft#getMeasurements()}
* @return this builder
*/
public ParcelDraftBuilder measurements(@Nullable final ParcelMeasurements measurements) {
this.measurements = measurements;
return this;
}
/**
* Sets the {@code trackingData} property of this builder.
*
* @param trackingData the value for {@link ParcelDraft#getTrackingData()}
* @return this builder
*/
public ParcelDraftBuilder trackingData(@Nullable final TrackingData trackingData) {
this.trackingData = trackingData;
return this;
}
@Nullable
public CustomFieldsDraft getCustom() {
return custom;
}
@Nullable
public List getItems() {
return items;
}
@Nullable
public ParcelMeasurements getMeasurements() {
return measurements;
}
@Nullable
public TrackingData getTrackingData() {
return trackingData;
}
/**
* Concatenate {@code items} parameter to the {@code items} list property of this builder.
*
* @param items the value for {@link ParcelDraft#getItems()}
* @return this builder
*/
public ParcelDraftBuilder plusItems(final List items) {
this.items = SphereInternalUtils.listOf(Optional.ofNullable(this.items).orElseGet(ArrayList::new), items);
return this;
}
/**
* Adds {@code items} parameter to the {@code items} list property of this builder.
*
* @param items the value of the element to add to {@link ParcelDraft#getItems()}
* @return this builder
*/
public ParcelDraftBuilder plusItems(final DeliveryItem items) {
this.items = SphereInternalUtils.listOf(Optional.ofNullable(this.items).orElseGet(ArrayList::new), Collections.singletonList(items));
return this;
}
/**
* Creates a new instance of {@code ParcelDraftDsl} with the values of this builder.
*
* @return the instance
*/
public ParcelDraftDsl build() {
return new ParcelDraftDsl(custom, items, measurements, trackingData);
}
/**
* Creates a new object initialized with the given values.
*
* @return new object initialized with the given values
*/
public static ParcelDraftBuilder of() {
return new ParcelDraftBuilder(null, null, null, null);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static ParcelDraftBuilder of(final ParcelDraft template) {
return new ParcelDraftBuilder(template.getCustom(), template.getItems(), template.getMeasurements(), template.getTrackingData());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy