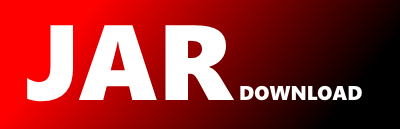
io.sphere.sdk.payments.PaymentDraftDsl Maven / Gradle / Ivy
package io.sphere.sdk.payments;
import com.fasterxml.jackson.annotation.JsonCreator;
import io.sphere.sdk.customers.Customer;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.models.Referenceable;
import io.sphere.sdk.types.CustomFieldsDraft;
import java.lang.Deprecated;
import java.lang.String;
import java.time.ZonedDateTime;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.money.MonetaryAmount;
/**
* Dsl class for {@link PaymentDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.ResourceDraftValueGenerator",
comments = "Generated from: io.sphere.sdk.payments.PaymentDraft"
)
public final class PaymentDraftDsl extends Base implements PaymentDraft {
@Nullable
private MonetaryAmount amountAuthorized;
@Nullable
private MonetaryAmount amountPaid;
private MonetaryAmount amountPlanned;
@Nullable
private MonetaryAmount amountRefunded;
@Nullable
private String anonymousId;
@Nullable
private ZonedDateTime authorizedUntil;
@Nullable
private CustomFieldsDraft custom;
@Nullable
private Reference customer;
@Nullable
private String externalId;
@Nullable
private String interfaceId;
@Nullable
private List interfaceInteractions;
@Nullable
private String key;
@Nullable
private PaymentMethodInfo paymentMethodInfo;
@Nullable
private PaymentStatus paymentStatus;
@Nullable
private List transactions;
@JsonCreator
PaymentDraftDsl(@Nullable final MonetaryAmount amountAuthorized,
@Nullable final MonetaryAmount amountPaid, final MonetaryAmount amountPlanned,
@Nullable final MonetaryAmount amountRefunded, @Nullable final String anonymousId,
@Nullable final ZonedDateTime authorizedUntil, @Nullable final CustomFieldsDraft custom,
@Nullable final Reference customer, @Nullable final String externalId,
@Nullable final String interfaceId,
@Nullable final List interfaceInteractions, @Nullable final String key,
@Nullable final PaymentMethodInfo paymentMethodInfo,
@Nullable final PaymentStatus paymentStatus,
@Nullable final List transactions) {
this.amountAuthorized = amountAuthorized;
this.amountPaid = amountPaid;
this.amountPlanned = amountPlanned;
this.amountRefunded = amountRefunded;
this.anonymousId = anonymousId;
this.authorizedUntil = authorizedUntil;
this.custom = custom;
this.customer = customer;
this.externalId = externalId;
this.interfaceId = interfaceId;
this.interfaceInteractions = interfaceInteractions;
this.key = key;
this.paymentMethodInfo = paymentMethodInfo;
this.paymentStatus = paymentStatus;
this.transactions = transactions;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public MonetaryAmount getAmountAuthorized() {
return amountAuthorized;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public MonetaryAmount getAmountPaid() {
return amountPaid;
}
public MonetaryAmount getAmountPlanned() {
return amountPlanned;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public MonetaryAmount getAmountRefunded() {
return amountRefunded;
}
@Nullable
public String getAnonymousId() {
return anonymousId;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public ZonedDateTime getAuthorizedUntil() {
return authorizedUntil;
}
@Nullable
public CustomFieldsDraft getCustom() {
return custom;
}
@Nullable
public Reference getCustomer() {
return customer;
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Nullable
@Deprecated
public String getExternalId() {
return externalId;
}
@Nullable
public String getInterfaceId() {
return interfaceId;
}
@Nullable
public List getInterfaceInteractions() {
return interfaceInteractions;
}
@Nullable
public String getKey() {
return key;
}
@Nullable
public PaymentMethodInfo getPaymentMethodInfo() {
return paymentMethodInfo;
}
@Nullable
public PaymentStatus getPaymentStatus() {
return paymentStatus;
}
@Nullable
public List getTransactions() {
return transactions;
}
/**
* Creates a new builder with the values of this object.
*
* @return new builder
*/
public PaymentDraftBuilder newBuilder() {
return new PaymentDraftBuilder(amountAuthorized, amountPaid, amountPlanned, amountRefunded, anonymousId, authorizedUntil, custom, customer, externalId, interfaceId, interfaceInteractions, key, paymentMethodInfo, paymentStatus, transactions);
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftDsl withAmountAuthorized(@Nullable final MonetaryAmount amountAuthorized) {
return newBuilder().amountAuthorized(amountAuthorized).build();
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftDsl withAmountPaid(@Nullable final MonetaryAmount amountPaid) {
return newBuilder().amountPaid(amountPaid).build();
}
public PaymentDraftDsl withAmountPlanned(final MonetaryAmount amountPlanned) {
return newBuilder().amountPlanned(amountPlanned).build();
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftDsl withAmountRefunded(@Nullable final MonetaryAmount amountRefunded) {
return newBuilder().amountRefunded(amountRefunded).build();
}
public PaymentDraftDsl withAnonymousId(@Nullable final String anonymousId) {
return newBuilder().anonymousId(anonymousId).build();
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftDsl withAuthorizedUntil(@Nullable final ZonedDateTime authorizedUntil) {
return newBuilder().authorizedUntil(authorizedUntil).build();
}
public PaymentDraftDsl withCustom(@Nullable final CustomFieldsDraft custom) {
return newBuilder().custom(custom).build();
}
public PaymentDraftDsl withCustomer(@Nullable final Referenceable customer) {
return newBuilder().customer(Optional.ofNullable(customer).map(Referenceable::toReference).orElse(null)).build();
}
/**
*
* @deprecated This method will be removed with the next major SDK update.
*/
@Deprecated
public PaymentDraftDsl withExternalId(@Nullable final String externalId) {
return newBuilder().externalId(externalId).build();
}
public PaymentDraftDsl withInterfaceId(@Nullable final String interfaceId) {
return newBuilder().interfaceId(interfaceId).build();
}
public PaymentDraftDsl withInterfaceInteractions(
@Nullable final List interfaceInteractions) {
return newBuilder().interfaceInteractions(interfaceInteractions).build();
}
public PaymentDraftDsl withKey(@Nullable final String key) {
return newBuilder().key(key).build();
}
public PaymentDraftDsl withPaymentMethodInfo(
@Nullable final PaymentMethodInfo paymentMethodInfo) {
return newBuilder().paymentMethodInfo(paymentMethodInfo).build();
}
public PaymentDraftDsl withPaymentStatus(@Nullable final PaymentStatus paymentStatus) {
return newBuilder().paymentStatus(paymentStatus).build();
}
public PaymentDraftDsl withTransactions(@Nullable final List transactions) {
return newBuilder().transactions(transactions).build();
}
/**
* Creates a new object initialized with the given values.
*
* @param amountPlanned initial value for the {@link PaymentDraft#getAmountPlanned()} property
* @return new object initialized with the given values
*/
public static PaymentDraftDsl of(final MonetaryAmount amountPlanned) {
return new PaymentDraftDsl(null, null, amountPlanned, null, null, null, null, null, null, null, null, null, null, null, null);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static PaymentDraftDsl of(final PaymentDraft template) {
return new PaymentDraftDsl(template.getAmountAuthorized(), template.getAmountPaid(), template.getAmountPlanned(), template.getAmountRefunded(), template.getAnonymousId(), template.getAuthorizedUntil(), template.getCustom(), template.getCustomer(), template.getExternalId(), template.getInterfaceId(), template.getInterfaceInteractions(), template.getKey(), template.getPaymentMethodInfo(), template.getPaymentStatus(), template.getTransactions());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy