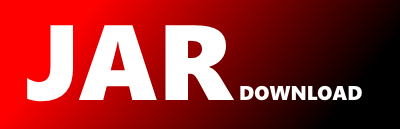
io.sphere.sdk.payments.TransactionDraftBuilder Maven / Gradle / Ivy
package io.sphere.sdk.payments;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.types.CustomFields;
import java.lang.String;
import java.time.ZonedDateTime;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.money.MonetaryAmount;
/**
* Builder for {@link TransactionDraft}.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.payments.TransactionDraft"
)
public final class TransactionDraftBuilder extends Base implements Builder {
private MonetaryAmount amount;
@Nullable
private CustomFields custom;
@Nullable
private String interactionId;
@Nullable
private TransactionState state;
@Nullable
private ZonedDateTime timestamp;
private TransactionType type;
TransactionDraftBuilder() {
}
TransactionDraftBuilder(final MonetaryAmount amount, @Nullable final CustomFields custom,
@Nullable final String interactionId, @Nullable final TransactionState state,
@Nullable final ZonedDateTime timestamp, final TransactionType type) {
this.amount = amount;
this.custom = custom;
this.interactionId = interactionId;
this.state = state;
this.timestamp = timestamp;
this.type = type;
}
/**
* Sets the {@code amount} property of this builder.
*
* @param amount the value for {@link TransactionDraft#getAmount()}
* @return this builder
*/
public TransactionDraftBuilder amount(final MonetaryAmount amount) {
this.amount = amount;
return this;
}
/**
* Sets the {@code custom} property of this builder.
*
* @param custom the value for {@link TransactionDraft#getCustom()}
* @return this builder
*/
public TransactionDraftBuilder custom(@Nullable final CustomFields custom) {
this.custom = custom;
return this;
}
/**
* Sets the {@code interactionId} property of this builder.
*
* @param interactionId the value for {@link TransactionDraft#getInteractionId()}
* @return this builder
*/
public TransactionDraftBuilder interactionId(@Nullable final String interactionId) {
this.interactionId = interactionId;
return this;
}
/**
* Sets the {@code state} property of this builder.
*
* @param state the value for {@link TransactionDraft#getState()}
* @return this builder
*/
public TransactionDraftBuilder state(@Nullable final TransactionState state) {
this.state = state;
return this;
}
/**
* Sets the {@code timestamp} property of this builder.
*
* @param timestamp the value for {@link TransactionDraft#getTimestamp()}
* @return this builder
*/
public TransactionDraftBuilder timestamp(@Nullable final ZonedDateTime timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Sets the {@code type} property of this builder.
*
* @param type the value for {@link TransactionDraft#getType()}
* @return this builder
*/
public TransactionDraftBuilder type(final TransactionType type) {
this.type = type;
return this;
}
public MonetaryAmount getAmount() {
return amount;
}
@Nullable
public CustomFields getCustom() {
return custom;
}
@Nullable
public String getInteractionId() {
return interactionId;
}
@Nullable
public TransactionState getState() {
return state;
}
@Nullable
public ZonedDateTime getTimestamp() {
return timestamp;
}
public TransactionType getType() {
return type;
}
/**
* Creates a new instance of {@code TransactionDraftDsl} with the values of this builder.
*
* @return the instance
*/
public TransactionDraftDsl build() {
return new TransactionDraftDsl(amount, custom, interactionId, state, timestamp, type);
}
/**
* Creates a new object initialized with the given values.
*
* @param type initial value for the {@link TransactionDraft#getType()} property
* @param amount initial value for the {@link TransactionDraft#getAmount()} property
* @return new object initialized with the given values
*/
public static TransactionDraftBuilder of(final TransactionType type,
final MonetaryAmount amount) {
return new TransactionDraftBuilder(amount, null, null, null, null, type);
}
/**
* Creates a new object initialized with the given values.
*
* @param type initial value for the {@link TransactionDraft#getType()} property
* @param amount initial value for the {@link TransactionDraft#getAmount()} property
* @param timestamp initial value for the {@link TransactionDraft#getTimestamp()} property
* @return new object initialized with the given values
*/
public static TransactionDraftBuilder of(final TransactionType type, final MonetaryAmount amount,
@Nullable final ZonedDateTime timestamp) {
return new TransactionDraftBuilder(amount, null, null, null, timestamp, type);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static TransactionDraftBuilder of(final TransactionDraft template) {
return new TransactionDraftBuilder(template.getAmount(), template.getCustom(), template.getInteractionId(), template.getState(), template.getTimestamp(), template.getType());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy