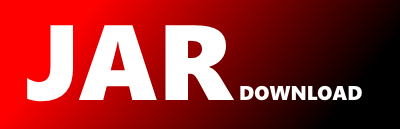
io.sphere.sdk.states.StateDraftBuilderBase Maven / Gradle / Ivy
package io.sphere.sdk.states;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.models.LocalizedString;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.utils.SphereInternalUtils;
import java.lang.Boolean;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.HashSet;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Generated;
import javax.annotation.Nullable;
/**
* Abstract base builder for {@link StateDraft} which needs to be extended to add additional methods.
* Subclasses have to provide the same non-default constructor as this class.
*/
@Generated(
value = "io.sphere.sdk.annotations.processors.generators.DraftBuilderGenerator",
comments = "Generated from: io.sphere.sdk.states.StateDraft"
)
abstract class StateDraftBuilderBase extends Base implements Builder {
@Nullable
LocalizedString description;
@Nullable
Boolean initial;
String key;
@Nullable
LocalizedString name;
@Nullable
Set roles;
@Nullable
Set> transitions;
StateType type;
protected StateDraftBuilderBase() {
}
protected StateDraftBuilderBase(@Nullable final LocalizedString description,
@Nullable final Boolean initial, final String key, @Nullable final LocalizedString name,
@Nullable final Set roles, @Nullable final Set> transitions,
final StateType type) {
this.description = description;
this.initial = initial;
this.key = key;
this.name = name;
this.roles = roles;
this.transitions = transitions;
this.type = type;
}
/**
* Sets the {@code description} property of this builder.
*
* @param description the value for {@link StateDraft#getDescription()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T description(@Nullable final LocalizedString description) {
this.description = description;
return (T) this;
}
/**
* Sets the {@code initial} property of this builder.
*
* @param initial the value for {@link StateDraft#isInitial()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T initial(@Nullable final Boolean initial) {
this.initial = initial;
return (T) this;
}
/**
* Sets the {@code initial} property of this builder.
*
* @param initial the value for {@link StateDraft#isInitial()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T isInitial(@Nullable final Boolean initial) {
this.initial = initial;
return (T) this;
}
/**
* Sets the {@code key} property of this builder.
*
* @param key the value for {@link StateDraft#getKey()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T key(final String key) {
this.key = key;
return (T) this;
}
/**
* Sets the {@code name} property of this builder.
*
* @param name the value for {@link StateDraft#getName()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T name(@Nullable final LocalizedString name) {
this.name = name;
return (T) this;
}
/**
* Sets the {@code roles} property of this builder.
*
* @param roles the value for {@link StateDraft#getRoles()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T roles(@Nullable final Set roles) {
this.roles = roles;
return (T) this;
}
/**
* Sets the {@code transitions} property of this builder.
*
* @param transitions the value for {@link StateDraft#getTransitions()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T transitions(@Nullable final Set> transitions) {
this.transitions = transitions;
return (T) this;
}
/**
* Sets the {@code type} property of this builder.
*
* @param type the value for {@link StateDraft#getType()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T type(final StateType type) {
this.type = type;
return (T) this;
}
@Nullable
public LocalizedString getDescription() {
return description;
}
@Nullable
@JsonProperty("initial")
public Boolean isInitial() {
return initial;
}
public String getKey() {
return key;
}
@Nullable
public LocalizedString getName() {
return name;
}
@Nullable
public Set getRoles() {
return roles;
}
@Nullable
public Set> getTransitions() {
return transitions;
}
public StateType getType() {
return type;
}
/**
* Concatenate {@code roles} parameter to the {@code roles} set property of this builder.
*
* @param roles the value for {@link StateDraft#getRoles()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusRoles(final Set roles) {
this.roles = SphereInternalUtils.setOf(Optional.ofNullable(this.roles).orElseGet(HashSet::new), roles);
return (T) this;
}
/**
* Concatenate {@code transitions} parameter to the {@code transitions} set property of this builder.
*
* @param transitions the value for {@link StateDraft#getTransitions()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusTransitions(final Set> transitions) {
this.transitions = SphereInternalUtils.setOf(Optional.ofNullable(this.transitions).orElseGet(HashSet::new), transitions);
return (T) this;
}
/**
* Adds {@code roles} parameter to the {@code roles} set property of this builder.
*
* @param roles the value of the element to add to {@link StateDraft#getRoles()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusRoles(final StateRole roles) {
this.roles = SphereInternalUtils.setOf(Collections.singleton(roles), Optional.ofNullable(this.roles).orElseGet(HashSet::new));
return (T) this;
}
/**
* Adds {@code transitions} parameter to the {@code transitions} set property of this builder.
*
* @param transitions the value of the element to add to {@link StateDraft#getTransitions()}
* @return this builder
*/
@SuppressWarnings("unchecked")
public T plusTransitions(final Reference transitions) {
this.transitions = SphereInternalUtils.setOf(Collections.singleton(transitions), Optional.ofNullable(this.transitions).orElseGet(HashSet::new));
return (T) this;
}
/**
* Creates a new instance of {@code StateDraftDsl} with the values of this builder.
*
* @return the instance
*/
public StateDraftDsl build() {
return new StateDraftDsl(description, initial, key, name, roles, transitions, type);
}
/**
* Creates a new object initialized with the given values.
*
* @param key initial value for the {@link StateDraft#getKey()} property
* @param type initial value for the {@link StateDraft#getType()} property
* @return new object initialized with the given values
*/
public static StateDraftBuilder of(final String key, final StateType type) {
return new StateDraftBuilder(null, null, key, null, null, null, type);
}
/**
* Creates a new object initialized with the fields of the template parameter.
*
* @param template the template
* @return a new object initialized from the template
*/
public static StateDraftBuilder of(final StateDraft template) {
return new StateDraftBuilder(template.getDescription(), template.isInitial(), template.getKey(), template.getName(), template.getRoles(), template.getTransitions(), template.getType());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy