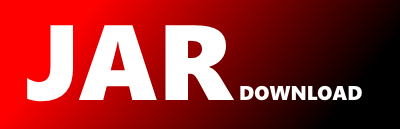
io.sphere.sdk.selection.StoreSelectionQueryParameters Maven / Gradle / Ivy
The newest version!
package io.sphere.sdk.selection;
import io.sphere.sdk.http.NameValuePair;
import io.sphere.sdk.models.Base;
import javax.annotation.Nullable;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import static java.util.stream.Collectors.toList;
public final class StoreSelectionQueryParameters extends Base {
public static final String STORE_PROJECTION = "storeProjection";
private StoreSelectionQueryParameters() {
}
public static List getQueryParametersWithStoreSelection(@Nullable final StoreSelection storeSelection, final List currentParameters) {
final List currentParametersWithoutStoreSelectionParameters = currentParameters.stream()
.filter(pair -> !STORE_PROJECTION.equals(pair.getName()))
.collect(toList());
final List resultingParameters = new LinkedList<>(currentParametersWithoutStoreSelectionParameters);
if (storeSelection != null && storeSelection.getStoreProjection() != null) {
addParamIfNotNull(resultingParameters, STORE_PROJECTION, storeSelection.getStoreProjection().toString());
}
return resultingParameters;
}
@Nullable
public static StoreSelection extractStoreSelectionFromHttpQueryParameters(final List additionalHttpQueryParameters) {
final List storeSelectionCandidates = additionalHttpQueryParameters
.stream()
.filter(pair -> STORE_PROJECTION.equals(pair.getName()))
.collect(Collectors.toList());
final boolean containsStoreSelection = storeSelectionCandidates.stream()
.anyMatch(pair -> STORE_PROJECTION.equals(pair.getName()));
return containsStoreSelection ? extractStoreSelection(storeSelectionCandidates) : null;
}
private static StoreSelection extractStoreSelection(final List storeSelectionCandidates) {
final Map map = NameValuePair.convertToStringMap(storeSelectionCandidates);
return StoreSelectionBuilder.of(map.get(STORE_PROJECTION))
.build();
}
private static void addParamIfNotNull(final List resultingParameters, final String name, final String value) {
if (value != null) {
resultingParameters.add(NameValuePair.of(name, value));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy