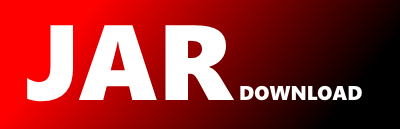
io.sphere.sdk.utils.SphereInternalUtils Maven / Gradle / Ivy
The newest version!
package io.sphere.sdk.utils;
import org.apache.commons.lang3.RandomStringUtils;
import java.text.Normalizer;
import java.util.*;
import java.util.function.Supplier;
import java.util.stream.Stream;
import static java.lang.String.format;
import static java.util.Arrays.asList;
import static java.util.stream.Collectors.joining;
public final class SphereInternalUtils {
private static final int MAX_SLUG_LENGTH = 256;
private SphereInternalUtils() {
}
public static String join(final List list) {
return list.stream().map(i -> i.toString()).collect(joining(", "));
}
public static List immutableCopyOf(final List list) {
return Collections.unmodifiableList(new ArrayList<>(list));
}
@SafeVarargs
@SuppressWarnings("varargs")
public static List asImmutableList(final T ... elements) {
return Collections.unmodifiableList(asList(elements));
}
@SuppressWarnings("varargs")
public static List listOf(final T element, final T[] array) {
final List result = new ArrayList<>(1 + array.length);
result.add(element);
result.addAll(asList(array));
return result;
}
public static List listOf(final List elements, final T element) {
final List result = new ArrayList<>(1 + elements.size());
result.addAll(elements);
result.add(element);
return result;
}
public static List listOf(List extends T> first, List extends T> second) {
final List result = new ArrayList<>(second.size() + first.size());
result.addAll(first);
result.addAll(second);
return result;
}
public static List reverse(final List list) {
final ArrayList result = new ArrayList<>(list);
Collections.reverse(result);
return result;
}
@SafeVarargs
@SuppressWarnings("varargs")
public static Set asSet(final T ... params) {
return new LinkedHashSet<>(asList(params));
}
public static Set setOf(final T element, final Set set) {
final Set result = new HashSet<>(1 + set.size());
result.add(element);
result.addAll(set);
return result;
}
public static Set setOf(final Set first, final Set second) {
final Set result = new HashSet<>(first.size() + second.size());
result.addAll(first);
result.addAll(second);
return result;
}
public static Set setOf(final T element, final T[] array) {
return setOf(element, asSet(array));
}
public static String slugifyUnique(final String s) {
final String postFix = "-" + RandomStringUtils.randomNumeric(8);
final String intermediate = slugify(s);
final String result = intermediate.substring(0, Math.min(intermediate.length(), MAX_SLUG_LENGTH - postFix.length())) + postFix;
return result;
}
public static String slugify(final String s) {
//algorithm used in https://github.com/slugify/slugify/blob/master/core/src/main/java/com/github/slugify/Slugify.java
final String intermediateResult = Normalizer.normalize(s, Normalizer.Form.NFD)
.replaceAll("[^\\p{ASCII}]", "")
.replaceAll("[^-_a-zA-Z0-9]", "-")
.replaceAll("\\s+", "-")
.replaceAll("[-]+", "-")
.replaceAll("^-", "")
.replaceAll("-$", "")
.toLowerCase();
return intermediateResult.substring(0, Math.min(MAX_SLUG_LENGTH, intermediateResult.length()));
}
public static V getOrThrow(final Map map, final K key, Supplier exceptionSupplier) throws E {
if (map.containsKey(key)) {
return map.get(key);
} else {
throw exceptionSupplier.get();
}
}
public static Map immutableCopyOf(final Map map) {
return Collections.unmodifiableMap(copyOf(map));
}
public static Map copyOf(final Map map) {
final Map copy = new LinkedHashMap<>();
copy.putAll(map);
return copy;
}
public static Map mapOf(final K key, final V value) {
final Map result = new LinkedHashMap<>();
result.put(key, value);
return result;
}
public static Map mapOf(final K key1, final V value1, final K key2, final V value2) {
if (key1.equals(key2)) {
throw new IllegalArgumentException(format("Duplicate keys (%s) for map creation.", key1));
}
final Map result = new LinkedHashMap<>();
result.put(key1, value1);
result.put(key2, value2);
return result;
}
public static boolean isEmpty(final Iterable> iterable) {
return !iterable.iterator().hasNext();
}
public static Iterable requireNonEmpty(final Iterable iterable) {
if (isEmpty(iterable)) {
throw new IllegalArgumentException("iterable must not be empty.");
}
return iterable;
}
public static List toList(final Iterable iterable) {
List list = new ArrayList<>();
for (final T item : iterable) {
list.add(item);
}
return list;
}
public static Stream toStream(final Iterable iterable) {
return toList(iterable).stream();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy