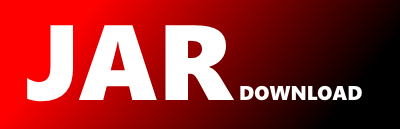
io.vrap.rmf.base.client.http.PolicyBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rmf-java-base Show documentation
Show all versions of rmf-java-base Show documentation
The e-commerce SDK from commercetools for Java
package io.vrap.rmf.base.client.http;
import java.time.Duration;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Function;
import io.vrap.rmf.base.client.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import dev.failsafe.*;
import dev.failsafe.spi.Scheduler;
/**
* PolicyBuilder
*
* The PolicyBuilder allows the combination of different policies for failing requests.
*
* The order of policies matters. For example applying a {@link #withTimeout(Duration) timeout} before
* {@link #withRetry(RetryPolicyBuilder)} retry} will time out across all requests whereas applying a timeout after the retry count
* against every single request even the retried ones.
*
* Retry
*
* Retrying on HTTP status codes
*
* {@include.example io.vrap.rmf.base.client.http.PolicyMiddlewareTest#retryConfigurationStatusCodes()}
*
* Retrying specific exceptions
*
* {@include.example io.vrap.rmf.base.client.http.PolicyMiddlewareTest#retryConfigurationExceptions()}
*
* Timeout
*
* {@include.example io.vrap.rmf.base.client.http.PolicyMiddlewareTest#timeoutConfiguration()}
*
* Bulkhead
*
* Implementation of a Queue to limit the number of concurrent requests handled by the client
*
* {@include.example io.vrap.rmf.base.client.http.PolicyMiddlewareTest#queueConfiguration()}
*/
public class PolicyBuilder {
static final String loggerName = ClientBuilder.COMMERCETOOLS + ".retry";
private static final InternalLogger logger = InternalLogger.getLogger(loggerName);
private static final Logger classLogger = LoggerFactory.getLogger(PolicyMiddleware.class);
private final List>> policies;
private final Scheduler scheduler;
public PolicyBuilder() {
this.policies = new ArrayList<>();
this.scheduler = Scheduler.DEFAULT;
}
public PolicyBuilder(List>> policies) {
this.policies = policies;
this.scheduler = Scheduler.DEFAULT;
}
public PolicyBuilder(Scheduler scheduler, List>> policies) {
this.policies = policies;
this.scheduler = scheduler;
}
public PolicyBuilder withScheduler(ScheduledExecutorService scheduler) {
return new PolicyBuilder(Scheduler.of(scheduler), policies);
}
public PolicyBuilder withScheduler(ExecutorService scheduler) {
return new PolicyBuilder(Scheduler.of(scheduler), policies);
}
public PolicyBuilder withScheduler(Scheduler scheduler) {
return new PolicyBuilder(scheduler, policies);
}
public PolicyBuilder withRetry(RetryPolicyBuilder retryPolicyBuilder) {
return withPolicy(retryPolicyBuilder.build());
}
public PolicyBuilder withRetry(Function fn) {
return withPolicy(fn.apply(RetryPolicyBuilder.of()).build());
}
public PolicyBuilder withRetry(final int maxRetries) {
return withRetry(retry -> retry.maxRetries(maxRetries));
}
public PolicyBuilder withRetry(final int maxRetries, final List statusCodes) {
return withRetry(retry -> retry.maxRetries(maxRetries).statusCodes(statusCodes));
}
public PolicyBuilder withRetry(final int maxRetries, final List statusCodes,
final List> failures) {
return withRetry(retry -> retry.maxRetries(maxRetries).statusCodes(statusCodes).failures(failures));
}
public PolicyBuilder withBulkhead(final int maxConcurrency) {
return withPolicy(bulkhead(maxConcurrency));
}
public PolicyBuilder withBulkhead(final int maxConcurrency, final Duration maxWaitTime) {
return withPolicy(bulkhead(maxConcurrency, maxWaitTime));
}
public PolicyBuilder withBulkhead(final int maxConcurrency, final FailsafeConcurrencyBuilderOptions fn) {
return withPolicy(bulkhead(maxConcurrency, fn));
}
public PolicyBuilder withBulkhead(final int maxConcurrency, final Duration maxWaitTime,
final FailsafeConcurrencyBuilderOptions fn) {
return withPolicy(bulkhead(maxConcurrency, maxWaitTime, fn));
}
public PolicyBuilder withTimeout(final Duration duration) {
return withPolicy(timeout(duration));
}
public PolicyBuilder withTimeout(final Duration duration, final FailsafeTimeoutBuilderOptions fn) {
return withPolicy(timeout(duration, fn));
}
public PolicyBuilder withPolicy(Policy> policy) {
List>> copy = new ArrayList<>(policies);
copy.add(policy);
return new PolicyBuilder(copy);
}
public PolicyMiddleware build() {
return PolicyMiddleware.of(scheduler, policies);
}
public static PolicyBuilder of() {
return new PolicyBuilder();
}
public static Timeout> timeout(final Duration duration) {
return timeout(duration, options -> options);
}
public static Timeout> timeout(final Duration duration,
final FailsafeTimeoutBuilderOptions fn) {
return fn.apply(Timeout.builder(duration)).build();
}
public static Bulkhead> bulkhead(final int maxConcurrency) {
return bulkhead(maxConcurrency, options -> options);
}
public static Bulkhead> bulkhead(final int maxConcurrency, final Duration maxWaitTime) {
return bulkhead(maxConcurrency, maxWaitTime, options -> options);
}
public static Bulkhead> bulkhead(final int maxConcurrency,
final FailsafeConcurrencyBuilderOptions fn) {
return fn.apply(Bulkhead.builder(maxConcurrency)).build();
}
public static Bulkhead> bulkhead(final int maxConcurrency, final Duration maxWaitTime,
final FailsafeConcurrencyBuilderOptions fn) {
return fn.apply(Bulkhead.> builder(maxConcurrency).withMaxWaitTime(maxWaitTime))
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy