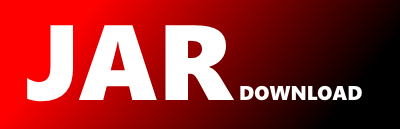
com.commercetools.sync.cartdiscounts.helpers.CartDiscountBatchValidator Maven / Gradle / Ivy
package com.commercetools.sync.cartdiscounts.helpers;
import static java.lang.String.format;
import static org.apache.commons.lang3.StringUtils.isBlank;
import com.commercetools.api.models.cart_discount.CartDiscountDraft;
import com.commercetools.sync.cartdiscounts.CartDiscountSyncOptions;
import com.commercetools.sync.commons.helpers.BaseBatchValidator;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.apache.commons.lang3.tuple.ImmutablePair;
public class CartDiscountBatchValidator
extends BaseBatchValidator<
CartDiscountDraft, CartDiscountSyncOptions, CartDiscountSyncStatistics> {
static final String CART_DISCOUNT_DRAFT_KEY_NOT_SET =
"CartDiscountDraft with name: %s doesn't have a key. "
+ "Please make sure all cart discount drafts have keys.";
static final String CART_DISCOUNT_DRAFT_IS_NULL = "CartDiscountDraft is null.";
public CartDiscountBatchValidator(
@Nonnull final CartDiscountSyncOptions syncOptions,
@Nonnull final CartDiscountSyncStatistics syncStatistics) {
super(syncOptions, syncStatistics);
}
/**
* Given the {@link java.util.List}<{@link CartDiscountDraft}> of drafts this method
* attempts to validate drafts and collect referenced type keys from the draft and return an
* {@link org.apache.commons.lang3.tuple.ImmutablePair}<{@link java.util.Set}<{@link
* CartDiscountDraft}> ,{@link java.util.Set}<{@link String}>> which contains the
* {@link java.util.Set} of valid drafts and referenced type keys.
*
* A valid cart discount draft is one which satisfies the following conditions:
*
*
* - It is not null
*
- It has a key which is not blank (null/empty)
*
*
* @param cartDiscountDrafts the cart discount drafts to validate and collect referenced type
* keys.
* @return {@link org.apache.commons.lang3.tuple.ImmutablePair}<{@link java.util.Set}<{@link
* CartDiscountDraft}>, {@link java.util.Set}<{@link String}>> which contains the
* {@link java.util.Set} of valid drafts and referenced type keys.
*/
@Override
public ImmutablePair, Set> validateAndCollectReferencedKeys(
@Nonnull final List cartDiscountDrafts) {
final Set typeKeys = new HashSet<>();
final Set validDrafts =
cartDiscountDrafts.stream()
.filter(this::isValidCartDiscountDraft)
.peek(
cartDiscountDraft ->
collectReferencedKeyFromCustomFieldsDraft(
cartDiscountDraft.getCustom(), typeKeys::add))
.collect(Collectors.toSet());
return ImmutablePair.of(validDrafts, typeKeys);
}
private boolean isValidCartDiscountDraft(@Nullable final CartDiscountDraft cartDiscountDraft) {
if (cartDiscountDraft == null) {
handleError(CART_DISCOUNT_DRAFT_IS_NULL);
} else if (isBlank(cartDiscountDraft.getKey())) {
handleError(format(CART_DISCOUNT_DRAFT_KEY_NOT_SET, cartDiscountDraft.getName()));
} else {
return true;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy