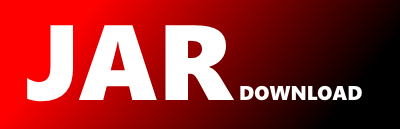
com.commercetools.sync.services.ShoppingListService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commercetools-sync-java Show documentation
Show all versions of commercetools-sync-java Show documentation
Java Library used to import and/or sync (taking care of changes) data into one or more commercetools projects from external sources such as CSV, XML, JSON, etc.. or even from an already existing commercetools project.
The newest version!
package com.commercetools.sync.services;
import com.commercetools.api.models.shopping_list.ShoppingList;
import com.commercetools.api.models.shopping_list.ShoppingListDraft;
import com.commercetools.api.models.shopping_list.ShoppingListUpdateAction;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.concurrent.CompletionStage;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
public interface ShoppingListService {
/**
* Filters out the keys which are already cached and fetches only the not-cached shopping list
* keys from the CTP project defined in an injected {@link
* com.commercetools.api.client.ProjectApiRoot} and stores a mapping for every shopping list to id
* in the cached map of keys -> ids and returns this cached map.
*
* Note: If all the supplied keys are already cached, the cached map is returned right away
* with no request to CTP.
*
* @param shoppingListKeys the shopping list keys to fetch and cache the ids for.
* @return {@link java.util.concurrent.CompletionStage}<{@link java.util.Map}> in which the
* result of it's completion contains a map of all shopping list keys -> ids
*/
@Nonnull
CompletionStage
© 2015 - 2025 Weber Informatics LLC | Privacy Policy