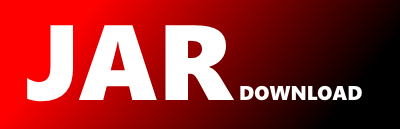
com.commercetools.sync.services.impl.TaxCategoryServiceImpl Maven / Gradle / Ivy
package com.commercetools.sync.services.impl;
import static com.commercetools.sync.commons.utils.SyncUtils.batchElements;
import com.commercetools.api.client.ByProjectKeyTaxCategoriesGet;
import com.commercetools.api.client.ByProjectKeyTaxCategoriesKeyByKeyGet;
import com.commercetools.api.client.ByProjectKeyTaxCategoriesPost;
import com.commercetools.api.models.tax_category.TaxCategory;
import com.commercetools.api.models.tax_category.TaxCategoryDraft;
import com.commercetools.api.models.tax_category.TaxCategoryPagedQueryResponse;
import com.commercetools.api.models.tax_category.TaxCategoryUpdateAction;
import com.commercetools.api.models.tax_category.TaxCategoryUpdateBuilder;
import com.commercetools.api.predicates.query.tax_category.TaxCategoryQueryBuilderDsl;
import com.commercetools.sync.commons.models.GraphQlQueryResource;
import com.commercetools.sync.services.TaxCategoryService;
import com.commercetools.sync.taxcategories.TaxCategorySyncOptions;
import io.vrap.rmf.base.client.ApiHttpResponse;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.function.Function;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
public final class TaxCategoryServiceImpl
extends BaseServiceWithKey<
TaxCategorySyncOptions,
TaxCategory,
TaxCategoryDraft,
ByProjectKeyTaxCategoriesGet,
TaxCategoryPagedQueryResponse,
ByProjectKeyTaxCategoriesKeyByKeyGet,
TaxCategory,
TaxCategoryQueryBuilderDsl,
ByProjectKeyTaxCategoriesPost>
implements TaxCategoryService {
public TaxCategoryServiceImpl(@Nonnull final TaxCategorySyncOptions syncOptions) {
super(syncOptions);
}
@Nonnull
@Override
public CompletionStage
© 2015 - 2025 Weber Informatics LLC | Privacy Policy