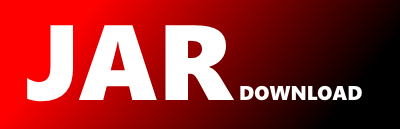
com.composum.ai.backend.base.service.chat.GPTTranslationService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of composum-ai-integration-backend-base Show documentation
Show all versions of composum-ai-integration-backend-base Show documentation
Basic functionality for Composum AI, somewhat platform agnostic
package com.composum.ai.backend.base.service.chat;
import java.util.List;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.composum.ai.backend.base.service.GPTException;
/**
* Building on {@link GPTChatCompletionService} this implements translation.
*/
public interface GPTTranslationService {
/**
* Translate the text from the target to destination language, either Java locale name or language name.
* @param text the text to translate
* @param sourceLanguage the language to translate from - human readable name, or null for autodetect
* @param targetLanguage the language to translate to - human readable name
* @param configuration the configuration to use
*/
@Nullable
String singleTranslation(@Nullable String text, @Nullable String sourceLanguage, @Nullable String targetLanguage, @Nullable GPTConfiguration configuration) throws GPTException;
/**
* Translate the text from the target to destination language, either Java locale name or language name.
*/
void streamingSingleTranslation(@Nonnull String text, @Nonnull String sourceLanguage, @Nonnull String targetLanguage, @Nullable GPTConfiguration configuration, @Nonnull GPTCompletionCallback callback) throws GPTException;
/**
* Translates the texts into the target language. The texts should belong together, like e.g. the texts of a page,
* since the translations might influence each other. (We try to translate them in one request.)
*
* @param texts the texts to translate
* @param targetLanguage the language to translate to - human readable name
* @return the translated texts
*/
@Nonnull
default List fragmentedTranslation(@Nonnull List texts, @Nonnull String targetLanguage, @Nullable GPTConfiguration configuration) throws GPTException {
return fragmentedTranslation(texts, targetLanguage, configuration, null);
}
/**
* Translates the texts into the target language. The texts should belong together, like e.g. the texts of a page,
* since the translations might influence each other. (We try to translate them in one request.)
*
* @param texts the texts to translate
* @param targetLanguage the language to translate to - human readable name
* @param configuration the configuration to use
* @param translationChecks additional checks to verify the translation
* @return the translated texts
*/
@Nonnull
List fragmentedTranslation(@Nonnull List texts, @Nonnull String targetLanguage, @Nullable GPTConfiguration configuration,
@Nullable List translationChecks) throws GPTException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy