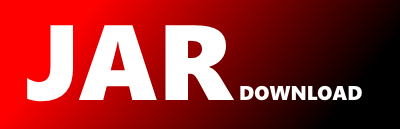
com.composum.sling.core.util.RequestUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of composum-nodes-commons Show documentation
Show all versions of composum-nodes-commons Show documentation
general components and objects to use the Sling API
package com.composum.sling.core.util;
import org.apache.commons.lang3.StringUtils;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.request.RequestParameter;
import org.apache.sling.api.request.RequestParameterMap;
import org.apache.sling.api.resource.ResourceResolver;
import javax.jcr.Session;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* A basic class for all '/bin/{service}/path/to/resource' servlets.
*/
public class RequestUtil extends org.apache.sling.api.request.RequestUtil {
public static Session getSession(SlingHttpServletRequest request) {
ResourceResolver resolver = request.getResourceResolver();
return resolver.adaptTo(Session.class);
}
/**
* Returns the enum value of the requests extension if appropriate otherwise the default value.
*
* @param request the request object with the extension info
* @param defaultValue the default enum value
* @param the enum type derived from the default value
*/
public static T getExtension(SlingHttpServletRequest request, T defaultValue) {
String extension = request.getRequestPathInfo().getExtension();
if (extension != null) {
Class type = defaultValue.getClass();
try {
T value = (T) T.valueOf(type, extension.toLowerCase());
return value;
} catch (IllegalArgumentException iaex) {
// ok, use default
}
}
return defaultValue;
}
/**
* Returns an enum value from selectors if an appropriate selector
* can be found otherwise the default value given.
*
* @param request the request object with the selector info
* @param defaultValue the default enum value
* @param the enum type derived from the default value
*/
public static T getSelector(SlingHttpServletRequest request, T defaultValue) {
String[] selectors = request.getRequestPathInfo().getSelectors();
Class type = defaultValue.getClass();
for (String selector : selectors) {
try {
T value = (T) T.valueOf(type, selector);
return value;
} catch (IllegalArgumentException iaex) {
// ok, try next
}
}
return defaultValue;
}
/**
* Retrieves a key in the selectors and returns 'true' is the key is present.
*
* @param request the request object with the selector info
* @param key the selector key which is checked
*/
public static boolean checkSelector(SlingHttpServletRequest request, String key) {
String[] selectors = request.getRequestPathInfo().getSelectors();
for (String selector : selectors) {
if (selector.equals(key)) {
return true;
}
}
return false;
}
/**
* Retrieves a number in the selectors and returns it if present otherwise the default value.
*
* @param request the request object with the selector info
* @param defaultValue the default number value
*/
public static int getIntSelector(SlingHttpServletRequest request, int defaultValue) {
String[] selectors = request.getRequestPathInfo().getSelectors();
for (String selector : selectors) {
try {
return Integer.parseInt(selector);
} catch (NumberFormatException nfex) {
// ok, try next
}
}
return defaultValue;
}
/**
* Retrieves a number in the selectors and returns it if present otherwise the default value.
*
* @param request the request object with the selector info
* @param groupPattern the regex to extract the value - as group '1'; e.g. 'key([\d]+)'
* @param defaultValue the default number value
*/
public static int getIntSelector(SlingHttpServletRequest request, Pattern groupPattern, int defaultValue) {
String[] selectors = request.getRequestPathInfo().getSelectors();
for (String selector : selectors) {
Matcher matcher = groupPattern.matcher(selector);
if (matcher.matches()) {
try {
return Integer.parseInt(matcher.group(1));
} catch (NumberFormatException nfex) {
// ok, try next
}
}
}
return defaultValue;
}
//
// parameter getters
//
public static String getParameter(RequestParameterMap parameters, String name, String defaultValue) {
RequestParameter parameter = parameters.getValue(name);
String string;
return parameter != null && StringUtils.isNotBlank(string = XSS.filter(parameter.getString()))
? string : defaultValue;
}
public static String getParameter(SlingHttpServletRequest request, String name, String defaultValue) {
String result = XSS.filter(request.getParameter(name));
return StringUtils.isNotBlank(result) ? result : defaultValue;
}
public static Integer getParameter(RequestParameterMap parameters, String name, Integer defaultValue) {
Integer result = defaultValue;
RequestParameter parameter = parameters.getValue(name);
String string;
if (parameter != null && StringUtils.isNotBlank(string = parameter.getString())) {
try {
result = Integer.parseInt(string);
} catch (NumberFormatException nfex) {
// ok, use default value
}
}
return result;
}
public static Integer getParameter(SlingHttpServletRequest request, String name, Integer defaultValue) {
Integer result = defaultValue;
String string = request.getParameter(name);
if (StringUtils.isNotBlank(string)) {
try {
result = Integer.parseInt(string);
} catch (NumberFormatException nfex) {
// ok, use default value
}
}
return result;
}
public static Boolean getParameter(SlingHttpServletRequest request, String name, Boolean defaultValue) {
Boolean result = null;
String string = request.getParameter(name);
if (string != null) {
result = StringUtils.isBlank(string) || name.equals(string) || Boolean.parseBoolean(string);
}
return result != null ? result : defaultValue;
}
public static T getParameter(RequestParameterMap parameters, String name, T defaultValue) {
T result = null;
RequestParameter parameter = parameters.getValue(name);
String string;
if (parameter != null && StringUtils.isNotBlank(string = parameter.getString())) {
try {
result = (T) T.valueOf(defaultValue.getClass(), string);
} catch (IllegalArgumentException iaex) {
// ok, use default value
}
}
return result != null ? result : defaultValue;
}
public static T getParameter(SlingHttpServletRequest request, String name, T defaultValue) {
T result = null;
String string = request.getParameter(name);
if (StringUtils.isNotBlank(string)) {
try {
result = (T) T.valueOf(defaultValue.getClass(), string);
} catch (IllegalArgumentException iaex) {
// ok, use default value
}
}
return result != null ? result : defaultValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy