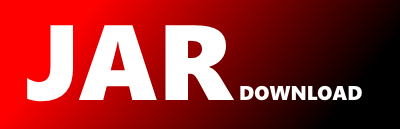
com.composum.sling.core.util.StructuredValueMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of composum-nodes-commons Show documentation
Show all versions of composum-nodes-commons Show documentation
general components and objects to use the Sling API
package com.composum.sling.core.util;
import org.apache.commons.lang3.StringUtils;
import org.apache.sling.api.resource.ValueMap;
import org.apache.sling.api.wrappers.ValueMapDecorator;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
/**
* A ValueMap which supports nested maps and resolves paths to properties of nested maps.
* This is useful if you want to use placeholders for a complex set of values,
* e.g. in a ValueEmbeddingReader.
* You can use either a path (delimiter: '/') or an object (delimiter: '.') notation,
* e.g. ${nested/immediate/prop} or ${nested.immediate.prop}.
* the path notation has precedence over the object notation,
* e.g. ${nested/prop.name} references the 'prop.name' property of the 'nested' map.
*/
public class StructuredValueMap implements ValueMap {
protected final ValueMap base;
public StructuredValueMap(Map base) {
this.base = base instanceof ValueMap ? (ValueMap) base : new ValueMapDecorator(base);
}
@Override
@SuppressWarnings("unchecked")
public T get(@NotNull String name, @NotNull Class type) {
return (T) _get(name, type);
}
@NotNull
@Override
@SuppressWarnings("unchecked")
public T get(@NotNull String name, @NotNull T defaultValue) {
T value = get(name, (Class) defaultValue.getClass());
return value == null ? defaultValue : value;
}
@Override
public int size() {
return _size(this.base);
}
@Override
public boolean isEmpty() {
return size() == 0;
}
@Override
public boolean containsKey(Object key) {
return key != null && _containsKey(key.toString());
}
@Override
public boolean containsValue(Object value) {
return value != null && _containsValue(value);
}
@Override
public Object get(Object key) {
return key != null ? _get(key.toString(), null) : null;
}
/**
* puts a new value at the path given by the name;
* if path starts with '/' the path delimiter is used to build nested maps but ignored in the key
*
* @param key the key (the path) of the value to store
* @param value the value to store
* @return the removed value; maybe 'null'
*/
@Nullable
@Override
public Object put(String key, Object value) {
return key != null ? _put(key, value) : null;
}
@Override
public Object remove(Object key) {
return key != null ? _remove(key.toString()) : null;
}
@Override
public void putAll(@NotNull Map extends String, ?> m) {
for (String key : m.keySet()) {
put(key, m.get(key));
}
}
@Override
public void clear() {
this.base.clear();
}
/**
* @return the stripped set of keys including nested keys as paths and excluding the nested maps itself
*/
@NotNull
@Override
public Set keySet() {
HashSet result = new LinkedHashSet<>();
_keySet(this.base, result, "");
return result;
}
/**
* @return the stripped set of values including nested values and excluding the nested maps itself
*/
@NotNull
@Override
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy