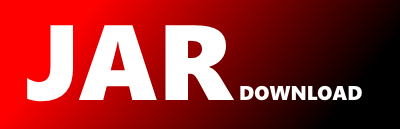
com.comprodls.services.product.ProductClientImpl Maven / Gradle / Ivy
The newest version!
/*************************************************************************
*
* COMPRO CONFIDENTIAL
* __________________
*
* [2015] - [2020] Compro Technologies Private Limited
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Compro Technologies Private Limited. The
* intellectual and technical concepts contained herein are
* proprietary to Compro Technologies Private Limited and may
* be covered by U.S. and Foreign Patents, patents in process,
* and are protected by trade secret or copyright law.
*
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Compro Technologies Pvt. Ltd..
***************************************************************************/
/***********************************************************
* comproDLS SDK Main Module
* This module provides definition of SDK for Javascript
************************************************************/
package com.comprodls.services.product;
import java.io.IOException;
import org.apache.http.HttpException;
import org.json.simple.JSONObject;
import org.json.simple.parser.ParseException;
import com.comprodls.Config;
import com.comprodls.Request;
import com.comprodls.ServiceConnectionException;
import com.comprodls.AbstractClient;
import com.comprodls.services.core.ComproDLS;
import com.comprodls.ProductClient;
public class ProductClientImpl extends AbstractClient implements ProductClient {
private String productId;
public ProductClientImpl(ComproDLS comproDLS, String productId)
{
super(comproDLS);
this.productId = productId;
}
public ProductClientImpl(ComproDLS comproDLS)
{
super(comproDLS);
}
/* (non-Javadoc)
* @see com.comprodls.ProductClient#getProducts(java.lang.String, java.lang.String, java.lang.String)
*/
public String getProducts(String search, String type, String cursor) throws HttpException, IOException, ParseException {
if(search==null || type==null || cursor==null)
throw new ServiceConnectionException("search, type or cursor string is invalid");
String queryParameters = "?search=" + search + "&type=" + type + "&cursor=" + cursor;
return callProductsAPI(queryParameters);
}
/* (non-Javadoc)
* @see com.comprodls.ProductClient#getProducts(java.lang.String, java.lang.String)
*/
public String getProducts(String search, String type) throws HttpException, IOException, ParseException {
if(search==null || type==null)
throw new ServiceConnectionException("search or type string is invalid");
String queryParameters = "?search=" + search + "&type=" + type;
return callProductsAPI(queryParameters);
}
/* (non-Javadoc)
* @see com.comprodls.ProductClient#getProducts(java.lang.String)
*/
public String getProducts(String search) throws HttpException, IOException, ParseException {
if(search==null)
throw new ServiceConnectionException("search string is invalid");
String queryParameters = "?search=" + search;
return callProductsAPI(queryParameters);
}
/* (non-Javadoc)
* @see com.comprodls.ProductClient#getProducts()
*/
public String getProducts() throws HttpException, IOException, ParseException {
return callProductsAPI("");
}
private String callProductsAPI(String queryParameters) throws HttpException, IOException, ParseException
{
JSONObject res;
String host = Config.getProperty(Config.DEFAULT_HOSTS, Config.PRODUCT);
String url = Config.getProperty(Config.PRODUCT_API_URLS, Config.productAPI);
url = Request.constructAPIUrl(url, comproDLS.getOrgId());
url = url + queryParameters;
res = Request.makeHTTPGETRequest(host, url, comproDLS.getToken());
return res.toJSONString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy