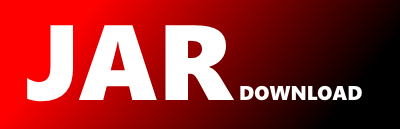
com.confidentify.client.model.EmailResponseRecord Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of confidentify-client-java Show documentation
Show all versions of confidentify-client-java Show documentation
This project provides a Java client for the Conf·ident·ify APIs.
/*
* Confidentify API
* Services that let you build confidence and identify matches in customer data. ## Features overview * Contact data processing services (tagged with `process`) which offer validation and enrichment backed by inference and knowledge on complex data types such as names, email addresses, phone numbers. * Data matching and searching services (tagged with `matching`) that allow you to identify duplicated data or matches against third party contact data list. * Dataset management services (tagged with `dataset`) that allow record storage and retrieval. ## Integrator notes: * Use the `/auth` endpoint to get an access token. Access tokens are temporary, so design the client the be capable of renewing it. * The APIs are rate-limited, so design the client to be capable of retrying with some delay upon HTTP 429 responses.
*
* The version of the OpenAPI document: 1.1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.confidentify.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.confidentify.client.model.ProcessorOutcome;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* EmailResponseRecord
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-04-21T21:21:06.848810800+02:00[Europe/Paris]")
public class EmailResponseRecord {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_EMAIL_FORMATTED = "email_formatted";
@SerializedName(SERIALIZED_NAME_EMAIL_FORMATTED)
private String emailFormatted;
public static final String SERIALIZED_NAME_EMAIL_SIMPLIFIED = "email_simplified";
@SerializedName(SERIALIZED_NAME_EMAIL_SIMPLIFIED)
private String emailSimplified;
public static final String SERIALIZED_NAME_USERNAME = "username";
@SerializedName(SERIALIZED_NAME_USERNAME)
private String username;
public static final String SERIALIZED_NAME_LABEL = "label";
@SerializedName(SERIALIZED_NAME_LABEL)
private String label;
public static final String SERIALIZED_NAME_SUBDOMAIN = "subdomain";
@SerializedName(SERIALIZED_NAME_SUBDOMAIN)
private String subdomain;
public static final String SERIALIZED_NAME_DOMAIN = "domain";
@SerializedName(SERIALIZED_NAME_DOMAIN)
private String domain;
public static final String SERIALIZED_NAME_TLD = "tld";
@SerializedName(SERIALIZED_NAME_TLD)
private String tld;
public static final String SERIALIZED_NAME_EMAIL_SUGGESTED = "email_suggested";
@SerializedName(SERIALIZED_NAME_EMAIL_SUGGESTED)
private String emailSuggested;
public static final String SERIALIZED_NAME_OUTCOME = "outcome";
@SerializedName(SERIALIZED_NAME_OUTCOME)
private ProcessorOutcome outcome;
public EmailResponseRecord id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "By7P02fmNx", value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public EmailResponseRecord emailFormatted(String emailFormatted) {
this.emailFormatted = emailFormatted;
return this;
}
/**
* Get emailFormatted
* @return emailFormatted
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[email protected]", value = "")
public String getEmailFormatted() {
return emailFormatted;
}
public void setEmailFormatted(String emailFormatted) {
this.emailFormatted = emailFormatted;
}
public EmailResponseRecord emailSimplified(String emailSimplified) {
this.emailSimplified = emailSimplified;
return this;
}
/**
* Simplified variant of the email address. This format strips out characters or parts of the email that may be technically irrelevant depending on the email domain's addressing rules. We do not recommend using the simplified email address for sending, but it may be beneficial for certain cases such as identifying duplicates.
* @return emailSimplified
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[email protected]", value = "Simplified variant of the email address. This format strips out characters or parts of the email that may be technically irrelevant depending on the email domain's addressing rules. We do not recommend using the simplified email address for sending, but it may be beneficial for certain cases such as identifying duplicates. ")
public String getEmailSimplified() {
return emailSimplified;
}
public void setEmailSimplified(String emailSimplified) {
this.emailSimplified = emailSimplified;
}
public EmailResponseRecord username(String username) {
this.username = username;
return this;
}
/**
* Get username
* @return username
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "info", value = "")
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public EmailResponseRecord label(String label) {
this.label = label;
return this;
}
/**
* Get label
* @return label
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "sales", value = "")
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public EmailResponseRecord subdomain(String subdomain) {
this.subdomain = subdomain;
return this;
}
/**
* Get subdomain
* @return subdomain
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "mail", value = "")
public String getSubdomain() {
return subdomain;
}
public void setSubdomain(String subdomain) {
this.subdomain = subdomain;
}
public EmailResponseRecord domain(String domain) {
this.domain = domain;
return this;
}
/**
* Get domain
* @return domain
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "domain", value = "")
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public EmailResponseRecord tld(String tld) {
this.tld = tld;
return this;
}
/**
* Get tld
* @return tld
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "com", value = "")
public String getTld() {
return tld;
}
public void setTld(String tld) {
this.tld = tld;
}
public EmailResponseRecord emailSuggested(String emailSuggested) {
this.emailSuggested = emailSuggested;
return this;
}
/**
* When available, a suggested corrected email address.
* @return emailSuggested
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[email protected]", value = "When available, a suggested corrected email address. ")
public String getEmailSuggested() {
return emailSuggested;
}
public void setEmailSuggested(String emailSuggested) {
this.emailSuggested = emailSuggested;
}
public EmailResponseRecord outcome(ProcessorOutcome outcome) {
this.outcome = outcome;
return this;
}
/**
* Get outcome
* @return outcome
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ProcessorOutcome getOutcome() {
return outcome;
}
public void setOutcome(ProcessorOutcome outcome) {
this.outcome = outcome;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailResponseRecord emailResponseRecord = (EmailResponseRecord) o;
return Objects.equals(this.id, emailResponseRecord.id) &&
Objects.equals(this.emailFormatted, emailResponseRecord.emailFormatted) &&
Objects.equals(this.emailSimplified, emailResponseRecord.emailSimplified) &&
Objects.equals(this.username, emailResponseRecord.username) &&
Objects.equals(this.label, emailResponseRecord.label) &&
Objects.equals(this.subdomain, emailResponseRecord.subdomain) &&
Objects.equals(this.domain, emailResponseRecord.domain) &&
Objects.equals(this.tld, emailResponseRecord.tld) &&
Objects.equals(this.emailSuggested, emailResponseRecord.emailSuggested) &&
Objects.equals(this.outcome, emailResponseRecord.outcome);
}
@Override
public int hashCode() {
return Objects.hash(id, emailFormatted, emailSimplified, username, label, subdomain, domain, tld, emailSuggested, outcome);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailResponseRecord {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" emailFormatted: ").append(toIndentedString(emailFormatted)).append("\n");
sb.append(" emailSimplified: ").append(toIndentedString(emailSimplified)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" label: ").append(toIndentedString(label)).append("\n");
sb.append(" subdomain: ").append(toIndentedString(subdomain)).append("\n");
sb.append(" domain: ").append(toIndentedString(domain)).append("\n");
sb.append(" tld: ").append(toIndentedString(tld)).append("\n");
sb.append(" emailSuggested: ").append(toIndentedString(emailSuggested)).append("\n");
sb.append(" outcome: ").append(toIndentedString(outcome)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy