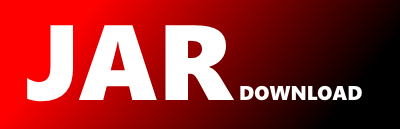
com.confidentify.client.model.PersonNameBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of confidentify-client-java Show documentation
Show all versions of confidentify-client-java Show documentation
This project provides a Java client for the Conf·ident·ify APIs.
/*
* Confidentify API
* Services that let you build confidence and identify matches in customer data. ## Features overview * Contact data processing services (tagged with `process`) which offer validation and enrichment backed by inference and knowledge on complex data types such as names, email addresses, phone numbers. * Data matching and searching services (tagged with `matching`) that allow you to identify duplicated data or matches against third party contact data list. * Dataset management services (tagged with `dataset`) that allow record storage and retrieval. ## Integrator notes: * Use the `/auth` endpoint to get an access token. Access tokens are temporary, so design the client the be capable of renewing it. * The APIs are rate-limited, so design the client to be capable of retrying with some delay upon HTTP 429 responses.
*
* The version of the OpenAPI document: 1.1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.confidentify.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* PersonNameBase
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-04-21T21:21:06.848810800+02:00[Europe/Paris]")
public class PersonNameBase {
public static final String SERIALIZED_NAME_GIVEN = "given";
@SerializedName(SERIALIZED_NAME_GIVEN)
private String given;
public static final String SERIALIZED_NAME_MIDDLE = "middle";
@SerializedName(SERIALIZED_NAME_MIDDLE)
private String middle;
public static final String SERIALIZED_NAME_FAMILY = "family";
@SerializedName(SERIALIZED_NAME_FAMILY)
private String family;
public static final String SERIALIZED_NAME_SUFFIX = "suffix";
@SerializedName(SERIALIZED_NAME_SUFFIX)
private String suffix;
public PersonNameBase given(String given) {
this.given = given;
return this;
}
/**
* Get given
* @return given
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Bill", value = "")
public String getGiven() {
return given;
}
public void setGiven(String given) {
this.given = given;
}
public PersonNameBase middle(String middle) {
this.middle = middle;
return this;
}
/**
* Get middle
* @return middle
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Randal", value = "")
public String getMiddle() {
return middle;
}
public void setMiddle(String middle) {
this.middle = middle;
}
public PersonNameBase family(String family) {
this.family = family;
return this;
}
/**
* Get family
* @return family
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "van Gogh", value = "")
public String getFamily() {
return family;
}
public void setFamily(String family) {
this.family = family;
}
public PersonNameBase suffix(String suffix) {
this.suffix = suffix;
return this;
}
/**
* Get suffix
* @return suffix
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Senior", value = "")
public String getSuffix() {
return suffix;
}
public void setSuffix(String suffix) {
this.suffix = suffix;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PersonNameBase personNameBase = (PersonNameBase) o;
return Objects.equals(this.given, personNameBase.given) &&
Objects.equals(this.middle, personNameBase.middle) &&
Objects.equals(this.family, personNameBase.family) &&
Objects.equals(this.suffix, personNameBase.suffix);
}
@Override
public int hashCode() {
return Objects.hash(given, middle, family, suffix);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PersonNameBase {\n");
sb.append(" given: ").append(toIndentedString(given)).append("\n");
sb.append(" middle: ").append(toIndentedString(middle)).append("\n");
sb.append(" family: ").append(toIndentedString(family)).append("\n");
sb.append(" suffix: ").append(toIndentedString(suffix)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy