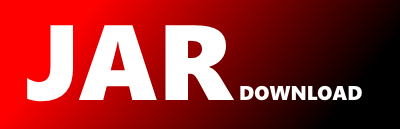
com.configcat.ConfigurationProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configcat-java-client Show documentation
Show all versions of configcat-java-client Show documentation
Java SDK for ConfigCat, a feature flag, feature toggle, and configuration management service. That lets you launch new features and change your software configuration remotely without actually (re)deploying code. ConfigCat even helps you do controlled roll-outs like canary releases and blue-green deployments.
The newest version!
package com.configcat;
import java.io.Closeable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
/**
* Defines the public interface of the {@link ConfigCatClient}.
*/
public interface ConfigurationProvider extends Closeable {
/**
* Gets the value of a feature flag or setting as T identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return the configuration value identified by the given key.
*/
T getValue(Class classOfT, String key, T defaultValue);
/**
* Gets the value of a feature flag or setting as T identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param user the user object.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return the configuration value identified by the given key.
*/
T getValue(Class classOfT, String key, User user, T defaultValue);
/**
* Gets the value of a feature flag or setting as T asynchronously identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return a future which computes the configuration value identified by the given key.
*/
CompletableFuture getValueAsync(Class classOfT, String key, T defaultValue);
/**
* Gets the value of a feature flag or setting as T asynchronously identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param user the user object.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return a future which computes the configuration value identified by the given key.
*/
CompletableFuture getValueAsync(Class classOfT, String key, User user, T defaultValue);
/**
* Gets the value of a feature flag or setting as T identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return the result of the evaluation.
*/
EvaluationDetails getValueDetails(Class classOfT, String key, T defaultValue);
/**
* Gets the value of a feature flag or setting as T identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param user the user object.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return the result of the evaluation.
*/
EvaluationDetails getValueDetails(Class classOfT, String key, User user, T defaultValue);
/**
* Gets the value of a feature flag or setting as T asynchronously identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return a future which computes the evaluation details.
*/
CompletableFuture> getValueDetailsAsync(Class classOfT, String key, T defaultValue);
/**
* Gets the value of a feature flag or setting as T asynchronously identified by the given {@code key}.
*
* @param classOfT the class of T. Only {@link String}, {@link Integer}, {@link Double} or {@link Boolean} types are supported.
* @param key the identifier of the feature flag or setting.
* @param user the user object.
* @param defaultValue in case of any failure, this value will be returned.
* @param the type of the desired feature flag or setting.
* @return a future which computes the evaluation details.
*/
CompletableFuture> getValueDetailsAsync(Class classOfT, String key, User user, T defaultValue);
/**
* Gets the values of all feature flags or settings synchronously.
*
* @return a collection of all values.
*/
Map getAllValues();
/**
* Gets the values of all feature flags or settings synchronously.
*
* @param user the user object.
* @return a collection of all values.
*/
Map getAllValues(User user);
/**
* Gets the values of all feature flags or settings asynchronously.
*
* @return a future which computes the collection of all values.
*/
CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy