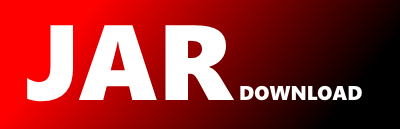
com.configcat.publicapi.java.client.model.RolloutRuleComparator Maven / Gradle / Ivy
/*
* ConfigCat Public Management API
* The purpose of this API is to access the ConfigCat platform programmatically. You can **Create**, **Read**, **Update** and **Delete** any entities like **Feature Flags, Configs, Environments** or **Products** within ConfigCat. **Base API URL**: https://api.configcat.com If you prefer the swagger documentation, you can find it here: [Swagger UI](https://api.configcat.com/swagger). The API is based on HTTP REST, uses resource-oriented URLs, status codes and supports JSON format. **Important:** Do not use this API for accessing and evaluating feature flag values. Use the [SDKs](https://configcat.com/docs/sdk-reference/overview) or the [ConfigCat Proxy](https://configcat.com/docs/advanced/proxy/proxy-overview/) instead. # OpenAPI Specification The complete specification is publicly available in the following formats: - [OpenAPI v3](https://api.configcat.com/docs/v1/swagger.json) - [Swagger v2](https://api.configcat.com/docs/v1/swagger.v2.json) You can use it to generate client libraries in various languages with [OpenAPI Generator](https://github.com/OpenAPITools/openapi-generator) or [Swagger Codegen](https://swagger.io/tools/swagger-codegen/) to interact with this API. # Authentication This API uses the [Basic HTTP Authentication Scheme](https://en.wikipedia.org/wiki/Basic_access_authentication). # Throttling and rate limits All the rate limited API calls are returning information about the current rate limit period in the following HTTP headers: | Header | Description | | :- | :- | | X-Rate-Limit-Remaining | The maximum number of requests remaining in the current rate limit period. | | X-Rate-Limit-Reset | The time when the current rate limit period resets. | When the rate limit is exceeded by a request, the API returns with a `HTTP 429 - Too many requests` status along with a `Retry-After` HTTP header.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.configcat.publicapi.java.client.model;
import com.google.gson.JsonElement;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
/**
* The comparison operator the evaluation process must use when it compares the given user
* attribute's value with the comparison value.
*/
@JsonAdapter(RolloutRuleComparator.Adapter.class)
public enum RolloutRuleComparator {
IS_ONE_OF("isOneOf"),
IS_NOT_ONE_OF("isNotOneOf"),
CONTAINS("contains"),
DOES_NOT_CONTAIN("doesNotContain"),
SEM_VER_IS_ONE_OF("semVerIsOneOf"),
SEM_VER_IS_NOT_ONE_OF("semVerIsNotOneOf"),
SEM_VER_LESS("semVerLess"),
SEM_VER_LESS_OR_EQUALS("semVerLessOrEquals"),
SEM_VER_GREATER("semVerGreater"),
SEM_VER_GREATER_OR_EQUALS("semVerGreaterOrEquals"),
NUMBER_EQUALS("numberEquals"),
NUMBER_DOES_NOT_EQUAL("numberDoesNotEqual"),
NUMBER_LESS("numberLess"),
NUMBER_LESS_OR_EQUALS("numberLessOrEquals"),
NUMBER_GREATER("numberGreater"),
NUMBER_GREATER_OR_EQUALS("numberGreaterOrEquals"),
SENSITIVE_IS_ONE_OF("sensitiveIsOneOf"),
SENSITIVE_IS_NOT_ONE_OF("sensitiveIsNotOneOf");
private String value;
RolloutRuleComparator(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static RolloutRuleComparator fromValue(String value) {
for (RolloutRuleComparator b : RolloutRuleComparator.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final RolloutRuleComparator enumeration)
throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public RolloutRuleComparator read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return RolloutRuleComparator.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
RolloutRuleComparator.fromValue(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy