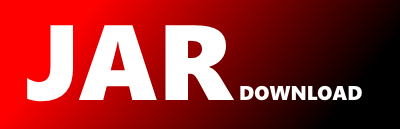
com.configcat.publicapi.java.client.model.SdkKeysModel Maven / Gradle / Ivy
/*
* ConfigCat Public Management API
* The purpose of this API is to access the ConfigCat platform programmatically. You can **Create**, **Read**, **Update** and **Delete** any entities like **Feature Flags, Configs, Environments** or **Products** within ConfigCat. **Base API URL**: https://api.configcat.com If you prefer the swagger documentation, you can find it here: [Swagger UI](https://api.configcat.com/swagger). The API is based on HTTP REST, uses resource-oriented URLs, status codes and supports JSON format. **Important:** Do not use this API for accessing and evaluating feature flag values. Use the [SDKs](https://configcat.com/docs/sdk-reference/overview) or the [ConfigCat Proxy](https://configcat.com/docs/advanced/proxy/proxy-overview/) instead. # OpenAPI Specification The complete specification is publicly available in the following formats: - [OpenAPI v3](https://api.configcat.com/docs/v1/swagger.json) - [Swagger v2](https://api.configcat.com/docs/v1/swagger.v2.json) You can use it to generate client libraries in various languages with [OpenAPI Generator](https://github.com/OpenAPITools/openapi-generator) or [Swagger Codegen](https://swagger.io/tools/swagger-codegen/) to interact with this API. # Authentication This API uses the [Basic HTTP Authentication Scheme](https://en.wikipedia.org/wiki/Basic_access_authentication). # Throttling and rate limits All the rate limited API calls are returning information about the current rate limit period in the following HTTP headers: | Header | Description | | :- | :- | | X-Rate-Limit-Remaining | The maximum number of requests remaining in the current rate limit period. | | X-Rate-Limit-Reset | The time when the current rate limit period resets. | When the rate limit is exceeded by a request, the API returns with a `HTTP 429 - Too many requests` status along with a `Retry-After` HTTP header.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.configcat.publicapi.java.client.model;
import com.configcat.publicapi.java.client.JSON;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.TypeAdapter;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.annotations.SerializedName;
import com.google.gson.reflect.TypeToken;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import org.openapitools.jackson.nullable.JsonNullable;
/** SdkKeysModel */
@javax.annotation.Generated(
value = "org.openapitools.codegen.languages.JavaClientCodegen",
date = "2024-10-09T12:38:06.739118192Z[Etc/UTC]",
comments = "Generator version: 7.7.0")
public class SdkKeysModel {
public static final String SERIALIZED_NAME_PRIMARY = "primary";
@SerializedName(SERIALIZED_NAME_PRIMARY)
private String primary;
public static final String SERIALIZED_NAME_SECONDARY = "secondary";
@SerializedName(SERIALIZED_NAME_SECONDARY)
private String secondary;
public SdkKeysModel() {}
public SdkKeysModel primary(String primary) {
this.primary = primary;
return this;
}
/**
* The primary SDK key.
*
* @return primary
*/
@javax.annotation.Nullable
public String getPrimary() {
return primary;
}
public void setPrimary(String primary) {
this.primary = primary;
}
public SdkKeysModel secondary(String secondary) {
this.secondary = secondary;
return this;
}
/**
* The secondary SDK key.
*
* @return secondary
*/
@javax.annotation.Nullable
public String getSecondary() {
return secondary;
}
public void setSecondary(String secondary) {
this.secondary = secondary;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SdkKeysModel sdkKeysModel = (SdkKeysModel) o;
return Objects.equals(this.primary, sdkKeysModel.primary)
&& Objects.equals(this.secondary, sdkKeysModel.secondary);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null
&& b != null
&& a.isPresent()
&& b.isPresent()
&& Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(primary, secondary);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] {a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SdkKeysModel {\n");
sb.append(" primary: ").append(toIndentedString(primary)).append("\n");
sb.append(" secondary: ").append(toIndentedString(secondary)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first
* line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("primary");
openapiFields.add("secondary");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to SdkKeysModel
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!SdkKeysModel.openapiRequiredFields
.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(
String.format(
"The required field(s) %s in SdkKeysModel is not found in the"
+ " empty JSON string",
SdkKeysModel.openapiRequiredFields.toString()));
}
}
Set> entries = jsonElement.getAsJsonObject().entrySet();
// check to see if the JSON string contains additional fields
for (Map.Entry entry : entries) {
if (!SdkKeysModel.openapiFields.contains(entry.getKey())) {
throw new IllegalArgumentException(
String.format(
"The field `%s` in the JSON string is not defined in the"
+ " `SdkKeysModel` properties. JSON: %s",
entry.getKey(), jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("primary") != null && !jsonObj.get("primary").isJsonNull())
&& !jsonObj.get("primary").isJsonPrimitive()) {
throw new IllegalArgumentException(
String.format(
"Expected the field `primary` to be a primitive type in the JSON"
+ " string but got `%s`",
jsonObj.get("primary").toString()));
}
if ((jsonObj.get("secondary") != null && !jsonObj.get("secondary").isJsonNull())
&& !jsonObj.get("secondary").isJsonPrimitive()) {
throw new IllegalArgumentException(
String.format(
"Expected the field `secondary` to be a primitive type in the JSON"
+ " string but got `%s`",
jsonObj.get("secondary").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!SdkKeysModel.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'SdkKeysModel' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter =
gson.getDelegateAdapter(this, TypeToken.get(SdkKeysModel.class));
return (TypeAdapter)
new TypeAdapter() {
@Override
public void write(JsonWriter out, SdkKeysModel value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public SdkKeysModel read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
return thisAdapter.fromJsonTree(jsonElement);
}
}.nullSafe();
}
}
/**
* Create an instance of SdkKeysModel given an JSON string
*
* @param jsonString JSON string
* @return An instance of SdkKeysModel
* @throws IOException if the JSON string is invalid with respect to SdkKeysModel
*/
public static SdkKeysModel fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, SdkKeysModel.class);
}
/**
* Convert an instance of SdkKeysModel to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy