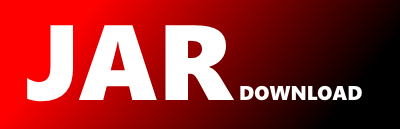
com.confluex.mock.http.matchers.HttpMatchers.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of confluex-mock-http Show documentation
Show all versions of confluex-mock-http Show documentation
Testing library for mocking interactions to external HTTP servers
package com.confluex.mock.http.matchers
import com.confluex.mock.http.ClientRequest
import org.hamcrest.BaseMatcher
import org.hamcrest.Description
import org.hamcrest.Matcher
import org.hamcrest.Matchers
import org.xml.sax.SAXException
import javax.xml.parsers.DocumentBuilderFactory
import javax.xml.xpath.XPathFactory
class HttpMatchers {
static HttpRequestMatcher anyRequest() {
new HttpRequestMatcher({
return true // matches everything
})
}
static HttpRequestMatcher path(String requestPath) {
path(Matchers.equalTo(requestPath))
}
static HttpRequestMatcher path(Matcher pathMatcher) {
new HttpRequestMatcher({ ClientRequest request ->
return pathMatcher.matches(request.path)
})
}
static HttpRequestMatcher method(Matcher methodMatcher) {
new HttpRequestMatcher({ ClientRequest request ->
return methodMatcher.matches(request.method)
})
}
static HttpRequestMatcher method(String requestMethod) {
method(Matchers.equalTo(requestMethod))
}
static HttpRequestMatcher get() {
method('GET')
}
static HttpRequestMatcher get(String requestPath) {
get().and(path(requestPath))
}
static HttpRequestMatcher get(Matcher pathMatcher) {
get().and(path(pathMatcher))
}
static HttpRequestMatcher put() {
method('PUT')
}
static HttpRequestMatcher put(String requestPath) {
put().and(path(requestPath))
}
static HttpRequestMatcher put(Matcher pathMatcher) {
put().and(path(pathMatcher))
}
static HttpRequestMatcher post() {
method('POST')
}
static HttpRequestMatcher post(String requestPath) {
post().and(path(requestPath))
}
static HttpRequestMatcher post(Matcher pathMatcher) {
post().and(path(pathMatcher))
}
static HttpRequestMatcher delete() {
method('DELETE')
}
static HttpRequestMatcher delete(String requestPath) {
delete().and(path(requestPath))
}
static HttpRequestMatcher delete(Matcher pathMatcher) {
delete().and(path(pathMatcher))
}
static HttpRequestMatcher body(String requestBody) {
return body(Matchers.equalTo(requestBody))
}
static HttpRequestMatcher body(Matcher bodyMatcher) {
new HttpRequestMatcher({ ClientRequest request ->
return bodyMatcher.matches(request.body)
})
}
static HttpRequestMatcher queryParam(String key) {
new HttpRequestMatcher({ ClientRequest request ->
return request.queryParams.containsKey(key)
})
}
static HttpRequestMatcher queryParam(String key, String value) {
queryParam(key, Matchers.equalTo(value))
}
static HttpRequestMatcher queryParam(String key, Matcher valueMatcher) {
new HttpRequestMatcher({ ClientRequest request ->
return valueMatcher.matches(request.queryParams[key])
})
}
static HttpRequestMatcher header(String key) {
new HttpRequestMatcher({ ClientRequest request ->
return request.headers.containsKey(key)
})
}
static HttpRequestMatcher header(String key, String value) {
header(key, Matchers.equalTo(value))
}
static HttpRequestMatcher header(String key, Matcher valueMatcher) {
new HttpRequestMatcher({ ClientRequest request ->
return valueMatcher.matches(request.headers[key])
})
}
static Matcher matchesPattern(final String regex) {
new BaseMatcher() {
@Override
boolean matches(Object o) {
return o ==~ regex
}
@Override
void describeTo(Description description) {
description.appendText("a string matching the regular expression ")
.appendValue(regex)
}
}
}
static Matcher stringHasXPath(final String xpath) {
stringHasXPath(xpath, Matchers.any(String))
}
static Matcher stringHasXPath(final String xpath, final Matcher valueMatcher) {
final def evaluator = XPathFactory.newInstance().newXPath()
new BaseMatcher() {
@Override
boolean matches(Object o) {
def builder = DocumentBuilderFactory.newInstance().newDocumentBuilder()
try {
def doc = builder.parse(new ByteArrayInputStream(o.bytes)).documentElement
return evaluator.evaluate(xpath, doc)
}
catch (SAXException e) {
return false
}
}
@Override
void describeTo(Description description) {
Matchers.hasXPath(xpath, valueMatcher).describeTo(description)
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy