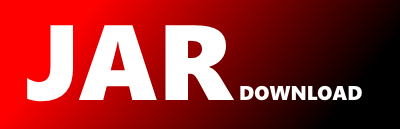
com.connectrpc.google.rpc.Status Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connect-kotlin-google-javalite-ext Show documentation
Show all versions of connect-kotlin-google-javalite-ext Show documentation
Simple, reliable, interoperable. A better RPC.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: google/rpc/status.proto
// Protobuf Java Version: 4.28.3
package com.connectrpc.google.rpc;
/**
*
* The `Status` type defines a logical error model that is suitable for
* different programming environments, including REST APIs and RPC APIs. It is
* used by [gRPC](https://github.com/grpc). Each `Status` message contains
* three pieces of data: error code, error message, and error details.
*
* You can find out more about this error model and how to work with it in the
* [API Design Guide](https://cloud.google.com/apis/design/errors).
*
*
* Protobuf type {@code google.rpc.Status}
*/
public final class Status extends
com.google.protobuf.GeneratedMessageLite<
Status, Status.Builder> implements
// @@protoc_insertion_point(message_implements:google.rpc.Status)
StatusOrBuilder {
private Status() {
message_ = "";
details_ = emptyProtobufList();
}
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
*
* The status code, which should be an enum value of
* [google.rpc.Code][google.rpc.Code].
*
*
* int32 code = 1 [json_name = "code"];
* @return The code.
*/
@java.lang.Override
public int getCode() {
return code_;
}
/**
*
* The status code, which should be an enum value of
* [google.rpc.Code][google.rpc.Code].
*
*
* int32 code = 1 [json_name = "code"];
* @param value The code to set.
*/
private void setCode(int value) {
code_ = value;
}
/**
*
* The status code, which should be an enum value of
* [google.rpc.Code][google.rpc.Code].
*
*
* int32 code = 1 [json_name = "code"];
*/
private void clearCode() {
code_ = 0;
}
public static final int MESSAGE_FIELD_NUMBER = 2;
private java.lang.String message_;
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
return message_;
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(message_);
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @param value The message to set.
*/
private void setMessage(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
message_ = value;
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
*/
private void clearMessage() {
message_ = getDefaultInstance().getMessage();
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @param value The bytes for message to set.
*/
private void setMessageBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
message_ = value.toStringUtf8();
}
public static final int DETAILS_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.ProtobufList details_;
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
@java.lang.Override
public java.util.List getDetailsList() {
return details_;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getDetailsOrBuilderList() {
return details_;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
@java.lang.Override
public int getDetailsCount() {
return details_.size();
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
@java.lang.Override
public com.google.protobuf.Any getDetails(int index) {
return details_.get(index);
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public com.google.protobuf.AnyOrBuilder getDetailsOrBuilder(
int index) {
return details_.get(index);
}
private void ensureDetailsIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = details_;
if (!tmp.isModifiable()) {
details_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
private void setDetails(
int index, com.google.protobuf.Any value) {
value.getClass();
ensureDetailsIsMutable();
details_.set(index, value);
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
private void addDetails(com.google.protobuf.Any value) {
value.getClass();
ensureDetailsIsMutable();
details_.add(value);
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
private void addDetails(
int index, com.google.protobuf.Any value) {
value.getClass();
ensureDetailsIsMutable();
details_.add(index, value);
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
private void addAllDetails(
java.lang.Iterable extends com.google.protobuf.Any> values) {
ensureDetailsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, details_);
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
private void clearDetails() {
details_ = emptyProtobufList();
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
private void removeDetails(int index) {
ensureDetailsIsMutable();
details_.remove(index);
}
public static com.connectrpc.google.rpc.Status parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.connectrpc.google.rpc.Status parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.connectrpc.google.rpc.Status parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.connectrpc.google.rpc.Status parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.connectrpc.google.rpc.Status parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.connectrpc.google.rpc.Status parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.connectrpc.google.rpc.Status parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.connectrpc.google.rpc.Status parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.connectrpc.google.rpc.Status parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.connectrpc.google.rpc.Status parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.connectrpc.google.rpc.Status parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.connectrpc.google.rpc.Status parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.connectrpc.google.rpc.Status prototype) {
return DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
* The `Status` type defines a logical error model that is suitable for
* different programming environments, including REST APIs and RPC APIs. It is
* used by [gRPC](https://github.com/grpc). Each `Status` message contains
* three pieces of data: error code, error message, and error details.
*
* You can find out more about this error model and how to work with it in the
* [API Design Guide](https://cloud.google.com/apis/design/errors).
*
*
* Protobuf type {@code google.rpc.Status}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.connectrpc.google.rpc.Status, Builder> implements
// @@protoc_insertion_point(builder_implements:google.rpc.Status)
com.connectrpc.google.rpc.StatusOrBuilder {
// Construct using com.connectrpc.google.rpc.Status.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
* The status code, which should be an enum value of
* [google.rpc.Code][google.rpc.Code].
*
*
* int32 code = 1 [json_name = "code"];
* @return The code.
*/
@java.lang.Override
public int getCode() {
return instance.getCode();
}
/**
*
* The status code, which should be an enum value of
* [google.rpc.Code][google.rpc.Code].
*
*
* int32 code = 1 [json_name = "code"];
* @param value The code to set.
* @return This builder for chaining.
*/
public Builder setCode(int value) {
copyOnWrite();
instance.setCode(value);
return this;
}
/**
*
* The status code, which should be an enum value of
* [google.rpc.Code][google.rpc.Code].
*
*
* int32 code = 1 [json_name = "code"];
* @return This builder for chaining.
*/
public Builder clearCode() {
copyOnWrite();
instance.clearCode();
return this;
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
return instance.getMessage();
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
return instance.getMessageBytes();
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
copyOnWrite();
instance.setMessage(value);
return this;
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @return This builder for chaining.
*/
public Builder clearMessage() {
copyOnWrite();
instance.clearMessage();
return this;
}
/**
*
* A developer-facing error message, which should be in English. Any
* user-facing error message should be localized and sent in the
* [google.rpc.Status.details][google.rpc.Status.details] field, or localized
* by the client.
*
*
* string message = 2 [json_name = "message"];
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setMessageBytes(value);
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
@java.lang.Override
public java.util.List getDetailsList() {
return java.util.Collections.unmodifiableList(
instance.getDetailsList());
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
@java.lang.Override
public int getDetailsCount() {
return instance.getDetailsCount();
}/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
@java.lang.Override
public com.google.protobuf.Any getDetails(int index) {
return instance.getDetails(index);
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder setDetails(
int index, com.google.protobuf.Any value) {
copyOnWrite();
instance.setDetails(index, value);
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder setDetails(
int index, com.google.protobuf.Any.Builder builderForValue) {
copyOnWrite();
instance.setDetails(index,
builderForValue.build());
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder addDetails(com.google.protobuf.Any value) {
copyOnWrite();
instance.addDetails(value);
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder addDetails(
int index, com.google.protobuf.Any value) {
copyOnWrite();
instance.addDetails(index, value);
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder addDetails(
com.google.protobuf.Any.Builder builderForValue) {
copyOnWrite();
instance.addDetails(builderForValue.build());
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder addDetails(
int index, com.google.protobuf.Any.Builder builderForValue) {
copyOnWrite();
instance.addDetails(index,
builderForValue.build());
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder addAllDetails(
java.lang.Iterable extends com.google.protobuf.Any> values) {
copyOnWrite();
instance.addAllDetails(values);
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder clearDetails() {
copyOnWrite();
instance.clearDetails();
return this;
}
/**
*
* A list of messages that carry the error details. There is a common set of
* message types for APIs to use.
*
*
* repeated .google.protobuf.Any details = 3 [json_name = "details"];
*/
public Builder removeDetails(int index) {
copyOnWrite();
instance.removeDetails(index);
return this;
}
// @@protoc_insertion_point(builder_scope:google.rpc.Status)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.connectrpc.google.rpc.Status();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"code_",
"message_",
"details_",
com.google.protobuf.Any.class,
};
java.lang.String info =
"\u0000\u0003\u0000\u0000\u0001\u0003\u0003\u0000\u0001\u0000\u0001\u0004\u0002\u0208" +
"\u0003\u001b";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.connectrpc.google.rpc.Status.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:google.rpc.Status)
private static final com.connectrpc.google.rpc.Status DEFAULT_INSTANCE;
static {
Status defaultInstance = new Status();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
Status.class, defaultInstance);
}
public static com.connectrpc.google.rpc.Status getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy