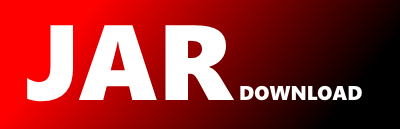
com.consol.citrus.functions.core.RandomEnumValueFunction Maven / Gradle / Ivy
/*
* Copyright 2006-2010 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.consol.citrus.functions.core;
import com.consol.citrus.context.TestContext;
import com.consol.citrus.exceptions.InvalidFunctionUsageException;
import com.consol.citrus.functions.Function;
import java.util.List;
import java.util.Random;
/**
* Function to choose one random value from a list of strings. The enumeration values to choose from
* can either be specified as parameters or in the {@link RandomEnumValueFunction#values} property of
* an instance of this class. These two possibilities can only be used exclusively - either empty values
* property and non-empty parameters or empty parameters and non-empty values property.
* Example custom function definition and the corresponding usage in a test:
*
*
*
* <bean id="myCustomFunctionLibrary" class="com.consol.citrus.functions.FunctionLibrary">
* <property name="name" value="myCustomFunctionLibrary" />
* <property name="prefix" value="custom:" />
* <property name="members">
* <map>
* <entry key="randomHttpStatusCode">
* <bean class="com.consol.citrus.functions.core.RandomEnumValueFunction">
* <property name="values">
* <list>
* <value>200</value>
* <value>500</value>
* <value>401</value>
* </list>
* </property>
* </bean>
* </entry>
* </map>
* </property>
* </bean>
*
*
* and the corresponding usage which sets the httpStatusCode to one of the configured values - 200, 500 or 401:
*
*
* <variable name="httpStatusCode" value="custom:randomHttpStatusCode()" />
*
*
* The other usage possibility is to choose a random value from a list of values given as argument
* like this which achieves the same result as the previously shown custom function:
*
*
* <variable name="httpStatusCode" value="citrus:randomEnumValue('200', '401', '500')" />
*
*
* You should choose which one of the two flavours to use based on the number of times you use this function - if you need it in
* only one special case you may go with specifying the list as arguments otherwise you should define a custom function and reuse it.
*
* @author Dimo Velev ([email protected])
*/
public class RandomEnumValueFunction implements Function {
private Random random = new Random(System.currentTimeMillis());
private List values = null;
/**
* @see Function#execute(java.util.List, com.consol.citrus.context.TestContext)
*/
public String execute(List params, TestContext context) {
if (values == null) {
return randomValue(params);
} else {
if (!params.isEmpty()) {
throw new InvalidFunctionUsageException("The enumeration values have already been set");
}
return randomValue(values);
}
}
/**
* Pseudo-randomly choose one of the supplied values and return it.
*
* @param values
* @return
* @throws IllegalArgumentException if the values supplied are null
or an empty list.
*/
protected String randomValue(List values) {
if (values == null || values.isEmpty()) {
throw new InvalidFunctionUsageException("No values to choose from");
}
final int idx = random.nextInt(values.size());
return values.get(idx);
}
public List getValues() {
return values;
}
public void setValues(List values) {
this.values = values;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy