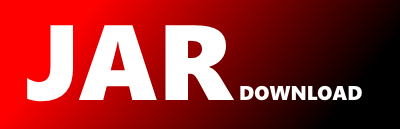
com.consol.citrus.doc.HtmlTestDocGenerator Maven / Gradle / Ivy
/*
* Copyright 2006-2010 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.consol.citrus.doc;
import com.consol.citrus.exceptions.CitrusRuntimeException;
import org.xml.sax.SAXException;
import javax.xml.transform.*;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import java.io.*;
import java.util.*;
/**
* Class to automatically generate a list of all available tests in HTML.
*
* @author Christoph Deppisch
* @since 2007
*/
public class HtmlTestDocGenerator extends AbstractTestDocGenerator {
/** Test doc specific information */
private String pageTitle = "Citrus Test Documentation";
private String overviewTitle = "Overview";
private String overviewColumns = "1";
private String logoFilePath = "logo.png";
/**
* Default constructor with test doc template name.
*/
public HtmlTestDocGenerator() {
super("CitrusTests.html", "testdoc.html.template");
}
@Override
public void doHeader(OutputStream buffered) throws TransformerException, IOException, SAXException {
List testFiles = getTestFiles();
int maxEntries = testFiles.size() / Integer.valueOf(getTestDocProperties().getProperty("overview.columns"));
buffered.write("".getBytes());
buffered.write("".getBytes());
for (int i = 0; i < testFiles.size(); i++) {
if (i != 0 && i % maxEntries == 0 && testFiles.size() - i >= maxEntries) {
buffered.write("
".getBytes());
buffered.write(" ".getBytes());
buffered.write("".getBytes());
buffered.write(("").getBytes());
}
buffered.write("- ".getBytes());
buffered.write(("").getBytes());
buffered.write(testFiles.get(i).getName().getBytes());
buffered.write("".getBytes());
}
buffered.write("
".getBytes());
buffered.write(" ".getBytes());
}
@Override
public void doBody(OutputStream buffered) throws TransformerException, IOException, SAXException {
StreamResult res = new StreamResult(buffered);
Transformer t = getTransformer("generate-html-doc.xslt", "text/html", "html");
int testNumber = 1;
for (File testFile : getTestFiles()) {
buffered.write("".getBytes());
Source xml = new DOMSource(getDocumentBuilder().parse(testFile));
buffered.write(("" + testNumber + ". ").getBytes());
buffered.write("".getBytes());
t.transform(xml, res);
buffered.write(("" + testFile.getName() + "").getBytes());
buffered.write(" ".getBytes());
buffered.write(" ".getBytes());
testNumber++;
}
}
/**
* Builds a new test doc generator.
* @return
*/
public static HtmlTestDocGenerator build() {
return new HtmlTestDocGenerator();
}
/**
* Adds a custom output file.
* @param filename the output file name.
* @return
*/
public HtmlTestDocGenerator withOutputFile(String filename) {
this.setOutputFile(filename);
return this;
}
/**
* Adds a custom page title.
* @param pageTitle the page title.
* @return
*/
public HtmlTestDocGenerator withPageTitle(String pageTitle) {
this.pageTitle = pageTitle;
return this;
}
/**
* Adds a custom overview title.
* @param overvieTitle the title.
* @return
*/
public HtmlTestDocGenerator withOverviewTitle(String overvieTitle) {
this.overviewTitle = overvieTitle;
return this;
}
/**
* Adds a column configuration.
* @param columns the column names.
* @return
*/
public HtmlTestDocGenerator withColumns(String columns) {
this.overviewColumns = columns;
return this;
}
/**
* Adds a custom logo file path.
* @param logoFilePath the file path.
* @return
*/
public HtmlTestDocGenerator withLogo(String logoFilePath) {
this.logoFilePath = logoFilePath;
return this;
}
/**
* Adds a custom test source directory.
* @param testDir the test source directory.
* @return
*/
public HtmlTestDocGenerator useSrcDirectory(String testDir) {
this.setSrcDirectory(testDir);
return this;
}
/**
* Executable application cli.
* @param args
*/
public static void main(String[] args) {
try {
HtmlTestDocGenerator creator = HtmlTestDocGenerator.build();
creator.useSrcDirectory(args.length == 1 ? args[0] : creator.srcDirectory)
.withOutputFile(args.length == 2 ? args[1] : creator.outputFile)
.withPageTitle(args.length == 3 ? args[2] : creator.pageTitle)
.withLogo(args.length == 4 ? args[3] : creator.logoFilePath)
.withOverviewTitle(args.length == 5 ? args[4] : creator.overviewTitle)
.withColumns(args.length == 6 ? args[5] : creator.overviewColumns);
creator.generateDoc();
} catch (ArrayIndexOutOfBoundsException e) {
throw new CitrusRuntimeException("Wrong usage exception! " +
"Use parameters in the following way: [test.directory] [output.file]", e);
}
}
@Override
protected Properties getTestDocProperties() {
Properties props = new Properties();
props.setProperty("page.title", pageTitle);
props.setProperty("overview.title", overviewTitle);
props.setProperty("overview.columns", overviewColumns);
props.setProperty("logo.file.path", logoFilePath);
props.setProperty("date", String.format("%1$tY-%1$tm-%1$td", new GregorianCalendar()));
return props;
}
/**
* @param pageTitle the pageTitle to set
*/
public void setPageTitle(String pageTitle) {
this.pageTitle = pageTitle;
}
/**
* @return the pageTitle
*/
public String getPageTitle() {
return pageTitle;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy