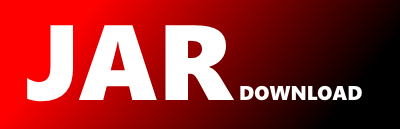
com.contentgrid.thunx.predicates.model.LogicalOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thunx-model Show documentation
Show all versions of thunx-model Show documentation
Set of (vendor-neutral) data structures to model authorization policy expressions
package com.contentgrid.thunx.predicates.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import lombok.EqualsAndHashCode;
@EqualsAndHashCode
public class LogicalOperation implements BooleanOperation {
private final Operator operator;
private List> terms;
private LogicalOperation(Operator operator, Stream> terms) {
this.operator = operator;
this.terms = terms.collect(Collectors.toUnmodifiableList());
}
public static LogicalOperation disjunction(Stream> terms) {
return new LogicalOperation(Operator.OR, terms);
}
public static LogicalOperation disjunction(List> terms) {
return disjunction(terms.stream());
}
public static LogicalOperation uncheckedDisjunction(List> terms) {
return disjunction(terms.stream().map(expr -> (ThunkExpression) expr));
}
public static LogicalOperation disjunction(ThunkExpression... terms) {
return disjunction(Arrays.stream(terms));
}
public static LogicalOperation conjunction(Stream> terms) {
return new LogicalOperation(Operator.AND, terms);
}
public static LogicalOperation conjunction(ThunkExpression... terms) {
return conjunction(Arrays.stream(terms));
}
public static LogicalOperation conjunction(List> terms) {
return conjunction(terms.stream());
}
public static LogicalOperation uncheckedConjunction(List> terms) {
return conjunction(terms.stream().map(expr -> (ThunkExpression) expr));
}
public static LogicalOperation uncheckedNegation(List> terms) {
if (terms.size() != 1) {
throw new IllegalArgumentException("Expected 1 term, not "+terms.size());
}
return negation((ThunkExpression) terms.get(0));
}
public static LogicalOperation negation(ThunkExpression term) {
return new LogicalOperation(Operator.NOT, Stream.of(term));
}
@Override
public Operator getOperator() {
return this.operator;
}
@Override
public List> getTerms() {
return Collections.unmodifiableList(this.terms);
}
@Override
public String toString() {
return this.toDebugString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy