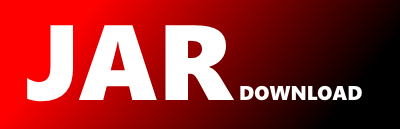
com.contentstack.sdk.Asset Maven / Gradle / Ivy
package com.contentstack.sdk;
import org.jetbrains.annotations.NotNull;
import org.json.JSONObject;
import retrofit2.Retrofit;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.logging.Logger;
import static com.contentstack.sdk.Constants.ENVIRONMENT;
import static com.contentstack.sdk.Constants.parseDate;
/**
* Assets
* refer to
* all the media files (images, videos, PDFs, audio files, and so on) uploaded
* in your Contentstack repository for
* future use. These files can be attached and used in multiple entries.
*
* You can now pass the branch header in the API request to fetch or manage
* modules located within specific branches of
* the stack.
*
* @author Shailesh Mishra
* @version 1.0.0
* @since 01-11-2017
*/
public class Asset {
protected static final Logger logger = Logger.getLogger(Asset.class.getSimpleName());
protected final JSONObject urlQueries = new JSONObject();
protected String assetUid = null;
protected String contentType = null;
protected String fileSize = null;
protected String fileName = null;
protected String uploadUrl = null;
protected JSONObject json = null;
protected String[] tagsArray = null;
protected LinkedHashMap headers;
protected Stack stackInstance;
protected Retrofit retrofit;
protected Asset() {
this.headers = new LinkedHashMap<>();
}
protected Asset(@NotNull String assetUid) {
this.assetUid = assetUid;
this.headers = new LinkedHashMap<>();
}
protected void setStackInstance(@NotNull Stack stack) {
this.stackInstance = stack;
this.headers = stack.headers;
}
/**
* Configure asset.
*
* @param jsonObject the json object
* @return the asset
*/
public Asset configure(JSONObject jsonObject) {
AssetModel model;
model = new AssetModel(jsonObject, true);
this.contentType = model.contentType;
this.fileSize = model.fileSize;
this.uploadUrl = model.uploadUrl;
this.fileName = model.fileName;
this.json = model.json;
this.assetUid = model.uploadedUid;
this.setTags(model.tags);
return this;
}
/**
* Sets header.
*
* @param headerKey the header key
* @param headerValue the header value
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment"); Asset asset =
* stack.asset(asset_uid); asset.setHeader();
*
*/
public void setHeader(@NotNull String headerKey, @NotNull String headerValue) {
headers.put(headerKey, headerValue);
}
/**
* The function removes a header from a collection of headers based on a given
* key.
*
* @param headerKey The parameter "headerKey" is a String that represents the
* key of the header to
* be removed.
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken",
* "environment"); Asset asset =
* stack.asset(asset_uid); asset.removeHeader();
*
*/
public void removeHeader(@NotNull String headerKey) {
headers.remove(headerKey);
}
protected void setUid(@NotNull String assetUid) {
if (!assetUid.isEmpty()) {
this.assetUid = assetUid;
}
}
/**
* Gets asset uid.
*
* @return the asset uid
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment"); Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() { @Override public void onCompletion(ResponseType responseType, Error
* error) { asset.getAssetUid(); } });
*
*
*/
public String getAssetUid() {
return assetUid;
}
/**
* Gets file type.
*
* @return the file type
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getFileType();
* }
* });
*
*/
public String getFileType() {
return contentType;
}
/**
* Gets file size.
*
* @return the file size
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getFileSize();
* }
* });
*
*
*/
public String getFileSize() {
return fileSize;
}
/**
* Gets file name.
*
* @return the file name
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getFileName();
* }
* });
*
*
*/
public String getFileName() {
return fileName;
}
/**
* Gets url.
*
* @return the url
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getUrl();
* }
* });
*
*
*/
public String getUrl() {
return uploadUrl;
}
/**
* To json json object.
*
* @return the json object
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.toJSON();
* }
* });
*
*
*/
public JSONObject toJSON() {
return json;
}
/**
* Gets create at.
*
* @return the create at
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getCreateAt();
* }
* });
*
*
*/
public Calendar getCreateAt() {
return parseDate(json.optString("created_at"), null);
}
/**
* Gets created by.
*
* @return the created by
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getCreatedBy();
* }
* });
*
*
*/
public String getCreatedBy() {
return json.optString("created_by");
}
/**
* Gets update at.
*
* @return the update at
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getUpdateAt();
* }
* });
*
*
*/
public Calendar getUpdateAt() {
return parseDate(json.optString("updated_at"), null);
}
/**
* Gets updated by.
*
* @return the updated by
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getUpdatedBy();
* }
* });
*
*
*/
public String getUpdatedBy() {
return json.optString("updated_by");
}
/**
* Gets delete at.
*
* @return the delete at
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getDeleteAt();
* }
* });
*
*
*/
public Calendar getDeleteAt() {
return parseDate(json.optString("deleted_at"), null);
}
/**
* Gets deleted by.
*
* @return the deleted by
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getDeletedBy();
* }
* });
*
*/
public String getDeletedBy() {
return json.optString("deleted_by");
}
/**
* Get tags string [ ].
*
* @return the string [ ]
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.fetch(new FetchResultCallback() {
* @Override
* public void onCompletion(ResponseType responseType, Error error) {
* asset.getTags();
* }
* });
*
*
*/
public String[] getTags() {
return tagsArray;
}
protected Asset setTags(String[] tags) {
tagsArray = tags;
return this;
}
/**
* Include dimension asset.
*
* @return the asset
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.includeDimension();
*
*/
public Asset includeDimension() {
urlQueries.put("include_dimension", true);
return this;
}
/**
* Add param asset.
*
* @param paramKey the param key
* @param paramValue the param value
* @return the asset
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.addParam();
*
*/
public Asset addParam(@NotNull String paramKey, @NotNull String paramValue) {
urlQueries.put(paramKey, paramValue);
return this;
}
/**
* Include fallback asset.
*
* @return the asset
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment");
* Asset asset = stack.asset(asset_uid);
* asset.includeFallback();
*
*/
public Asset includeFallback() {
urlQueries.put("include_fallback", true);
return this;
}
/**
* Includes Branch in the asset response
*
* @return {@link Asset} object, so you can chain this call.
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment"); Asset asset = stack.asset(asset_uid);
* asset.includeBranch();
*
*/
public Asset includeBranch() {
urlQueries.put("include_branch", true);
return this;
}
/**
* Includes Metadata in the asset response
*
* @return {@link Asset} object, so you can chain this call.
*
*
*
* Example :
*
*
* Stack stack = Contentstack.stack("apiKey", "deliveryToken", "environment"); Asset asset = stack.asset(asset_uid);
* asset.includeMetadata();
*
*/
public Asset includeMetadata() {
urlQueries.put("include_metadata", true);
return this;
}
/**
* Fetch.
*
* @param callback the callback
*/
public void fetch(FetchResultCallback callback) {
urlQueries.put(ENVIRONMENT, this.headers.get(ENVIRONMENT));
fetchFromNetwork("assets/" + assetUid, urlQueries, this.headers, callback);
}
private void fetchFromNetwork(String url, JSONObject urlQueries, LinkedHashMap headers,
FetchResultCallback callback) {
if (callback != null) {
HashMap urlParams = getUrlParams(urlQueries);
new CSBackgroundTask(this, stackInstance, Constants.FETCHASSETS, url, headers, urlParams,
Constants.REQUEST_CONTROLLER.ASSET.toString(), callback);
}
}
private HashMap getUrlParams(JSONObject urlQueriesJSON) {
HashMap hashMap = new HashMap<>();
if (urlQueriesJSON != null && urlQueriesJSON.length() > 0) {
Iterator keyArrays = urlQueriesJSON.keys();
while (keyArrays.hasNext()) {
String key = keyArrays.next();
Object value = urlQueriesJSON.opt(key);
hashMap.put(key, value);
}
}
return hashMap;
}
}