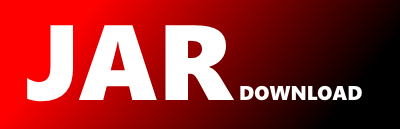
com.contentstack.sdk.Entry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java Show documentation
Show all versions of java Show documentation
Java SDK for Contentstack Content Delivery API
package com.contentstack.sdk;
import com.contentstack.sdk.utility.CSAppConstants;
import com.contentstack.sdk.utility.CSAppUtils;
import com.contentstack.sdk.utility.CSController;
import com.contentstack.sdk.utility.ContentstackUtil;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.*;
/**
*
* MIT License
*
* Copyright (c) 2012 - 2019 Contentstack
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
public class Entry {
private static final String TAG = "Entry";
private String contentTypeName = null;
private LinkedHashMap localHeader = null;
protected LinkedHashMap formHeader = null;
protected HashMap owner = null;
protected HashMap _metadata = null;
private ContentType contentTypeInstance = null;
private String[] tags = null;
protected String uid = null;
protected JSONObject resultJson = null;
protected String ownerEmailId = null;
protected String ownerUid = null;
protected String title = null;
protected String url = null;
protected String language = null;
private JSONArray referenceArray;
private JSONObject otherPostJSON;
private JSONArray objectUidForOnly;
private JSONArray objectUidForExcept;
private JSONObject onlyJsonObject;
private JSONObject exceptJsonObject;
private Entry(){}
protected Entry(String contentTypeName) {
this.contentTypeName = contentTypeName;
this.localHeader = new LinkedHashMap<>();
this.otherPostJSON = new JSONObject();
}
protected void setContentTypeInstance(ContentType contentTypeInstance) {
this.contentTypeInstance = contentTypeInstance;
}
public Entry configure(JSONObject jsonObject){
EntryModel model = new EntryModel(jsonObject, null,true,false,false);
this.resultJson = model.jsonObject;
this.ownerEmailId = model.ownerEmailId;
this.ownerUid = model.ownerUid;
this.title = model.title;
this.url = model.url;
this.language = model.language;
if(model.ownerMap != null) {
this.owner = new HashMap<>(model.ownerMap);
}
if(model._metadata != null) {
this._metadata = new HashMap<>(model._metadata);
}
this.uid = model.entryUid;
this.setTags(model.tags);
model = null;
return this;
}
/**
* Set headers.
* @param key
* custom_header_key
* @param value
* custom_header_value
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* entry.setHeader("custom_header_key", "custom_header_value");
*
*/
public void setHeader(String key, String value){
if(!key.isEmpty() && !value.isEmpty()){
localHeader.put(key, value);
}
}
/**
* Remove header key.
* @param key
* custom_header_key
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* entry.removeHeader("custom_header_key");
*
*/
public void removeHeader(String key){
if(!key.isEmpty()){
localHeader.remove(key);
}
}
/**
* Get title string
* @return String @title
*
Example :
*
* String title = entry.getTitle();
*
*/
public String getTitle(){ return title; }
/**
* Get url string
* @return String @url
*
Example :
*
* String url = entry.getURL();
*
*/
public String getURL(){ return url; }
/**
* Get tags.
* @return String @tags
*
Example :
*
* String[] tags = entry.getURL();
*
*/
public String[] getTags() {
return tags;
}
/**
* Get contentType name.
* @return String @contentTypeName
*
Example :
*
* String contentType = entry.getFileType();
*
*/
public String getContentType() {
return contentTypeName;
}
/**
* Get uid.
* @return String @uid
*
Example :
*
* String uid = entry.getUid();
*
*/
public String getUid() {
return uid;
}
/**
* Get metadata of entry.
*
Example :
*
* HashMap metaData = entry.getMetadata();
*
*/
private HashMap getMetadata() {
return _metadata;
}
/**
* Get {@link Language} instance
* @return Language @getLanguage
*
Example :
*
* Language local = entry.getLanguage();
*
*
*/
@Deprecated
public Language getLanguage(){
String localeCode = null;
if(_metadata != null && _metadata.size() > 0 && _metadata.containsKey("locale")){
localeCode = (String) _metadata.get("locale");
} else if(resultJson.has("locale")){
localeCode = (String) resultJson.optString("locale");
}
if(localeCode != null) {
localeCode = localeCode.replace("-", "_");
LanguageCode codeValue = LanguageCode.valueOf(localeCode);
int localeValue = codeValue.ordinal();
Language[] language = Language.values();
return language[localeValue];
}
return null;
}
/**
*
* @param locale {@link String}
* @return Entry
*
Example :
*
* Entry entry = entry.setLanguage();
*
*/
public Entry setLocale(String locale){
if (locale !=null){
try {
otherPostJSON.put("locale", locale);
} catch (JSONException e) {
e.printStackTrace();
}
}
return this;
}
public String getLocale(){
return this.language;
}
public HashMap getOwner() {
return owner;
}
/**
* Get entry representation in json
* @return JSONObject @resultJson
*
Example :
*
* JSONObject json = entry.toJSON();
*
*
*/
public JSONObject toJSON(){
return resultJson;
}
/**
* Get object value for key.
* @return Object @resultJson
* @param key
* field_uid as key.
*
Example :
*
* Object obj = entry.get("key");
*
*/
public Object get(String key){
try{
if(resultJson != null && key != null){
return resultJson.get(key);
}else{
return null;
}
}catch (Exception e) {
Stack.log(TAG, e.getLocalizedMessage());
return null;
}
}
/**
* Get string value for key.
* @return String @getString
* @param key field_uid as key.
*
Example :
*
* String value = entry.getString("key");
*
*/
public String getString(String key){
Object value = get(key);
if(value != null){
if(value instanceof String){
return (String) value;
}
}
return null;
}
/**
* Get boolean value for key.
* @return boolean @getBoolean
* @param key
* field_uid as key.
*
Example :
*
* Boolean value = entry.getBoolean("key");
*
*/
public Boolean getBoolean(String key){
Object value = get(key);
if(value != null){
if(value instanceof Boolean){
return (Boolean) value;
}
}
return false;
}
/**
* Get {@link JSONArray} value for key
* @return JSONArray @getJSONArray
* @param key
* field_uid as key.
*
Example :
*
* JSONArray value = entry.getJSONArray("key");
*
*/
public JSONArray getJSONArray(String key){
Object value = get(key);
if(value != null){
if(value instanceof JSONArray){
return (JSONArray) value;
}
}
return null;
}
/**
* Get {@link JSONObject} value for key
* @return JSONObject @getJSONObject
* @param key field_uid as key.
*
Example :
*
* JSONObject value = entry.getJSONObject("key");
*
*/
public JSONObject getJSONObject(String key){
Object value = get(key);
if(value != null){
if(value instanceof JSONObject){
return (JSONObject) value;
}
}
return null;
}
/**
* Get {@link JSONObject} value for key
* @return Number @getNumber
* @param key
* field_uid as key.
*
Example :
*
* JSONObject value = entry.getJSONObject("key");
*
*/
public Number getNumber(String key){
Object value = get(key);
if(value != null){
if(value instanceof Number){
return (Number) value;
}
}
return null;
}
/**
* Get integer value for key
* @return int @getInt
* @param key
* field_uid as key.
*
Example :
*
* int value = entry.getInt("key");
*
*/
public int getInt(String key){
Number value = getNumber(key);
if(value != null){
return value.intValue();
}
return 0;
}
/**
* Get integer value for key
* @param key
* field_uid as key.
* @return float @getFloat
*
Example :
*
* float value = entry.getFloat("key");
*
*/
public float getFloat(String key){
Number value = getNumber(key);
if(value != null){
return value.floatValue();
}
return (float) 0;
}
/**
* Get double value for key
* @param key field_uid as key.
* @return double @getDouble
*
Example :
*
* double value = entry.getDouble("key");
*
*/
public double getDouble(String key){
Number value = getNumber(key);
if(value != null){
return value.doubleValue();
}
return (double) 0;
}
/**
* Get long value for key
* @return long @getLong
* @param key field_uid as key.
*
Example :
*
* long value = entry.getLong("key");
*
*/
public long getLong(String key){
Number value = getNumber(key);
if(value != null){
return value.longValue();
}
return (long) 0;
}
/**
* Get short value for key
* @return short @getShort
* @param key
* field_uid as key.
*
*
Example :
*
* short value = entry.getShort("key");
*
*/
public short getShort(String key){
Number value = getNumber(key);
if(value != null){
return value.shortValue();
}
return (short) 0;
}
/**
* Get {@link Calendar} value for key
* @return Calendar @getDate
* @param key field_uid as key.
*
Example :
*
* Calendar value = entry.getDate("key");
*
*/
public Calendar getDate(String key){
try {
String value = getString(key);
return ContentstackUtil.parseDate(value, null);
} catch (Exception e) {
Stack.log(TAG, e.getLocalizedMessage());
}
return null;
}
/**
* Get {@link Calendar} value of creation time of entry.
* @return Calendar @getCreateAt
*
Example :
*
* Calendar createdAt = entry.getCreateAt("key");
*
*/
public Calendar getCreateAt(){
try {
String value = getString("created_at");
return ContentstackUtil.parseDate(value, null);
} catch (Exception e) {
Stack.log(TAG, e.getLocalizedMessage());
}
return null;
}
/**
* Get uid who created this entry.
* @return String @getCreatedBy
*
Example :
*
* String createdBy_uid = entry.getCreatedBy();
*
*/
public String getCreatedBy(){
return getString("created_by");
}
/**
* Get {@link Calendar} value of updating time of entry.
* @return Calendar @getUpdateAt
*
Example :
*
* Calendar updatedAt = entry.getUpdateAt("key");
*
*/
public Calendar getUpdateAt(){
try {
String value = getString("updated_at");
return ContentstackUtil.parseDate(value, null);
} catch (Exception e) {
Stack.log(TAG, e.getLocalizedMessage());
}
return null;
}
/**
* Get uid who updated this entry.
* @return String @getString
*
Example :
*
* String updatedBy_uid = entry.getUpdatedBy();
*
*/
public String getUpdatedBy(){
return getString("updated_by");
}
/**
* Get {@link Calendar} value of deletion time of entry.
*
* @return Calendar
*
*
Example :
*
* Calendar updatedAt = entry.getUpdateAt("key");
*
*/
public Calendar getDeleteAt(){
try {
String value = getString("deleted_at");
return ContentstackUtil.parseDate(value, null);
} catch (Exception e) {
Stack.log(TAG, e.getLocalizedMessage());
}
return null;
}
/**
* Get uid who deleted this entry.
* @return String
*
Example :
*
* String deletedBy_uid = entry.getDeletedBy();
*
*/
public String getDeletedBy(){
return getString("deleted_by");
}
/**
* Get an asset from the entry
* @param key
* field_uid as key.
* @return Asset
*
Example :
*
* Asset asset = entry.getAsset("key");
*
*/
public Asset getAsset(String key){
JSONObject assetObject = getJSONObject(key);
Asset asset = contentTypeInstance.stackInstance.asset().configure(assetObject);
return asset;
}
/**
* Get an assets from the entry. This works with multiple true fields
*
Example :
*
* {@code List asset = entry.getAssets("key"); }
*
* @param key This is the String key
* @return ArrayList This returns list of Assets.
*/
public List getAssets(String key){
List assets = new ArrayList<>();
JSONArray assetArray = getJSONArray(key);
for (int i = 0; i < assetArray.length(); i++) {
if(assetArray.opt(i) instanceof JSONObject){
Asset asset = contentTypeInstance.stackInstance.asset().configure(assetArray.optJSONObject(i));
assets.add(asset);
}
}
return assets;
}
/**
* Get a group from entry.
* @param key
* field_uid as key.
*
Example :
*
* Group innerGroup = entry.getGroup("key");
* @return null
*
*/
public Group getGroup(String key){
if(!key.isEmpty() && resultJson.has(key) && resultJson.opt(key) instanceof JSONObject ){
return new Group(contentTypeInstance.stackInstance, resultJson.optJSONObject(key));
}
return null;
}
/**
* Get a list of group from entry.
*
* Note :- This will work when group is multiple true.
* @param key field_uid as key.
* @return list of group from entry
*
Example :
*
* Group innerGroup = entry.getGroups("key");
*
*/
public List getGroups(String key){
if(!key.isEmpty() && resultJson.has(key) && resultJson.opt(key) instanceof JSONArray ){
JSONArray array = resultJson.optJSONArray(key);
List groupList = new ArrayList<>();
for (int i = 0; i < array.length(); i++) {
if(array.opt(i) instanceof JSONObject){
Group group = new Group(contentTypeInstance.stackInstance, array.optJSONObject(i));
groupList.add(group);
}
}
return groupList;
}
return null;
}
/**
* Get value for the given reference key.
* @param refKey key of a reference field.
* @param refContentType class uid.
* @return {@link ArrayList} of {@link Entry} instances.
* Also specified contentType value will be set as class uid for all {@link Entry} instance.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* {@code
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Query csQuery = stack.contentType("contentType_name").query();
* csQuery.includeReference("for_bug");
* csQuery.find(new QueryResultsCallBack() {
* @Override
* public void onCompletion(ResponseType responseType, QueryResult queryResult, Error error) {
* if(error == null){
* List<Entry> list = builtqueryresult.getResultObjects();
* for (int i = 0; i < list.queueSize(); i++) {
* Entry entry = list.get(i);
* Entry taskEntry = entry.getAllEntries("for_task", "task");
* }
* }
* }
* });
* }
*
*/
public ArrayList getAllEntries(String refKey, String refContentType) {
try {
if (resultJson != null) {
if (resultJson.get(refKey) instanceof JSONArray) {
int count = ((JSONArray) resultJson.get(refKey)).length();
ArrayList builtObjectList = new ArrayList();
for (int i = 0; i < count; i++) {
EntryModel model = new EntryModel(((JSONArray) resultJson.get(refKey)).getJSONObject(i), null, false, false, true);
Entry entryInstance = null;
try {
entryInstance = contentTypeInstance.stackInstance.contentType(refContentType).entry();
} catch (Exception e) {
entryInstance = new Entry(refContentType);
Stack.log("BuiltObject", "---------getAllEntries" + e.toString());
}
entryInstance.setUid(model.entryUid);
entryInstance.ownerEmailId = model.ownerEmailId;
entryInstance.ownerUid = model.ownerUid;
if(model.ownerMap != null) {
entryInstance.owner = new HashMap<>(model.ownerMap);
}
entryInstance.resultJson = model.jsonObject;
entryInstance.setTags(model.tags);
builtObjectList.add(entryInstance);
model = null;
}
return builtObjectList;
}
}
} catch (Exception e) {
Stack.log(TAG, "-----------------get|" + e);
return null;
}
return null;
}
/**
* Specifies list of field uids that would be 'excluded' from the response.
* @param fieldUid
* field uid which get 'excluded' from the response.
* @return {@link Entry} object, so you can chain this call.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* entry.except(new String[]{"name", "description"});
*
*/
public Entry except(String[] fieldUid){
try{
if(fieldUid != null && fieldUid.length > 0){
if(objectUidForExcept == null){
objectUidForExcept = new JSONArray();
}
int count = fieldUid.length;
for(int i = 0; i < count; i++){
objectUidForExcept.put(fieldUid[i]);
}
}
}catch(Exception e) {
Stack.log(TAG, "--except-catch|" + e);
}
return this;
}
/**
* Add a constraint that requires a particular reference key details.
* @param referenceField key that to be constrained.
* @return {@link Entry} object, so you can chain this call.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* entry.includeReference("referenceUid");
*
*/
public Entry includeReference(String referenceField) {
try {
if (!referenceField.isEmpty()) {
if (referenceArray == null) {
referenceArray = new JSONArray();
}
referenceArray.put(referenceField);
otherPostJSON.put("include[]", referenceArray);
}
} catch (Exception e) {
Stack.log(TAG, "--include Reference-catch|" + e);
}
return this;
}
/**
* Add a constraint that requires a particular reference key details.
* @param referenceFields array key that to be constrained.
* @return {@link Entry} object, so you can chain this call.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* entry.includeReference(new String[]{"referenceUid_A", "referenceUid_B"});
*
*/
public Entry includeReference(String[] referenceFields) {
try {
if (referenceFields != null && referenceFields.length > 0) {
if (referenceArray == null) {
referenceArray = new JSONArray();
}
for (int i = 0; i < referenceFields.length; i++) {
referenceArray.put(referenceFields[i]);
}
otherPostJSON.put("include[]", referenceArray);
}
} catch (Exception e) {
Stack.log(TAG, "--include Reference-catch|" + e);
}
return this;
}
/**
* Specifies an array of 'only' keys in BASE object that would be 'included' in the response.
* @param fieldUid
* Array of the 'only' reference keys to be included in response.
* @return
* {@link Entry} object, so you can chain this call.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* entry.only(new String[]{"name", "description"});
*
*/
public Entry only(String[] fieldUid) {
try{
if (fieldUid != null && fieldUid.length > 0) {
if(objectUidForOnly == null){
objectUidForOnly = new JSONArray();
}
int count = fieldUid.length;
for(int i = 0; i < count; i++){
objectUidForOnly.put(fieldUid[i]);
}
}
}catch(Exception e) {
Stack.log(TAG, "--include Reference-catch|" + e);
}
return this;
}
/**
* Specifies an array of 'only' keys that would be 'included' in the response.
* @param fieldUid
* Array of the 'only' reference keys to be included in response.
* @param referenceFieldUid
* Key who has reference to some other class object..
* @return {@link Entry} object, so you can chain this call.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* ArrayList<String> array = new ArrayList<String>();
* array.add("description");
* array.add("name");
* entry.onlyWithReferenceUid(array, "referenceUid");
*
*/
public Entry onlyWithReferenceUid(ArrayList fieldUid, String referenceFieldUid){
try{
if(fieldUid != null && referenceFieldUid != null){
if(onlyJsonObject == null){
onlyJsonObject = new JSONObject();
}
JSONArray fieldValueArray = new JSONArray();
int count = fieldUid.size();
for(int i = 0; i < count; i++){
fieldValueArray.put(fieldUid.get(i));
}
onlyJsonObject.put(referenceFieldUid, fieldValueArray);
includeReference(referenceFieldUid);
}
}catch(Exception e) {
Stack.log(TAG, "--onlyWithReferenceUid-catch|" + e);
}
return this;
}
/**
* Specifies an array of 'except' keys that would be 'excluded' in the response.
* @param fieldUid Array of the 'except' reference keys to be excluded in response.
* @param referenceFieldUid Key who has reference to some other class object.
* @return {@link Entry} object, so you can chain this call.
*
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* ArrayList<String> array = new ArrayList<String>();
* array.add("description");
* array.add("name");
* entry.onlyWithReferenceUid(array, "referenceUid");
*
*/
public Entry exceptWithReferenceUid(ArrayList fieldUid, String referenceFieldUid){
try{
if(fieldUid != null && referenceFieldUid != null){
if(exceptJsonObject == null){
exceptJsonObject = new JSONObject();
}
JSONArray fieldValueArray = new JSONArray();
int count = fieldUid.size();
for(int i = 0; i < count; i++){
fieldValueArray.put(fieldUid.get(i));
}
exceptJsonObject.put(referenceFieldUid, fieldValueArray);
includeReference(referenceFieldUid);
}
}catch(Exception e) {
Stack.log(TAG, "--exceptWithReferenceUid-catch|" + e);
}
return this;
}
protected void setTags(String[] tags) {
this.tags = tags;
}
protected void setUid(String uid) {
this.uid = uid;
}
/**
* Fetches the latest version of the entries from Contentstack.com content stack
* @param callBack
* {@link EntryResultCallBack} object to notify the application when the request has completed.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* {@code
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* Entry entry = stack.contentType("form_name").entry("entry_uid");
* entry.fetch(new BuiltResultCallBack() {
* @Override
* public void onCompletion(ResponseType responseType, BuiltError builtError) {
* }
* });
* }
*
*/
public void fetch(EntryResultCallBack callBack){
try {
if (!uid.isEmpty()) {
String URL = "/" + contentTypeInstance.stackInstance.VERSION + "/content_types/" + contentTypeName + "/entries/" + uid;
LinkedHashMap headers = getHeader(localHeader);
LinkedHashMap headerAll = new LinkedHashMap();
JSONObject urlQueries= new JSONObject();
if (headers != null && headers.size() > 0) {
for (Map.Entry entry : headers.entrySet()) {
headerAll.put(entry.getKey(), (String)entry.getValue());
}
if(headers.containsKey("environment")){
urlQueries.put("environment", headers.get("environment"));
}
}
fetchFromNetwork(URL, urlQueries, callBack);
}
}catch(Exception e){
throwException(null, e, callBack);
}
}
private void fetchFromNetwork(String URL, JSONObject urlQueries, EntryResultCallBack callBack){
try{
JSONObject mainJson = new JSONObject();
setIncludeJSON(urlQueries, callBack);
mainJson.put("query", urlQueries);
mainJson.put("_method", CSAppConstants.RequestMethod.GET.toString());
HashMap urlParams = getUrlParams(mainJson);
new CSBackgroundTask(this, contentTypeInstance.stackInstance, CSController.FETCHENTRY, URL, getHeader(localHeader), urlParams, new JSONObject(), CSAppConstants.callController.ENTRY.toString(), false, CSAppConstants.RequestMethod.GET, callBack);
}catch(Exception e){
throwException(null, e, callBack);
}
}
private LinkedHashMap getUrlParams(JSONObject jsonMain) {
JSONObject queryJSON = jsonMain.optJSONObject("query");
LinkedHashMap hashMap = new LinkedHashMap<>();
if(queryJSON != null && queryJSON.length() > 0){
Iterator iter = queryJSON.keys();
while (iter.hasNext()) {
String key = iter.next();
try {
Object value = queryJSON.opt(key);
hashMap.put(key, value);
} catch (Exception e) {
Stack.log(TAG, "----------------setQueryJson"+e.toString());
}
}
return hashMap;
}
return null;
}
private void setIncludeJSON(JSONObject mainJson, ResultCallBack callBack){
try {
Iterator iterator = otherPostJSON.keys();
while (iterator.hasNext()) {
String key = iterator.next();
Object value = otherPostJSON.get(key);
mainJson.put(key, value);
}
if(objectUidForOnly!= null && objectUidForOnly.length() > 0){
mainJson.put("only[BASE][]", objectUidForOnly);
objectUidForOnly = null;
}
if(objectUidForExcept != null && objectUidForExcept.length() > 0){
mainJson.put("except[BASE][]", objectUidForExcept);
objectUidForExcept = null;
}
if(exceptJsonObject != null && exceptJsonObject.length() > 0){
mainJson.put("except", exceptJsonObject);
exceptJsonObject = null;
}
if(onlyJsonObject != null && onlyJsonObject.length() > 0){
mainJson.put("only", onlyJsonObject);
onlyJsonObject = null;
}
}catch(Exception e){
throwException(null, e, (EntryResultCallBack)callBack);
}
}
private void throwException(String errorMsg, Exception e, EntryResultCallBack callBack) {
Error error = new Error();
if(errorMsg != null){
error.setErrorMessage(errorMsg);
}else{
error.setErrorMessage(e.toString());
}
callBack.onRequestFail(ResponseType.UNKNOWN, error);
}
private LinkedHashMap getHeader(LinkedHashMap localHeader) {
LinkedHashMap mainHeader = formHeader;
LinkedHashMap classHeaders = new LinkedHashMap<>();
if(localHeader != null && localHeader.size() > 0){
if(mainHeader != null && mainHeader.size() > 0) {
for (Map.Entry entry : localHeader.entrySet()) {
String key = entry.getKey();
classHeaders.put(key, entry.getValue());
}
for (Map.Entry entry : mainHeader.entrySet()) {
String key = entry.getKey();
if(!classHeaders.containsKey(key)) {
classHeaders.put(key, entry.getValue());
}
}
return classHeaders;
}else{
return localHeader;
}
}else{
return formHeader;
}
}
/**
* This method adds key and value to an Entry.
* @param key The key as string which needs to be added to an Entry
* @param value The value as string which needs to be added to an Entry
* @return {@link Entry}
*
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* {@code
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* final Entry entry = stack.contentType("user").entry("blt3b0aaebf6f1c3762");
* entry.addParam("include_dimensions", "true");
* entry.fetch(new BuiltResultCallBack() {
*
@
* Override
* public void onCompletion(ResponseType responseType, BuiltError builtError) {
* }
* });
* }
*
*
*
*/
public Entry addParam(String key, String value){
if(key != null && value != null){
try {
otherPostJSON.put(key, value);
} catch (JSONException e) {
e.printStackTrace();
}
}
return this;
}
/**
* This method also includes the content type UIDs of the referenced entries returned in the response
* @return {@link Entry}
*
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* {@code
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* final Entry entry = stack.contentType("user").entry("blt3b0aaebf6f1c3762");
* entry.includeReferenceContentTypeUID;
* entry.fetch(new BuiltResultCallBack() {
*
@
* Override
* public void onCompletion(ResponseType responseType, BuiltError builtError) {
* }
* });
* }
*
*
*
*/
public Entry includeReferenceContentTypeUID(){
try {
otherPostJSON.put("include_reference_content_type_uid", "true");
} catch (JSONException e) {
e.printStackTrace();
}
return this;
}
/**
* Include Content Type of all returned objects along with objects themselves.
* @return {@link Entry} object, so you can chain this call.
*
Example :
*
* //'blt5d4sample2633b' is a dummy Stack API key
* //'blt6d0240b5sample254090d' is dummy access token.
* Stack stack = Contentstack.stack(context, "blt5d4sample2633b", "blt6d0240b5sample254090d", "stag", false);
* final Entry entry = stack.contentType("user").entry("blt3b0aaebf6f1c3762");
* entry.includeContentType();
*
*/
public Entry includeContentType(){
try {
if (otherPostJSON.has("include_schema")){
otherPostJSON.remove("include_schema");
}
otherPostJSON.put("include_content_type",true);
otherPostJSON.put("include_global_field_schema",true);
} catch (Exception e) {
e.printStackTrace();
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy