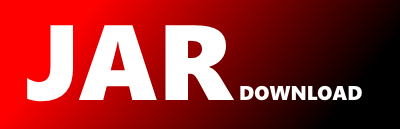
com.contentstack.sdk.marketplace.apps.AppService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marketplace Show documentation
Show all versions of marketplace Show documentation
Contentstack Java Management SDK for Content Management API
package com.contentstack.sdk.marketplace.apps;
import okhttp3.ResponseBody;
import org.json.simple.JSONObject;
import retrofit2.Call;
import retrofit2.http.*;
import java.util.Map;
/**
* The interface App service.
*/
public interface AppService {
/**
* Create installation call.
*
* @param headers the headers
* @param uid the uid
* @param body the body
* @param param the param
* @return the call
*/
@POST("manifests/{uid}/install")
Call createInstallation(
@HeaderMap Map headers,
@Path("uid") String uid,
@Body JSONObject body,
@QueryMap Map param);
/**
* Update version call.
*
* @param headers the headers
* @param id the id
* @param body the body
* @param queryParams the query params
* @return the call
*/
@PUT("manifests/{id}/reinstall")
Call updateVersion(
@HeaderMap Map headers,
@Path("id") String id,
@Body JSONObject body,
@QueryMap Map queryParams);
/**
* Find app authorizations call.
*
* @param headers the headers
* @param uid the uid
* @param queryParams the query params
* @return the call
*/
@GET("manifests/{uid}/authorizations")
Call findAppAuthorizations(
@HeaderMap Map headers,
@Path("uid") String uid, @QueryMap Map queryParams);
/**
* Delete authorization call.
*
* @param headers the headers
* @param uid the uid
* @param orgId the org id
* @return the call
*/
@DELETE("manifests/{uid}/authorizations/{uid}")
Call deleteAuthorization(
@HeaderMap Map headers,
@Path("uid") String uid,
@Path("uid") String orgId);
/**
* List app installations call.
*
* @param headers the headers
* @param uid the uid
* @param queryParameters the query parameters
* @return the call
*/
@GET("manifests/{uid}/installations")
Call listAppInstallations(
@HeaderMap Map headers,
@Path("uid") String uid,
@QueryMap Map queryParameters);
/**
* List apps call.
*
* @param headers the headers
* @param queryParameters the query parameters
* @return the call
*/
@GET("manifests")
Call listApps(
@HeaderMap Map headers,
@QueryMap Map queryParameters);
/**
* Create app call.
*
* @param headers the headers
* @param queryParameters the query parameters
* @param body the body
* @return the call
*/
@POST("manifests")
Call createApp(
@HeaderMap Map headers,
@QueryMap Map queryParameters,
@Body JSONObject body);
/**
* Fetch app call.
*
* @param headers the headers
* @param uid the uid
* @param queryParameters the query parameters
* @return the call
*/
@GET("manifests/{uid}")
Call fetchApp(
@HeaderMap Map headers,
@Path("uid") String uid,
@QueryMap Map queryParameters);
/**
* Update app call.
*
* @param headers the headers
* @param uid the uid
* @param body the body
* @return the call
*/
@PUT("manifests/{uid}")
Call updateApp(
@HeaderMap Map headers,
@Path("uid") String uid,
@Body JSONObject body);
/**
* Delete call.
*
* @param headers the headers
* @param uid the uid
* @return the call
*/
@DELETE("manifests/{uid}")
Call delete(
@HeaderMap Map headers,
@Path("uid") String uid);
/**
* List app requests call.
*
* @param headers the headers
* @param uid the uid
* @param params the params
* @return the call
*/
@GET("manifests/{uid}/requests")
Call listAppRequests(
@HeaderMap Map headers,
@Path("uid") String uid, @QueryMap Map params);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy