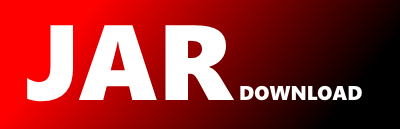
com.contrastsecurity.sdk.scan.AutoValue_CodeArtifactInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of contrast-sdk-java Show documentation
Show all versions of contrast-sdk-java Show documentation
Java SDK for using Contrast Security APIs
package com.contrastsecurity.sdk.scan;
/*-
* #%L
* Contrast Java SDK
* %%
* Copyright (C) 2021 Contrast Security, Inc.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.time.Instant;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_CodeArtifactInner extends CodeArtifactInner {
private final String id;
private final String projectId;
private final String organizationId;
private final String filename;
private final Instant createdTime;
private AutoValue_CodeArtifactInner(
String id,
String projectId,
String organizationId,
String filename,
Instant createdTime) {
this.id = id;
this.projectId = projectId;
this.organizationId = organizationId;
this.filename = filename;
this.createdTime = createdTime;
}
@Override
String id() {
return id;
}
@Override
String projectId() {
return projectId;
}
@Override
String organizationId() {
return organizationId;
}
@Override
String filename() {
return filename;
}
@Override
Instant createdTime() {
return createdTime;
}
@Override
public String toString() {
return "CodeArtifactInner{"
+ "id=" + id + ", "
+ "projectId=" + projectId + ", "
+ "organizationId=" + organizationId + ", "
+ "filename=" + filename + ", "
+ "createdTime=" + createdTime
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CodeArtifactInner) {
CodeArtifactInner that = (CodeArtifactInner) o;
return this.id.equals(that.id())
&& this.projectId.equals(that.projectId())
&& this.organizationId.equals(that.organizationId())
&& this.filename.equals(that.filename())
&& this.createdTime.equals(that.createdTime());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= projectId.hashCode();
h$ *= 1000003;
h$ ^= organizationId.hashCode();
h$ *= 1000003;
h$ ^= filename.hashCode();
h$ *= 1000003;
h$ ^= createdTime.hashCode();
return h$;
}
static final class Builder extends CodeArtifactInner.Builder {
private String id;
private String projectId;
private String organizationId;
private String filename;
private Instant createdTime;
Builder() {
}
@Override
CodeArtifactInner.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
CodeArtifactInner.Builder projectId(String projectId) {
if (projectId == null) {
throw new NullPointerException("Null projectId");
}
this.projectId = projectId;
return this;
}
@Override
CodeArtifactInner.Builder organizationId(String organizationId) {
if (organizationId == null) {
throw new NullPointerException("Null organizationId");
}
this.organizationId = organizationId;
return this;
}
@Override
CodeArtifactInner.Builder filename(String filename) {
if (filename == null) {
throw new NullPointerException("Null filename");
}
this.filename = filename;
return this;
}
@Override
CodeArtifactInner.Builder createdTime(Instant createdTime) {
if (createdTime == null) {
throw new NullPointerException("Null createdTime");
}
this.createdTime = createdTime;
return this;
}
@Override
CodeArtifactInner build() {
if (this.id == null
|| this.projectId == null
|| this.organizationId == null
|| this.filename == null
|| this.createdTime == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.projectId == null) {
missing.append(" projectId");
}
if (this.organizationId == null) {
missing.append(" organizationId");
}
if (this.filename == null) {
missing.append(" filename");
}
if (this.createdTime == null) {
missing.append(" createdTime");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CodeArtifactInner(
this.id,
this.projectId,
this.organizationId,
this.filename,
this.createdTime);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy