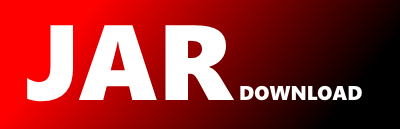
com.contrastsecurity.sdk.scan.AutoValue_ProjectInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of contrast-sdk-java Show documentation
Show all versions of contrast-sdk-java Show documentation
Java SDK for using Contrast Security APIs
package com.contrastsecurity.sdk.scan;
/*-
* #%L
* Contrast Java SDK
* %%
* Copyright (C) 2021 Contrast Security, Inc.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.contrastsecurity.sdk.internal.Nullable;
import java.time.Instant;
import java.util.Collection;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ProjectInner extends ProjectInner {
private final String id;
private final String organizationId;
private final String name;
private final boolean archived;
private final String language;
private final int critical;
private final int high;
private final int medium;
private final int low;
private final int note;
private final String lastScanId;
private final Instant lastScanTime;
private final int completedScans;
private final Collection includeNamespaceFilters;
private final Collection excludeNamespaceFilters;
private AutoValue_ProjectInner(
String id,
String organizationId,
String name,
boolean archived,
String language,
int critical,
int high,
int medium,
int low,
int note,
@Nullable String lastScanId,
@Nullable Instant lastScanTime,
int completedScans,
Collection includeNamespaceFilters,
Collection excludeNamespaceFilters) {
this.id = id;
this.organizationId = organizationId;
this.name = name;
this.archived = archived;
this.language = language;
this.critical = critical;
this.high = high;
this.medium = medium;
this.low = low;
this.note = note;
this.lastScanId = lastScanId;
this.lastScanTime = lastScanTime;
this.completedScans = completedScans;
this.includeNamespaceFilters = includeNamespaceFilters;
this.excludeNamespaceFilters = excludeNamespaceFilters;
}
@Override
String id() {
return id;
}
@Override
String organizationId() {
return organizationId;
}
@Override
String name() {
return name;
}
@Override
boolean archived() {
return archived;
}
@Override
String language() {
return language;
}
@Override
int critical() {
return critical;
}
@Override
int high() {
return high;
}
@Override
int medium() {
return medium;
}
@Override
int low() {
return low;
}
@Override
int note() {
return note;
}
@Nullable
@Override
String lastScanId() {
return lastScanId;
}
@Nullable
@Override
Instant lastScanTime() {
return lastScanTime;
}
@Override
int completedScans() {
return completedScans;
}
@Override
Collection includeNamespaceFilters() {
return includeNamespaceFilters;
}
@Override
Collection excludeNamespaceFilters() {
return excludeNamespaceFilters;
}
@Override
public String toString() {
return "ProjectInner{"
+ "id=" + id + ", "
+ "organizationId=" + organizationId + ", "
+ "name=" + name + ", "
+ "archived=" + archived + ", "
+ "language=" + language + ", "
+ "critical=" + critical + ", "
+ "high=" + high + ", "
+ "medium=" + medium + ", "
+ "low=" + low + ", "
+ "note=" + note + ", "
+ "lastScanId=" + lastScanId + ", "
+ "lastScanTime=" + lastScanTime + ", "
+ "completedScans=" + completedScans + ", "
+ "includeNamespaceFilters=" + includeNamespaceFilters + ", "
+ "excludeNamespaceFilters=" + excludeNamespaceFilters
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ProjectInner) {
ProjectInner that = (ProjectInner) o;
return this.id.equals(that.id())
&& this.organizationId.equals(that.organizationId())
&& this.name.equals(that.name())
&& this.archived == that.archived()
&& this.language.equals(that.language())
&& this.critical == that.critical()
&& this.high == that.high()
&& this.medium == that.medium()
&& this.low == that.low()
&& this.note == that.note()
&& (this.lastScanId == null ? that.lastScanId() == null : this.lastScanId.equals(that.lastScanId()))
&& (this.lastScanTime == null ? that.lastScanTime() == null : this.lastScanTime.equals(that.lastScanTime()))
&& this.completedScans == that.completedScans()
&& this.includeNamespaceFilters.equals(that.includeNamespaceFilters())
&& this.excludeNamespaceFilters.equals(that.excludeNamespaceFilters());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= organizationId.hashCode();
h$ *= 1000003;
h$ ^= name.hashCode();
h$ *= 1000003;
h$ ^= archived ? 1231 : 1237;
h$ *= 1000003;
h$ ^= language.hashCode();
h$ *= 1000003;
h$ ^= critical;
h$ *= 1000003;
h$ ^= high;
h$ *= 1000003;
h$ ^= medium;
h$ *= 1000003;
h$ ^= low;
h$ *= 1000003;
h$ ^= note;
h$ *= 1000003;
h$ ^= (lastScanId == null) ? 0 : lastScanId.hashCode();
h$ *= 1000003;
h$ ^= (lastScanTime == null) ? 0 : lastScanTime.hashCode();
h$ *= 1000003;
h$ ^= completedScans;
h$ *= 1000003;
h$ ^= includeNamespaceFilters.hashCode();
h$ *= 1000003;
h$ ^= excludeNamespaceFilters.hashCode();
return h$;
}
static final class Builder extends ProjectInner.Builder {
private String id;
private String organizationId;
private String name;
private Boolean archived;
private String language;
private Integer critical;
private Integer high;
private Integer medium;
private Integer low;
private Integer note;
private String lastScanId;
private Instant lastScanTime;
private Integer completedScans;
private Collection includeNamespaceFilters;
private Collection excludeNamespaceFilters;
Builder() {
}
@Override
ProjectInner.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
ProjectInner.Builder organizationId(String organizationId) {
if (organizationId == null) {
throw new NullPointerException("Null organizationId");
}
this.organizationId = organizationId;
return this;
}
@Override
ProjectInner.Builder name(String name) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
return this;
}
@Override
ProjectInner.Builder archived(boolean archived) {
this.archived = archived;
return this;
}
@Override
ProjectInner.Builder language(String language) {
if (language == null) {
throw new NullPointerException("Null language");
}
this.language = language;
return this;
}
@Override
ProjectInner.Builder critical(int critical) {
this.critical = critical;
return this;
}
@Override
ProjectInner.Builder high(int high) {
this.high = high;
return this;
}
@Override
ProjectInner.Builder medium(int medium) {
this.medium = medium;
return this;
}
@Override
ProjectInner.Builder low(int low) {
this.low = low;
return this;
}
@Override
ProjectInner.Builder note(int note) {
this.note = note;
return this;
}
@Override
ProjectInner.Builder lastScanId(String lastScanId) {
this.lastScanId = lastScanId;
return this;
}
@Override
ProjectInner.Builder lastScanTime(Instant lastScanTime) {
this.lastScanTime = lastScanTime;
return this;
}
@Override
ProjectInner.Builder completedScans(int completedScans) {
this.completedScans = completedScans;
return this;
}
@Override
ProjectInner.Builder includeNamespaceFilters(Collection includeNamespaceFilters) {
if (includeNamespaceFilters == null) {
throw new NullPointerException("Null includeNamespaceFilters");
}
this.includeNamespaceFilters = includeNamespaceFilters;
return this;
}
@Override
ProjectInner.Builder excludeNamespaceFilters(Collection excludeNamespaceFilters) {
if (excludeNamespaceFilters == null) {
throw new NullPointerException("Null excludeNamespaceFilters");
}
this.excludeNamespaceFilters = excludeNamespaceFilters;
return this;
}
@Override
ProjectInner build() {
if (this.id == null
|| this.organizationId == null
|| this.name == null
|| this.archived == null
|| this.language == null
|| this.critical == null
|| this.high == null
|| this.medium == null
|| this.low == null
|| this.note == null
|| this.completedScans == null
|| this.includeNamespaceFilters == null
|| this.excludeNamespaceFilters == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.organizationId == null) {
missing.append(" organizationId");
}
if (this.name == null) {
missing.append(" name");
}
if (this.archived == null) {
missing.append(" archived");
}
if (this.language == null) {
missing.append(" language");
}
if (this.critical == null) {
missing.append(" critical");
}
if (this.high == null) {
missing.append(" high");
}
if (this.medium == null) {
missing.append(" medium");
}
if (this.low == null) {
missing.append(" low");
}
if (this.note == null) {
missing.append(" note");
}
if (this.completedScans == null) {
missing.append(" completedScans");
}
if (this.includeNamespaceFilters == null) {
missing.append(" includeNamespaceFilters");
}
if (this.excludeNamespaceFilters == null) {
missing.append(" excludeNamespaceFilters");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ProjectInner(
this.id,
this.organizationId,
this.name,
this.archived,
this.language,
this.critical,
this.high,
this.medium,
this.low,
this.note,
this.lastScanId,
this.lastScanTime,
this.completedScans,
this.includeNamespaceFilters,
this.excludeNamespaceFilters);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy