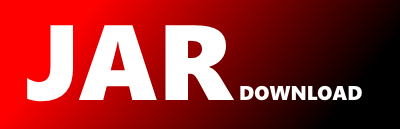
com.conveyal.gtfs.graphql.fetchers.NestedJDBCFetcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gtfs-lib Show documentation
Show all versions of gtfs-lib Show documentation
A library to load and index GTFS feeds of arbitrary size using disk-backed storage
package com.conveyal.gtfs.graphql.fetchers;
import com.conveyal.gtfs.graphql.GraphQLGtfsSchema;
import com.google.common.collect.ListMultimap;
import com.google.common.collect.MultimapBuilder;
import graphql.schema.DataFetcher;
import graphql.schema.DataFetchingEnvironment;
import graphql.schema.GraphQLFieldDefinition;
import graphql.schema.GraphQLList;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import static com.conveyal.gtfs.graphql.GraphQLUtil.multiStringArg;
import static com.conveyal.gtfs.graphql.GraphQLUtil.stringArg;
import static graphql.schema.GraphQLFieldDefinition.newFieldDefinition;
/**
* This fetcher uses nested calls to the general JDBCFetcher for SQL data. It uses the parent entity and a series of
* joins to leap frog from one entity to another more distantly-related entity. For example, if we want to know the
* routes that serve a specific stop, starting with a top-level stop type, we can nest joins from stop ABC -> pattern
* stops -> patterns -> routes (see below example implementation for more details).
*/
public class NestedJDBCFetcher implements DataFetcher>> {
private static final Logger LOG = LoggerFactory.getLogger(NestedJDBCFetcher.class);
private final JDBCFetcher[] jdbcFetchers;
// Supply an SQL result row -> Object transformer
/**
* Constructor for data that must be derived by chaining multiple joins together.
*/
public NestedJDBCFetcher (JDBCFetcher ...jdbcFetchers) {
this.jdbcFetchers = jdbcFetchers;
}
// NestedJDBCFetcher should only be used in conjunction with more than one JDBCFetcher in order to chain multiple
// joins together. As seen below, the type of the field must match the final JDBCFetcher table type (routeType ->
// routes).
public static GraphQLFieldDefinition field (String tableName) {
return newFieldDefinition()
.name(tableName)
// Field type should be equivalent to the final JDBCFetcher table type.
.type(new GraphQLList(GraphQLGtfsSchema.routeType))
.argument(stringArg("namespace"))
// Optional argument should match only the first join field.
.argument(multiStringArg("stop_id"))
.dataFetcher(new NestedJDBCFetcher(
// Auto limit is set to false in first fetchers because otherwise the limit could cut short the
// queries which first get the fields to join to routes.
new JDBCFetcher("pattern_stops", "stop_id", null, false),
new JDBCFetcher("patterns", "pattern_id", null, false),
new JDBCFetcher("routes", "route_id")))
.build();
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy