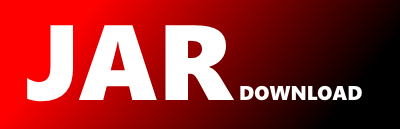
com.conveyal.gtfs.util.json.JsonManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gtfs-lib Show documentation
Show all versions of gtfs-lib Show documentation
A library to load and index GTFS feeds of arbitrary size using disk-backed storage
package com.conveyal.gtfs.util.json;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectWriter;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.fasterxml.jackson.databind.ser.impl.SimpleBeanPropertyFilter;
import com.fasterxml.jackson.databind.ser.impl.SimpleFilterProvider;
import java.awt.geom.Rectangle2D;
import java.io.IOException;
import java.time.LocalDate;
import java.util.Collection;
import java.util.Map;
/**
* Helper methods for writing REST API routines
* @author mattwigway
*
*/
public class JsonManager {
private ObjectWriter writer;
private ObjectMapper mapper;
SimpleFilterProvider filters;
/**
* Create a new JsonManager
* @param theClass The class to create a json manager for (yes, also in the diamonds).
*/
public JsonManager (Class theClass) {
this.theClass = theClass;
this.mapper = new ObjectMapper();
mapper.addMixInAnnotations(Rectangle2D.class, Rectangle2DMixIn.class);
// FIXME: This codepath is deprecated in versions greater than 4.x, but there may be a need to replace this
// Jackson module to handle GeoJSON. If there is an issue with de-/serialization, might be good to take a look
// at https://github.com/opentripplanner/OpenTripPlanner/blob/08e034faace255e092211290220af3f60e553fa7/pom.xml#L515-L532
// (dependency used in OTP).
// mapper.registerModule(new GeoJsonModule());
SimpleModule deser = new SimpleModule();
deser.addDeserializer(LocalDate.class, new JacksonSerializers.LocalDateStringDeserializer());
deser.addSerializer(LocalDate.class, new JacksonSerializers.LocalDateStringSerializer());
deser.addDeserializer(Rectangle2D.class, new Rectangle2DDeserializer());
mapper.registerModule(deser);
mapper.getSerializerProvider().setNullKeySerializer(new JacksonSerializers.MyDtoNullKeySerializer());
filters = new SimpleFilterProvider();
filters.addFilter("bbox", SimpleBeanPropertyFilter.filterOutAllExcept("west", "east", "south", "north"));
this.writer = mapper.writer(filters);
}
private Class theClass;
/**
* Add an additional mixin for serialization with this object mapper.
*/
public void addMixin(Class target, Class mixin) {
mapper.addMixInAnnotations(target, mixin);
}
public String write(Object o) throws JsonProcessingException {
if (o instanceof String) {
return (String) o;
}
return writer.writeValueAsString(o);
}
public String writePretty(Object o) throws JsonProcessingException {
if (o instanceof String) {
return (String) o;
}
return mapper.writerWithDefaultPrettyPrinter().with(filters).writeValueAsString(o);
}
/**
* Convert an object to its JSON representation
* @param o the object to convert
* @return the JSON string
* @throws JsonProcessingException
*/
/*public String write (T o) throws JsonProcessingException {
return writer.writeValueAsString(o);
}*/
/**
* Convert a collection of objects to their JSON representation.
* @param c the collection
* @return A JsonNode representing the collection
* @throws JsonProcessingException
*/
public String write (Collection c) throws JsonProcessingException {
return writer.writeValueAsString(c);
}
public String write (Map map) throws JsonProcessingException {
return writer.writeValueAsString(map);
}
public T read (String s) throws JsonParseException, JsonMappingException, IOException {
return mapper.readValue(s, theClass);
}
public T read (JsonParser p) throws JsonParseException, JsonMappingException, IOException {
return mapper.readValue(p, theClass);
}
public T read(JsonNode asJson) {
return mapper.convertValue(asJson, theClass);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy