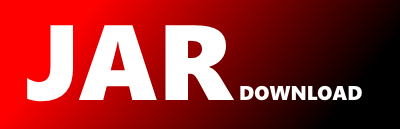
com.cosmicpush.gateway.push.UserEventPush Maven / Gradle / Ivy
/*
* Copyright (c) 2014 Jacob D. Parr
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cosmicpush.gateway.push;
import com.cosmicpush.gateway.CosmicPushGateway;
import com.cosmicpush.gateway.common.*;
import com.cosmicpush.gateway.internal.*;
import com.fasterxml.jackson.annotation.*;
import java.util.*;
import org.crazyyak.dev.common.*;
import org.crazyyak.dev.common.exceptions.*;
import org.joda.time.*;
public class UserEventPush implements Push, Comparable {
private boolean sendStory;
private String deviceId;
private String sessionId;
private String ipAddress;
private String userName;
private LocalDateTime createdAt;
private String message;
private LinkedHashMap traits = new LinkedHashMap();
private PushType pushType = PushType.userEvent;
private UserAgent userAgent;
private UserEventPush() {
}
public static UserEventPush sendStory(String sessionId, LocalDateTime createdAt) {
return new UserEventPush(sessionId, createdAt);
}
private UserEventPush(String sessionId, LocalDateTime createdAt) {
this.sendStory = true;
this.sessionId = ExceptionUtils.assertNotNull(sessionId, "sessionId");
this.createdAt = ExceptionUtils.assertNotNull(createdAt, "createdAt");
}
public UserEventPush(CpRemoteClient remoteClient, LocalDateTime createdAt, String message) {
this(remoteClient, createdAt, message, new LinkedHashMap());
}
public UserEventPush(CpRemoteClient remoteClient, LocalDateTime createdAt, String message, LinkedHashMap traits) {
this.sendStory = false;
this.deviceId = remoteClient.getDeviceId();
this.sessionId = ExceptionUtils.assertNotNull(remoteClient.getSessionId(), "sessionId");
this.userName = remoteClient.getUserName();
this.ipAddress = ExceptionUtils.assertNotNull(remoteClient.getIpAddress(), "ipAddress");
this.createdAt = ExceptionUtils.assertNotNull(createdAt, "createdAt");
this.message = (message == null) ? "No message" : message.trim();
this.traits.putAll(traits);
}
public boolean isSendStory() {
return sendStory;
}
@Override
public PushType getPushType() {
return pushType;
}
public String getMessage() {
return message;
}
public String getUserName() {
return userName;
}
public String getDeviceId() {
return deviceId;
}
public String getSessionId() {
return sessionId;
}
public String getIpAddress() {
return ipAddress;
}
public LocalDateTime getCreatedAt() {
return createdAt;
}
public LinkedHashMap getTraits() {
return traits;
}
public UserAgent getUserAgent() {
return userAgent;
}
public String getUserAgentString() {
if (traits == null) return null;
for (String key : Arrays.asList("User-Agent", "user-agent", "USER-AGENT", "User-agent", "user-Agent")) {
if (traits.get(key) != null)
return traits.get(key);
}
return null;
}
@Override
@JsonIgnore
public String getEndPoint() {
return CosmicPushGateway.PUSH_USER_EVENT;
}
@Override
public RequestErrors validate(RequestErrors errors) {
if (isSendStory() == false) {
ValidationUtils.requireValue(errors, message, "The message must be specified.");
}
return errors;
}
@Override
public int compareTo(UserEventPush that) {
return this.getCreatedAt().compareTo(that.getCreatedAt());
}
public boolean containsTrait(String key, Object value) {
if (traits == null) return false;
return BeanUtils.objectsEqual(traits.get(key), (value == null ? null : value.toString()));
}
public void assignUserAgent(UserAgent userAgent) {
this.userAgent = userAgent;
}
public String toString() {
return message;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy