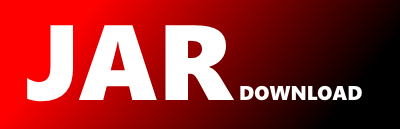
com.couchbase.client.CouchbaseClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of couchbase-client Show documentation
Show all versions of couchbase-client Show documentation
The official Couchbase Java SDK
/**
* Copyright (C) 2009-2013 Couchbase, Inc.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALING
* IN THE SOFTWARE.
*/
package com.couchbase.client;
import com.couchbase.client.clustermanager.FlushResponse;
import com.couchbase.client.internal.HttpFuture;
import com.couchbase.client.internal.ObserveFuture;
import com.couchbase.client.internal.ReplicaGetFuture;
import com.couchbase.client.internal.ViewFuture;
import com.couchbase.client.protocol.views.AbstractView;
import com.couchbase.client.protocol.views.DesignDocFetcherOperation;
import com.couchbase.client.protocol.views.DesignDocFetcherOperationImpl;
import com.couchbase.client.protocol.views.DesignDocOperationImpl;
import com.couchbase.client.protocol.views.DesignDocument;
import com.couchbase.client.protocol.views.DocsOperationImpl;
import com.couchbase.client.protocol.views.HttpOperation;
import com.couchbase.client.protocol.views.HttpOperationImpl;
import com.couchbase.client.protocol.views.InvalidViewException;
import com.couchbase.client.protocol.views.NoDocsOperationImpl;
import com.couchbase.client.protocol.views.Paginator;
import com.couchbase.client.protocol.views.Query;
import com.couchbase.client.protocol.views.ReducedOperationImpl;
import com.couchbase.client.protocol.views.SpatialView;
import com.couchbase.client.protocol.views.SpatialViewFetcherOperation;
import com.couchbase.client.protocol.views.SpatialViewFetcherOperationImpl;
import com.couchbase.client.protocol.views.View;
import com.couchbase.client.protocol.views.ViewFetcherOperation;
import com.couchbase.client.protocol.views.ViewFetcherOperationImpl;
import com.couchbase.client.protocol.views.ViewOperation.ViewCallback;
import com.couchbase.client.protocol.views.ViewResponse;
import com.couchbase.client.protocol.views.ViewRow;
import com.couchbase.client.vbucket.Reconfigurable;
import com.couchbase.client.vbucket.VBucketNodeLocator;
import com.couchbase.client.vbucket.config.Bucket;
import com.couchbase.client.vbucket.config.Config;
import com.couchbase.client.vbucket.config.ConfigType;
import net.spy.memcached.AddrUtil;
import net.spy.memcached.BroadcastOpFactory;
import net.spy.memcached.CASResponse;
import net.spy.memcached.CASValue;
import net.spy.memcached.CachedData;
import net.spy.memcached.MemcachedClient;
import net.spy.memcached.MemcachedNode;
import net.spy.memcached.ObserveResponse;
import net.spy.memcached.OperationTimeoutException;
import net.spy.memcached.PersistTo;
import net.spy.memcached.ReplicateTo;
import net.spy.memcached.internal.GetFuture;
import net.spy.memcached.internal.OperationCompletionListener;
import net.spy.memcached.internal.OperationFuture;
import net.spy.memcached.ops.GetOperation;
import net.spy.memcached.ops.GetlOperation;
import net.spy.memcached.ops.GetsOperation;
import net.spy.memcached.ops.ObserveOperation;
import net.spy.memcached.ops.Operation;
import net.spy.memcached.ops.OperationCallback;
import net.spy.memcached.ops.OperationStatus;
import net.spy.memcached.ops.ReplicaGetOperation;
import net.spy.memcached.ops.ReplicaGetsOperation;
import net.spy.memcached.ops.StatsOperation;
import net.spy.memcached.transcoders.Transcoder;
import org.apache.http.HttpRequest;
import org.apache.http.HttpVersion;
import org.apache.http.entity.StringEntity;
import org.apache.http.message.BasicHeader;
import org.apache.http.message.BasicHttpEntityEnclosingRequest;
import org.apache.http.message.BasicHttpRequest;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.InetSocketAddress;
import java.net.SocketAddress;
import java.net.URI;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.concurrent.CancellationException;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.concurrent.atomic.AtomicReference;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* A client for Couchbase Server.
*
* This class acts as your main entry point while working with your Couchbase
* cluster (if you want to work with TAP, see the TapClient instead).
*
* If you are working with Couchbase Server 2.0, remember to set the appropriate
* view mode depending on your environment.
*/
public class CouchbaseClient extends MemcachedClient
implements CouchbaseClientIF, Reconfigurable {
private static final String MODE_PRODUCTION = "production";
private static final String MODE_DEVELOPMENT = "development";
private static final String DEV_PREFIX = "dev_";
private static final String PROD_PREFIX = "";
public static final String MODE_PREFIX;
private static final String MODE_ERROR;
private ViewConnection vconn = null;
protected volatile boolean reconfiguring = false;
private final CouchbaseConnectionFactory cbConnFactory;
protected final ExecutorService executorService;
/**
* Try to load the cbclient.properties file and check for the viewmode.
*
* If no viewmode (either through "cbclient.viewmode" or "viewmode")
* property is set, the fallback is always "production". Possible options
* are either "development" or "production".
*/
static {
CouchbaseProperties.setPropertyFile("cbclient.properties");
String viewmode = CouchbaseProperties.getProperty("viewmode");
if (viewmode == null) {
viewmode = CouchbaseProperties.getProperty("viewmode", true);
}
if (viewmode == null) {
MODE_ERROR = "viewmode property isn't defined. Setting viewmode to"
+ " production mode";
MODE_PREFIX = PROD_PREFIX;
} else if (viewmode.equals(MODE_PRODUCTION)) {
MODE_ERROR = "viewmode set to production mode";
MODE_PREFIX = PROD_PREFIX;
} else if (viewmode.equals(MODE_DEVELOPMENT)) {
MODE_ERROR = "viewmode set to development mode";
MODE_PREFIX = DEV_PREFIX;
} else {
MODE_ERROR = "unknown value \"" + viewmode + "\" for property viewmode"
+ " Setting to production mode";
MODE_PREFIX = PROD_PREFIX;
}
}
/**
* Get a CouchbaseClient based on the initial server list provided.
*
* This constructor should be used if the bucket name is the same as the
* username (which is normally the case). If your bucket does not have
* a password (likely the "default" bucket), use an empty string instead.
*
* This method is only a convenience method so you don't have to create a
* CouchbaseConnectionFactory for yourself.
*
* @param baseList the URI list of one or more servers from the cluster
* @param bucketName the bucket name in the cluster you wish to use
* @param pwd the password for the bucket
* @throws IOException if connections could not be made
* @throws com.couchbase.client.vbucket.ConfigurationException if the
* configuration provided by the server has issues or is not
* compatible.
*/
public CouchbaseClient(final List baseList, final String bucketName,
final String pwd)
throws IOException {
this(new CouchbaseConnectionFactory(baseList, bucketName, pwd));
}
/**
* Get a CouchbaseClient based on the initial server list provided.
*
* Currently, Couchbase Server does not support a different username than the
* bucket name. Therefore, this method ignores the given username for now
* but will likely use it in the future.
*
* This constructor should be used if the bucket name is NOT the same as the
* username. If your bucket does not have a password (likely the "default"
* bucket), use an empty string instead.
*
* This method is only a convenience method so you don't have to create a
* CouchbaseConnectionFactory for yourself.
*
* @param baseList the URI list of one or more servers from the cluster
* @param bucketName the bucket name in the cluster you wish to use
* @param user the username for the bucket
* @param pwd the password for the bucket
* @throws IOException if connections could not be made
* @throws com.couchbase.client.vbucket.ConfigurationException if the
* configuration provided by the server has issues or is not
* compatible.
*/
public CouchbaseClient(final List baseList, final String bucketName,
final String user, final String pwd)
throws IOException {
this(new CouchbaseConnectionFactory(baseList, bucketName, pwd));
}
/**
* Get a CouchbaseClient based on the settings from the given
* CouchbaseConnectionFactory.
*
* If your bucket does not have a password (likely the "default" bucket), use
* an empty string instead.
*
* The URI list provided here is only used during the initial connection to
* the cluster. Afterwards, the actual cluster-map is synchronized from the
* cluster and maintained internally by the client. This allows the client to
* update the map as needed (when the cluster topology changes).
*
* Note that when specifying a ConnectionFactory you must specify a
* BinaryConnectionFactory (which is the case if you use the
* CouchbaseConnectionFactory). Also the ConnectionFactory's protocol and
* locator values are always overwritten. The protocol will always be binary
* and the locator will be chosen based on the bucket type you are connecting
* to.
*
* The subscribe variable determines whether or not we will subscribe to
* the configuration changes feed. This constructor should be used when
* calling super from subclasses of CouchbaseClient since the subclass might
* want to start the changes feed later.
*
* @param cf the ConnectionFactory to use to create connections
* @throws IOException if connections could not be made
* @throws com.couchbase.client.vbucket.ConfigurationException if the
* configuration provided by the server has issues or is not
* compatible.
*/
public CouchbaseClient(CouchbaseConnectionFactory cf)
throws IOException {
super(cf, AddrUtil.getAddresses(cf.getVBucketConfig().getServers()));
getLogger().info(cf.toString());
cbConnFactory = cf;
if(cf.getVBucketConfig().getConfigType() == ConfigType.COUCHBASE) {
List addrs =
AddrUtil.getAddressesFromURL(cf.getVBucketConfig().getCouchServers());
vconn = cf.createViewConnection(addrs);
}
executorService = cbConnFactory.getListenerExecutorService();
getLogger().info(MODE_ERROR);
cf.getConfigurationProvider().subscribe(this);
}
@Override
public void reconfigure(Bucket bucket) {
reconfiguring = true;
try {
if(vconn != null) {
vconn.reconfigure(bucket);
}
if (mconn instanceof CouchbaseConnection) {
CouchbaseConnection cbConn = (CouchbaseConnection) mconn;
cbConn.reconfigure(bucket);
} else {
CouchbaseMemcachedConnection cbMConn =
(CouchbaseMemcachedConnection) mconn;
cbMConn.reconfigure(bucket);
}
} catch (IllegalArgumentException ex) {
getLogger().warn("Failed to reconfigure client, staying with "
+ "previous configuration.", ex);
} finally {
reconfiguring = false;
}
}
@Override
public void connectionLost(SocketAddress sa) {
getLogger().debug("Connection lost for node: " + sa);
cbConnFactory.configurationProvider.reloadConfig();
super.connectionLost(sa);
}
@Override
public HttpFuture asyncGetView(String designDocumentName,
final String viewName) {
CouchbaseConnectionFactory factory =
(CouchbaseConnectionFactory) connFactory;
designDocumentName = MODE_PREFIX + designDocumentName;
String bucket = factory.getBucketName();
String uri = "/" + bucket + "/_design/" + designDocumentName;
final CountDownLatch couchLatch = new CountDownLatch(1);
final HttpFuture crv = new HttpFuture(couchLatch,
factory.getViewTimeout(), executorService);
final HttpRequest request =
new BasicHttpRequest("GET", uri, HttpVersion.HTTP_1_1);
final HttpOperation op =
new ViewFetcherOperationImpl(request, bucket, designDocumentName,
viewName, new ViewFetcherOperation.ViewFetcherCallback() {
private View view = null;
@Override
public void receivedStatus(OperationStatus status) {
crv.set(view, status);
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
@Override
public void gotData(View v) {
view = v;
}
});
crv.setOperation(op);
addOp(op);
assert crv != null : "Problem retrieving view";
return crv;
}
@Override
public HttpFuture asyncGetSpatialView(String designDocumentName,
final String viewName) {
CouchbaseConnectionFactory factory =
(CouchbaseConnectionFactory) connFactory;
designDocumentName = MODE_PREFIX + designDocumentName;
String bucket = factory.getBucketName();
String uri = "/" + bucket + "/_design/" + designDocumentName;
final CountDownLatch couchLatch = new CountDownLatch(1);
final HttpFuture crv = new HttpFuture(
couchLatch, factory.getViewTimeout(), executorService);
final HttpRequest request =
new BasicHttpRequest("GET", uri, HttpVersion.HTTP_1_1);
final HttpOperation op =
new SpatialViewFetcherOperationImpl(request, bucket, designDocumentName,
viewName, new SpatialViewFetcherOperation.ViewFetcherCallback() {
private SpatialView view = null;
@Override
public void receivedStatus(OperationStatus status) {
crv.set(view, status);
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
@Override
public void gotData(SpatialView v) {
view = v;
}
});
crv.setOperation(op);
addOp(op);
assert crv != null : "Problem retrieving spatial view";
return crv;
}
@Override
public HttpFuture asyncGetDesignDoc(
String designDocumentName) {
designDocumentName = MODE_PREFIX + designDocumentName;
String bucket = ((CouchbaseConnectionFactory)connFactory).getBucketName();
String uri = "/" + bucket + "/_design/" + designDocumentName;
final CountDownLatch couchLatch = new CountDownLatch(1);
final HttpFuture crv =
new HttpFuture(couchLatch, 60000, executorService);
final HttpRequest request =
new BasicHttpRequest("GET", uri, HttpVersion.HTTP_1_1);
final HttpOperation op = new DesignDocFetcherOperationImpl(
request,
designDocumentName,
new DesignDocFetcherOperation.DesignDocFetcherCallback() {
private DesignDocument design = null;
@Override
public void receivedStatus(OperationStatus status) {
crv.set(design, status);
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
@Override
public void gotData(DesignDocument d) {
design = d;
}
});
crv.setOperation(op);
addOp(op);
return crv;
}
@Override
public View getView(final String designDocumentName, final String viewName) {
try {
View view = asyncGetView(designDocumentName, viewName).get();
if(view == null) {
throw new InvalidViewException("Could not load view \""
+ viewName + "\" for design doc \"" + designDocumentName + "\"");
}
return view;
} catch (InterruptedException e) {
throw new RuntimeException("Interrupted getting views", e);
} catch (ExecutionException e) {
if(e.getCause() instanceof CancellationException) {
throw (CancellationException) e.getCause();
} else {
throw new RuntimeException("Failed getting views", e);
}
}
}
@Override
public SpatialView getSpatialView(final String designDocumentName,
final String viewName) {
try {
SpatialView view = asyncGetSpatialView(designDocumentName, viewName)
.get();
if(view == null) {
throw new InvalidViewException("Could not load spatial view \""
+ viewName + "\" for design doc \"" + designDocumentName + "\"");
}
return view;
} catch (InterruptedException e) {
throw new RuntimeException("Interrupted getting spatial view", e);
} catch (ExecutionException e) {
if(e.getCause() instanceof CancellationException) {
throw (CancellationException) e.getCause();
} else {
throw new RuntimeException("Failed getting views", e);
}
}
}
@Override
public DesignDocument getDesignDoc(final String designDocumentName) {
try {
DesignDocument design = asyncGetDesignDocument(designDocumentName).get();
if(design == null) {
throw new InvalidViewException("Could not load design document \""
+ designDocumentName + "\"");
}
return design;
} catch (InterruptedException e) {
throw new RuntimeException("Interrupted getting design document", e);
} catch (ExecutionException e) {
if(e.getCause() instanceof CancellationException) {
throw (CancellationException) e.getCause();
} else {
throw new RuntimeException("Failed getting design document", e);
}
}
}
@Override
@Deprecated
public HttpFuture asyncGetDesignDocument(
final String designDocumentName) {
return asyncGetDesignDoc(designDocumentName);
}
@Override
@Deprecated
public DesignDocument getDesignDocument(final String designDocumentName) {
return getDesignDoc(designDocumentName);
}
@Override
public Boolean createDesignDoc(final DesignDocument doc) {
try {
return asyncCreateDesignDoc(doc).get();
} catch (InterruptedException e) {
throw new RuntimeException("Interrupted creating design document", e);
} catch (ExecutionException e) {
if(e.getCause() instanceof CancellationException) {
throw (CancellationException) e.getCause();
} else {
throw new RuntimeException("Failed creating design document", e);
}
} catch (UnsupportedEncodingException e) {
throw new RuntimeException("Failed creating design document", e);
}
}
@Override
public HttpFuture asyncCreateDesignDoc(String name, String value)
throws UnsupportedEncodingException {
getLogger().info("Creating Design Document:" + name);
String bucket = ((CouchbaseConnectionFactory)connFactory).getBucketName();
final String uri = "/" + bucket + "/_design/" + MODE_PREFIX + name;
final CountDownLatch couchLatch = new CountDownLatch(1);
final HttpFuture crv = new HttpFuture(couchLatch, 60000,
executorService);
HttpRequest request = new BasicHttpEntityEnclosingRequest("PUT", uri,
HttpVersion.HTTP_1_1);
request.setHeader(new BasicHeader("Content-Type", "application/json"));
StringEntity entity = new StringEntity(value);
((BasicHttpEntityEnclosingRequest) request).setEntity(entity);
HttpOperationImpl op = new DesignDocOperationImpl(request,
new OperationCallback() {
@Override
public void receivedStatus(OperationStatus status) {
crv.set(status.getMessage().equals("Error Code: 201"), status);
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
});
crv.setOperation(op);
addOp(op);
return crv;
}
@Override
public HttpFuture asyncCreateDesignDoc(final DesignDocument doc)
throws UnsupportedEncodingException {
return asyncCreateDesignDoc(doc.getName(), doc.toJson());
}
@Override
public Boolean deleteDesignDoc(final String name) {
try {
return asyncDeleteDesignDoc(name).get();
} catch (InterruptedException e) {
throw new RuntimeException("Interrupted deleting design document", e);
} catch (ExecutionException e) {
if(e.getCause() instanceof CancellationException) {
throw (CancellationException) e.getCause();
} else {
throw new RuntimeException("Failed deleting design document", e);
}
} catch (UnsupportedEncodingException e) {
throw new RuntimeException("Failed deleting design document", e);
}
}
@Override
public HttpFuture asyncDeleteDesignDoc(final String name)
throws UnsupportedEncodingException {
getLogger().info("Deleting Design Document:" + name);
String bucket = ((CouchbaseConnectionFactory)connFactory).getBucketName();
final String uri = "/" + bucket + "/_design/" + MODE_PREFIX + name;
final CountDownLatch couchLatch = new CountDownLatch(1);
final HttpFuture crv = new HttpFuture(couchLatch, 60000,
executorService);
HttpRequest request = new BasicHttpEntityEnclosingRequest("DELETE", uri,
HttpVersion.HTTP_1_1);
request.setHeader(new BasicHeader("Content-Type", "application/json"));
HttpOperationImpl op = new DesignDocOperationImpl(request,
new OperationCallback() {
@Override
public void receivedStatus(OperationStatus status) {
crv.set(status.getMessage().equals("Error Code: 200"), status);
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
});
crv.setOperation(op);
addOp(op);
return crv;
}
@Override
public HttpFuture asyncQuery(AbstractView view, Query query) {
if(view.hasReduce() && !query.getArgs().containsKey("reduce")) {
query.setReduce(true);
}
if (query.willReduce()) {
return asyncQueryAndReduce(view, query);
} else if (query.willIncludeDocs()) {
return asyncQueryAndIncludeDocs(view, query);
} else {
return asyncQueryAndExcludeDocs(view, query);
}
}
/**
* Asynchronously queries a Couchbase view and returns the result.
* The result can be accessed row-wise via an iterator. This
* type of query will return the view result along with all of the documents
* for each row in the query.
*
* @param view the view to run the query against.
* @param query the type of query to run against the view.
* @return a Future containing the results of the query.
*/
private HttpFuture asyncQueryAndIncludeDocs(AbstractView view,
Query query) {
assert view != null : "Who passed me a null view";
assert query != null : "who passed me a null query";
String viewUri = view.getURI();
String queryToRun = query.toString();
assert viewUri != null : "view URI seems to be null";
assert queryToRun != null : "query seems to be null";
String uri = viewUri + queryToRun;
final CountDownLatch couchLatch = new CountDownLatch(1);
int timeout = ((CouchbaseConnectionFactory) connFactory).getViewTimeout();
final ViewFuture crv = new ViewFuture(couchLatch, timeout, view,
executorService);
final HttpRequest request =
new BasicHttpRequest("GET", uri, HttpVersion.HTTP_1_1);
final HttpOperation op = new DocsOperationImpl(request, view,
new ViewCallback() {
private ViewResponse vr = null;
@Override
public void receivedStatus(OperationStatus status) {
if (vr != null) {
Collection ids = new LinkedList();
Iterator itr = vr.iterator();
while (itr.hasNext()) {
ids.add(itr.next().getId());
}
crv.set(vr, asyncGetBulk(ids), status);
} else {
crv.set(null, null, status);
}
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
@Override
public void gotData(ViewResponse response) {
vr = response;
}
});
crv.setOperation(op);
addOp(op);
return crv;
}
/**
* Asynchronously queries a Couchbase view and returns the result.
* The result can be accessed row-wise via an iterator. This
* type of query will return the view result but will not
* get the documents associated with each row of the query.
*
* @param view the view to run the query against.
* @param query the type of query to run against the view.
* @return a Future containing the results of the query.
*/
private HttpFuture asyncQueryAndExcludeDocs(AbstractView view,
Query query) {
String uri = view.getURI() + query.toString();
final CountDownLatch couchLatch = new CountDownLatch(1);
int timeout = ((CouchbaseConnectionFactory) connFactory).getViewTimeout();
final HttpFuture crv =
new HttpFuture(couchLatch, timeout, executorService);
final HttpRequest request =
new BasicHttpRequest("GET", uri, HttpVersion.HTTP_1_1);
final HttpOperation op =
new NoDocsOperationImpl(request, view, new ViewCallback() {
private ViewResponse vr = null;
@Override
public void receivedStatus(OperationStatus status) {
crv.set(vr, status);
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
@Override
public void gotData(ViewResponse response) {
vr = response;
}
});
crv.setOperation(op);
addOp(op);
return crv;
}
/**
* Asynchronously queries a Couchbase view and returns the result.
* The result can be accessed row-wise via an iterator.
*
* @param view the view to run the query against.
* @param query the type of query to run against the view.
* @return a Future containing the results of the query.
*/
private HttpFuture asyncQueryAndReduce(final AbstractView view,
final Query query) {
if (!view.hasReduce()) {
throw new RuntimeException("This view doesn't contain a reduce function");
}
String uri = view.getURI() + query.toString();
final CountDownLatch couchLatch = new CountDownLatch(1);
int timeout = ((CouchbaseConnectionFactory) connFactory).getViewTimeout();
final HttpFuture crv =
new HttpFuture(couchLatch, timeout, executorService);
final HttpRequest request =
new BasicHttpRequest("GET", uri, HttpVersion.HTTP_1_1);
final HttpOperation op =
new ReducedOperationImpl(request, view, new ViewCallback() {
private ViewResponse vr = null;
@Override
public void receivedStatus(OperationStatus status) {
crv.set(vr, status);
}
@Override
public void complete() {
couchLatch.countDown();
crv.signalComplete();
}
@Override
public void gotData(ViewResponse response) {
vr = response;
}
});
crv.setOperation(op);
addOp(op);
return crv;
}
@Override
public ViewResponse query(AbstractView view, Query query) {
try {
return asyncQuery(view, query).get();
} catch (InterruptedException e) {
throw new RuntimeException("Interrupted while accessing the view", e);
} catch (ExecutionException e) {
if(e.getCause() instanceof CancellationException) {
throw (CancellationException) e.getCause();
} else {
throw new RuntimeException("Failed to access the view", e);
}
}
}
@Override
public Paginator paginatedQuery(View view, Query query, int docsPerPage) {
return new Paginator(this, view, query, docsPerPage);
}
/**
* Adds an operation to the queue where it waits to be sent to Couchbase.
*/
protected void addOp(final HttpOperation op) {
if(vconn != null) {
vconn.addOp(op);
}
}
@Override
public OperationFuture> asyncGetAndLock(final String key,
int exp, final Transcoder tc) {
final CountDownLatch latch = new CountDownLatch(1);
final OperationFuture> rv =
new OperationFuture>(key, latch, operationTimeout,
executorService);
Operation op = opFact.getl(key, exp, new GetlOperation.Callback() {
private CASValue val = null;
public void receivedStatus(OperationStatus status) {
if (!status.isSuccess()) {
val = new CASValue(-1, null);
}
rv.set(val, status);
}
public void gotData(String k, int flags, long cas, byte[] data) {
assert key.equals(k) : "Wrong key returned";
assert cas > 0 : "CAS was less than zero: " + cas;
val =
new CASValue(cas, tc.decode(new CachedData(flags, data, tc
.getMaxSize())));
}
public void complete() {
latch.countDown();
rv.signalComplete();
}
});
rv.setOperation(op);
mconn.enqueueOperation(key, op);
return rv;
}
@Override
public OperationFuture> asyncGetAndLock(final String key,
int exp) {
return asyncGetAndLock(key, exp, transcoder);
}
@Override
public Object getFromReplica(String key) {
return getFromReplica(key, transcoder);
}
@Override
public CASValue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy