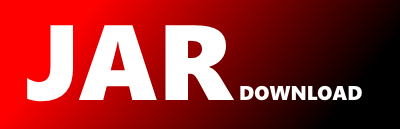
com.couchbase.client.java.query.DefaultN1qlQueryResult Maven / Gradle / Ivy
package com.couchbase.client.java.query;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.couchbase.client.java.document.json.JsonObject;
public class DefaultN1qlQueryResult implements N1qlQueryResult {
private final String status;
private final boolean finalSuccess;
private final boolean parseSuccess;
private final List allRows;
private final Object signature;
private final N1qlMetrics info;
private final List errors;
private final String requestId;
private final String clientContextId;
/**
* Create a default blocking representation of a query result.
*
* @param rows the list of rows.
* @param signature the signature for rows.
* @param info the metrics.
* @param errors the list of errors and warnings.
* @param finalStatus the definitive (but potentially delayed) status of the query.
* @param finalSuccess the definitive (but potentially delayed) success of the query.
* @param parseSuccess the intermediate result of the query
*/
public DefaultN1qlQueryResult(List rows, Object signature,
N1qlMetrics info, List errors,
String finalStatus, Boolean finalSuccess, boolean parseSuccess,
String requestId, String clientContextId) {
this.requestId = requestId;
this.clientContextId = clientContextId;
this.parseSuccess = parseSuccess;
this.finalSuccess = finalSuccess != null && finalSuccess;
this.status = finalStatus;
this.allRows = new ArrayList(rows.size());
for (AsyncN1qlQueryRow row : rows) {
this.allRows.add(new DefaultN1qlQueryRow(row));
}
this.signature = signature;
this.errors = errors;
this.info = info;
}
@Override
public List allRows() {
return this.allRows;
}
@Override
public Iterator rows() {
return this.allRows.iterator();
}
@Override
public Object signature() {
return this.signature;
}
@Override
public N1qlMetrics info() {
return this.info;
}
@Override
public boolean parseSuccess() {
return this.parseSuccess;
}
@Override
public List errors() {
return this.errors;
}
@Override
public boolean finalSuccess() {
return this.finalSuccess;
}
@Override
public String status() {
return status;
}
@Override
public Iterator iterator() {
return rows();
}
@Override
public String requestId() {
return this.requestId;
}
@Override
public String clientContextId() {
return this.clientContextId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy